70+ Spring Interview Questions
Published on May 1st, 2024
Top Questions
1. Question: Can you explain what the Spring Framework is?
Sample Answer: The Spring Framework is a widely used Java EE framework known for its lightweight and flexible architecture. It provides comprehensive infrastructure support for developing Java applications, offering solutions for dependency injection, aspect-oriented programming, and more.
2. Question: Why do developers often choose Spring Framework for Java EE development?
Sample Answer: Spring Framework is favored in Java EE development due to its robust features such as Dependency Injection (DI) and Aspect-Oriented Programming (AOP). It promotes loose coupling and modularity, making it easier to develop and maintain complex applications.
3. Question: How does Spring Framework encourage loose coupling in software development?
Sample Answer: Spring promotes loose coupling by implementing the Dependency Injection (DI) design pattern. With DI, components are decoupled from their dependencies, allowing for easier maintenance and scalability. Additionally, Spring's modular architecture enables developers to choose and integrate only the components they need, further enhancing modularity.
Question 4: What is Dependency Injection and how does Spring facilitate it?
Sample Answer: Dependency Injection (DI) is a design pattern used in software development to achieve loose coupling between components. In Spring, DI involves injecting the dependencies of a class from an external source, usually a Spring container. This allows for greater flexibility and easier management of dependencies, as the class itself doesn't need to create or manage its dependencies.
Question 5: Explain the benefits of Dependency Injection in Spring.
Sample Answer: Dependency Injection in Spring offers several benefits, including:
- Reduced Coupling: DI promotes loose coupling between components by removing the direct dependency between them.
- Increased Testability: With DI, it's easier to unit test components by injecting mock dependencies during testing.
- Flexibility: Components can be easily configured and replaced without modifying the code, promoting scalability and maintainability.
Question 6: Sample interview questions on Dependency Injection in Spring.
Sample Answer: Dependency Injection (DI) is a design pattern that allows an object to receive its dependencies from an external source rather than creating them itself. This promotes loose coupling, making the system more modular, maintainable, and testable.
Spring facilitates Dependency Injection primarily through the use of its IoC (Inversion of Control) container. The IoC container manages the lifecycle and configuration of application objects. There are two main types of dependency injection in Spring:
- Constructor Injection: Dependencies are provided through the constructor.
- Setter Injection: Dependencies are provided through setter methods.
Spring allows these dependencies to be injected via XML configuration, Java-based configuration using @Configuration and @Bean annotations, or annotation-based configuration using @Autowired.
Question 7: How does Spring resolve dependencies?
Sample Answer: Spring resolves dependencies through constructor injection, setter injection, or field injection.
Question 8: What are the different types of dependency injection supported by Spring?
Sample Answer: Spring supports constructor injection, setter injection, and field injection.
Question 9: Can you explain the difference between constructor injection and setter injection in Spring?
Sample Answer: Constructor injection involves passing dependencies to a class through its constructor, while setter injection involves setting dependencies through setter methods.
Question 10: How do you configure dependency injection in Spring?
Sample Answer: Dependency injection in Spring can be configured through XML-based configuration, Java-based configuration, or annotation-based configuration.
Question 11: Why is Spring Framework considered lightweight?
Sample Answer: Spring Framework is considered lightweight because it imposes minimal overhead on application development. It achieves this by providing only essential features and allowing developers to selectively use components based on project requirements. Additionally, Spring's modular architecture enables developers to include only the necessary modules, further reducing overhead.
Question 12: Discuss the advantages of minimal overhead in Spring development.
Sample Answer: The minimal overhead in Spring development offers several advantages, including:
- Improved Performance: With fewer layers and components, Spring applications tend to have better performance compared to heavier frameworks.
- Simplified Maintenance: The lightweight nature of Spring makes it easier to maintain and update applications, as there are fewer dependencies and complexities to manage.
- Faster Development: Developers can build applications more quickly and efficiently with Spring's lightweight architecture, leading to faster time-to-market.
Question 13: How does Spring's lightweight architecture contribute to application performance?
Sample Answer: Spring's minimal overhead reduces the complexity of applications, resulting in improved performance due to faster execution and reduced resource consumption.
Question 14: Can you explain how Spring allows developers to customize the framework based on project requirements?
Sample Answer: Spring's modular design enables developers to include only the necessary components and modules, reducing unnecessary overhead and optimizing application performance.
Question 15: How does Spring's lightweight nature benefit application scalability?
Sample Answer: By minimizing overhead and providing essential features, Spring simplifies the process of scaling applications, allowing them to handle increased loads more efficiently.
Question 16: How does Spring implement Dependency Injection and Inversion of Control?
Sample Answer: Spring implements Dependency Injection (DI) and Inversion of Control (IoC) through its IoC container, which manages the creation and injection of dependencies into classes. In DI, dependencies are injected into a class from an external source, while IoC dictates that control of object creation is transferred to the container.
Question 17: Explain the significance of DI and IoC in Spring.
Sample Answer: DI and IoC are fundamental concepts in Spring that promote loose coupling, modularity, and testability in applications. By allowing classes to depend on interfaces rather than concrete implementations, DI facilitates easier maintenance and scalability. IoC, on the other hand, simplifies object management by delegating control to the Spring container, resulting in more manageable and flexible code.
Question18: How does Dependency Injection promote modularity in Spring applications
Sample Answer: Dependency Injection decouples classes by removing hard-coded dependencies, making it easier to replace or modify components without affecting the overall system.
Question 19: Can you explain the difference between Dependency Injection and Inversion of Control?
Sample Answer: Dependency Injection focuses on injecting dependencies into a class, while Inversion of Control refers to delegating control of object creation and management to an external container, such as the Spring IoC container.
Question 20: How does Spring's IoC container manage object creation and dependency injection?
Sample Answer: The Spring IoC container is responsible for instantiating beans, resolving dependencies, and injecting them into classes based on configuration, either through XML, Java annotations, or Java configuration classes.
Question21: Overview of key Spring modules and their functionalities.
Sample Answer: Spring Framework comprises various modules, each serving a specific purpose in application development. Some key modules include Spring Core, Spring MVC, Spring JDBC, Spring Security, and Spring Boot. These modules provide functionalities such as dependency injection, web development, database access, security, and rapid application development.
Question 22: Importance of Spring community and resources.
Sample Answer: The Spring community plays a crucial role in supporting developers by providing resources, documentation, forums, and tutorials. The active community ensures that developers have access to up-to-date information, best practices, and solutions to common problems. Additionally, Spring's extensive documentation and official website offer comprehensive guides and references for developers at all skill levels.
Question 23: Can you name some of the key modules in the Spring Framework and their functionalities?
Sample Answer: Some key modules include Spring Core for dependency injection, Spring MVC for web development, Spring JDBC for database access, Spring Security for authentication and authorization, and Spring Boot for rapid application development.
Question 24: How does the Spring community support developers in learning and implementing the framework?
Sample Answer: The Spring community provides resources such as documentation, forums, tutorials, and sample projects to help developers learn and implement the framework effectively. Additionally, community members actively participate in discussions, share best practices, and contribute to open-source projects.
Question 24: What are some common challenges faced by developers when working with Spring, and how does the community address them?
Sample Answer: Common challenges include configuration errors, version compatibility issues, and debugging complex issues. The Spring community offers support through forums, mailing lists, and online communities, where developers can seek assistance, share experiences, and collaborate on solutions.
Question25: What are the major updates and enhancements in Spring 5?
Sample Answer: Spring 5 introduces several significant updates and enhancements, including:
- Full compatibility with Java 8 and higher versions, enabling the use of lambda expressions and other modern Java features.
- Introduction of Spring WebFlux for reactive programming, providing asynchronous and non-blocking capabilities for building web applications.
- Support for Java EE7 and Servlet 4.0 specifications, enhancing compatibility and interoperability with other Java technologies.
- Improved file operations using NIO 2 streams, resulting in better performance and efficiency for applications dealing with file handling.
- Streamlined logging with the introduction of spring-jcl, simplifying logging configuration and management in Spring applications.
Question 26: How does Spring 5 support Java 8 and higher versions?
Sample Answer: Spring 5 fully embraces Java 8 and higher versions by leveraging new language features such as lambda expressions, functional interfaces, and the Stream API. By adopting these features, Spring 5 enables developers to write more concise and expressive code, improving readability and maintainability. Additionally, Spring 5 provides compatibility with newer Java EE specifications, ensuring seamless integration with the latest Java technologies.
Question 27: Can you explain the significance of Spring WebFlux in Spring 5?
Sample Answer: Spring WebFlux introduces reactive programming capabilities to Spring, allowing developers to build asynchronous and non-blocking applications that can handle high concurrency and scalability requirements.
Question 28: How does Spring 5 enhance file operations compared to previous versions?
Sample Answer: Spring 5 utilizes NIO 2 streams for file operations, resulting in improved performance and efficiency, especially for applications that extensively handle file I/O operations.
Question 28: What are the benefits of using lambda expressions in Spring 5?
Sample Answer: Lambda expressions in Spring 5 enable developers to write more concise and expressive code, leading to improved readability and maintainability. They also facilitate the implementation of functional programming paradigms, enhancing code flexibility and reusability.
Question 29 : Explain the concept of Spring WebFlux and its significance.
Sample Answer: Spring WebFlux is a new module introduced in Spring 5 that brings reactive programming capabilities to the Spring Framework. It allows developers to build asynchronous and non-blocking web applications using the event-loop execution model. The significance of Spring WebFlux lies in its ability to handle high concurrency and scalability requirements, making it ideal for modern web development scenarios where responsiveness and performance are critical.
Question 30: Discuss the benefits of reactive programming in Spring.
Sample Answer: Reactive programming in Spring offers several benefits, including:
- Improved scalability: Reactive programming allows applications to handle a large number of concurrent requests efficiently, making it suitable for high-traffic scenarios.
- Enhanced responsiveness: By adopting non-blocking I/O operations, reactive applications can respond to requests more quickly, leading to a better user experience.
- Simplified concurrency: Reactive programming simplifies concurrency management by providing abstractions such as reactive streams and asynchronous processing, reducing the complexity of handling concurrent operations.
Question 31: How does reactive programming differ from traditional imperative programming?
Sample Answer: Reactive programming focuses on asynchronous and non-blocking operations, whereas traditional imperative programming relies on synchronous and blocking operations. Reactive programming emphasizes event-driven and declarative approaches to handle data streams efficiently.
Question 32: Can you explain the role of reactive streams in Spring WebFlux?
Sample Answer: Reactive streams provide a standard API for asynchronous stream processing in Java, enabling interoperability and compatibility among different reactive libraries and frameworks. In Spring WebFlux, reactive streams facilitate the processing of data streams asynchronously, allowing applications to handle concurrency and scalability requirements effectively.
Question 33: What are some common use cases for Spring WebFlux?
Sample Answer: Spring WebFlux is well-suited for scenarios that require high concurrency, real-time data processing, and event-driven architectures. Common use cases include real-time analytics, IoT applications, and streaming data processing.
Question 34: What are the different ways to configure Spring beans?
Sample Answer: Spring beans can be configured using XML configuration, Java-based configuration, or annotation-based configuration. XML configuration involves defining beans in an XML file using <bean> elements. Java-based configuration utilizes @Configuration classes and @Bean methods to define beans programmatically. Annotation-based configuration involves using annotations such as @Component, @Service, @Repository, and @Controller to mark classes as Spring beans.
Question 35: How does Spring manage the scopes and lifecycle of beans?
Sample Answer: Spring provides various bean scopes such as singleton, prototype, request, session, and global session to manage the lifecycle of beans. The singleton scope creates a single instance of the bean per container, while the prototype scope creates a new instance each time the bean is requested. Spring manages the bean lifecycle through initialization and destruction callbacks, allowing beans to perform custom initialization and cleanup tasks.
Question 36: Explain the difference between singleton and prototype bean scopes in Spring.
Sample Answer: Singleton scope creates a single instance of the bean per container, while prototype scope creates a new instance each time the bean is requested. Singleton beans are shared across the application context, while prototype beans are not.
Question 37: Compare and contrast Spring AOP and AspectJ.
Sample Answer: Spring AOP is a proxy-based AOP framework provided by the Spring Framework, whereas AspectJ is a more comprehensive and powerful AOP framework that operates at the bytecode level. Spring AOP offers simpler configuration and integration with Spring applications, while AspectJ provides more advanced features such as load-time weaving and compile-time weaving.
Question 38: What are aspects, advices, and pointcuts in Spring AOP?
Sample Answer:
- Aspects: Aspects encapsulate cross-cutting concerns and define reusable components that can be applied across multiple classes or modules.
- Advices: Advices are the actions taken by aspects at specific points, such as before, after, or around method execution.
- Pointcuts: Pointcuts define the join points in the application where advices should be applied. They specify the criteria for selecting the methods or join points to which an advice should be applied.
Question 39: How do you implement logging using Spring AOP?
Sample Answer: Logging can be implemented using an around advice, where the advice intercepts method invocations and performs logging before and after the method execution.
Question 40: What are the different types of advices supported by Spring AOP?
Sample Answer: Spring AOP supports different types of advices, including before advice, after returning advice, after throwing advice, and around advice, each serving different purposes in intercepting method invocations.
Question 41: Provide an overview of Spring MVC architecture.
Sample Answer: Spring MVC follows the Model-View-Controller architectural pattern, where the model represents the application data, the view represents the presentation layer, and the controller acts as an intermediary between the model and the view. The DispatcherServlet is the front controller that receives incoming requests, delegates processing to appropriate handlers, and dispatches responses to the client.
Question 42: What is the role of controllers in Spring MVC?
Sample Answer: Controllers in Spring MVC are responsible for handling incoming requests, processing business logic, and preparing model data for rendering views. Controllers typically contain handler methods annotated with @RequestMapping that map to specific URI patterns and HTTP methods.
Question 43: How do you handle form submissions in Spring MVC?
Sample Answer: Form submissions can be handled by defining a handler method in the controller annotated with @PostMapping or @RequestMapping for the corresponding URI pattern. The method should accept form data as parameters and process them accordingly.
Question 44: Explain the role of the @Controller annotation in Spring MVC.
Sample Answer: The @Controller annotation is used to mark a class as a controller in Spring MVC, indicating that it contains handler methods for processing incoming requests. Spring automatically detects and registers @Controller beans during application context initialization.
Question 45: What are some tips for effectively preparing for a Spring interview?
Sample Answer:
- Review core Spring concepts such as Dependency Injection, Aspect-Oriented Programming, and Spring MVC.
- Practice implementing Spring projects and solving real-world scenarios using Spring framework.
- Familiarize yourself with the latest features and updates in Spring, particularly in Spring 5.
- Be prepared to discuss your past experience with Spring projects and any challenges you encountered.
Question 46: How can one demonstrate proficiency in Spring during an interview?
Sample Answer:
- Showcase your understanding of Spring concepts by explaining them clearly and providing examples from your experience.
- Demonstrate your ability to solve problems using Spring framework, such as implementing dependency injection, configuring beans, and handling web requests in Spring MVC.
- Be prepared to discuss best practices and design patterns commonly used in Spring development.
Question 47: What are some common mistakes candidates make during Spring interviews?
Sample Answer:
- Lack of understanding of core Spring concepts such as Dependency Injection and Inversion of Control.
- Inability to explain their past Spring projects or provide clear examples of their work with Spring framework.
- Over-reliance on memorized answers without demonstrating problem-solving skills or critical thinking.
Question 48: How can candidates avoid common pitfalls during Spring interviews?
Sample Answer:
- Take the time to thoroughly understand core Spring concepts and their practical applications.
- Practice explaining your past Spring projects in detail, highlighting your role, challenges faced, and solutions implemented.
- Be prepared to demonstrate your problem-solving skills by discussing how you would approach different scenarios using Spring framework.
Question 49: What are some recommended resources for further study and practice in Spring development?
Sample Answer:
- Official Spring documentation and guides provide comprehensive explanations of Spring concepts, features, and best practices.
- Online tutorials and courses on platforms like Udemy, Coursera, and Pluralsight offer hands-on learning experiences and practical exercises.
- Books such as "Spring in Action" by Craig Walls and "Pro Spring 5" by Iuliana Cosmina provide in-depth coverage of Spring framework and its various modules.
- Participating in Spring community forums, discussion groups, and attending meetups or conferences can also enhance your understanding and networking opportunities.
Question 50: What are the different scopes of Spring beans?
Sample Answer: Spring supports several bean scopes:
- Singleton: Only one instance of the bean is created and shared throughout the container.
- Prototype: A new instance is created every time the bean is requested.
- Request: A new instance is created for each HTTP request (only for web applications).
- Session: A new instance is created for each HTTP session (only for web applications).
- Global Session: A new instance is created for each global HTTP session (only for web applications, typically used in Portlet contexts).
Question 51: How do you define a bean in Spring using Java configuration?
Sample Answer: In Java configuration, you can define a bean using the @Bean annotation inside a class annotated with @Configuration. Example:
java
@Configuration public class AppConfig { @Bean public MyService myService() { return new MyService(); } }
Category: Core Concepts of Spring Framework
Question 52: How does Spring promote loose coupling through DI and IoC?
Sample Answer: Spring promotes loose coupling by using Dependency Injection (DI) and Inversion of Control (IoC). Instead of hardcoding dependencies within the class, Spring allows dependencies to be injected externally. This decouples the class from the creation and management of its dependencies, making the system more flexible and easier to manage and test.
Question 53: What is Spring Boot and how does it simplify Spring application development?
Sample Answer: Spring Boot is a project within the Spring ecosystem that simplifies the development of Spring applications. It provides:
- Auto-configuration: Automatically configures Spring and third-party libraries based on the dependencies present in the project.
- Starter POMs: Set of convenient dependency descriptors that you can include in your application.
- Embedded Servers: Run web applications without the need for deploying to an external server.
This reduces boilerplate code and configuration, making it faster to get started with Spring development.
Question 54: What are some new features introduced in Spring 5?
Sample Answer: Spring 5 introduced several new features, including:
- Reactive Programming: Support for reactive programming with Spring WebFlux.
- Functional Programming: Support for functional bean registration using FunctionalWebApplication.
- Java 8 and 9 Support: Enhanced support for Java 8 features like lambda expressions, Streams API, and Java 9's java.util.Optional.
- HTTP/2: Support for HTTP/2 and reactive streams.
Question 55: How does Spring 5 support reactive programming?
Sample Answer: Spring 5 supports reactive programming through the introduction of Spring WebFlux, a reactive web framework. WebFlux uses Project Reactor and provides support for non-blocking, asynchronous request handling. This allows developers to build scalable and resilient applications that can handle a large number of concurrent users with lower resource utilization.
Question 56: How do you manage transactions in Spring?
Sample Answer: Transactions in Spring can be managed using the @Transactional annotation. This annotation can be applied to methods or classes to demarcate transactional boundaries. Spring manages the transaction lifecycle, including starting, committing, and rolling back transactions, based on the propagation and isolation settings specified.
Example:
java
Copy code
@Service public class MyService { @Transactional public void performTransaction() { // business logic here } }
Question 57: What are pointcuts in Spring AOP?
Sample Answer: Pointcuts are expressions that define where an advice should be applied in Spring AOP. They match join points (points in the execution of a program, such as method execution or exception handling). Pointcuts can be defined using annotations or XML, and they allow for fine-grained control over where cross-cutting concerns are applied.
Example using annotation:
java
Copy code
@Aspect public class MyAspect { @Pointcut("execution(* com.example.service.*.*(..))") public void serviceMethods() {} }
Question 58: What are some tips for preparing for a Spring interview?
Sample Answer: To prepare for a Spring interview:
- Understand Core Concepts: Have a solid grasp of DI, IoC, Spring Bean lifecycle, AOP, and transaction management.
- Hands-on Practice: Build sample applications and understand how different modules work together.
- Stay Updated: Keep up with the latest Spring releases and features.
- Study Common Patterns: Know the design patterns commonly used in Spring applications.
- Review Code Examples: Study code snippets and understand their functionality and design.
Question 59: What are some common pitfalls to avoid during a Spring interview?
Sample Answer: Common pitfalls to avoid during a Spring interview:
- Lack of Hands-on Experience: Theoretical knowledge without practical application can be a drawback.
- Ignoring Basic Concepts: Overlooking fundamental concepts like bean scopes, lifecycle, and DI.
- Not Understanding Configuration Options: Failing to understand different configuration methods (XML, Java, Annotations).
- Skipping New Features: Ignoring updates and new features in the latest Spring versions.
- Overlooking Performance Implications: Not considering the performance impact of certain Spring configurations and practices.
Question 60: What is the Spring Bean lifecycle?
Sample Answer: The Spring Bean lifecycle includes the following stages:
- Instantiation: The container creates an instance of the bean.
- Property Setting: Spring's dependency injection mechanism sets bean properties.
- Initialization: If the bean implements InitializingBean or defines an init-method, the afterPropertiesSet or init-method is called.
- Post Initialization: Any BeanPostProcessor beans are invoked before and after the initialization methods.
- Destruction: When the container is closed, if the bean implements DisposableBean or defines a destroy-method, the destroy or destroy-method is called.
Question 61: How does Spring handle dependency injection with annotations?
Sample Answer: Spring handles dependency injection with annotations using the @Autowired, @Inject, and @Resource annotations.
- @Autowired is a Spring-specific annotation that injects beans by type.
- @Inject is part of the Java CDI (Contexts and Dependency Injection) and works similarly to @Autowired.
- @Resource is part of JSR-250 and injects beans by name.
Example:
java
Copy code
@Service public class MyService { @Autowired private MyRepository myRepository; }
Question 62: What are some advantages of using Spring Boot?
Sample Answer: Advantages of using Spring Boot include:
- Auto-Configuration: Automatically configures Spring applications based on the dependencies present in the project.
- Starter POMs: Provide a quick way to include dependencies needed for a specific functionality.
- Embedded Servers: Allows running web applications without the need for an external web server.
- Production-ready Features: Includes metrics, health checks, and externalized configuration for production environments.
- Quick Setup: Reduces setup and development time, allowing developers to focus on business logic.
Question 63: How does Spring handle security?
Sample Answer: Spring Security is a powerful and highly customizable authentication and access-control framework. It provides comprehensive security services for Java applications, including:
- Authentication: Ensuring that a user is who they claim to be.
- Authorization: Deciding whether a user is allowed to perform an action.
- Protection Against Attacks: Defends against common exploits like CSRF, session fixation, and clickjacking.
- Integration: Works seamlessly with Spring applications and supports various authentication mechanisms (LDAP, OAuth2, JWT, etc.).
Question 64: How does Spring 5 integrate with Kotlin?
Sample Answer: Spring 5 offers first-class support for Kotlin, providing a more concise and expressive syntax. Key features include:
- Null Safety: Kotlin’s type system helps prevent null pointer exceptions.
- Extension Functions: Simplify code by allowing new functions to be added to existing classes.
- Coroutines: Enable easier asynchronous programming with less boilerplate code.
- DSL (Domain-Specific Language) Support: Create domain-specific languages to simplify configuration and usage patterns.
Question 65: What improvements in functional programming does Spring 5 provide?
Sample Answer: Spring 5 enhances functional programming with the following improvements:
- Functional Bean Registration: Allows defining beans using Java 8 lambda expressions and method references.
- Functional Endpoints: Provides a way to define web endpoints using the RouterFunction and HandlerFunction classes for a more functional approach to request handling.
- Integration with Java 8: Utilizes Java 8 features like lambda expressions, Optional, and Streams API.
Question 66: How does Spring handle transaction management with annotations?
Sample Answer: Spring handles transaction management with the @Transactional annotation, which can be applied to methods or classes to define transactional boundaries. Key attributes include:
- propagation: Defines how transactions relate to each other (e.g., REQUIRED, REQUIRES_NEW).
- isolation: Defines the isolation level for transactions (e.g., READ_COMMITTED, SERIALIZABLE).
- timeout: Specifies the timeout duration for a transaction.
- readOnly: Indicates whether the transaction is read-only.
Example:
java
Copy code
@Transactional(propagation = Propagation.REQUIRED, isolation = Isolation.READ_COMMITTED) public void performTransactionalOperation() { // business logic here }
Category: Advanced Topics in Spring Framework
Question 67: What are the key components of Spring AOP?
Sample Answer: Key components of Spring AOP include:
- Aspect: A module that contains the cross-cutting concerns.
- Join Point: A point during the execution of a program, such as a method execution.
- Advice: Action taken by an aspect at a particular join point.
- Pointcut: A predicate that matches join points.
- Introduction: Adding new methods or fields to existing classes.
- Weaving: The process of linking aspects with other application types or objects to create an advised object.
Question 68: What is the difference between @Controller and @RestController in Spring MVC?
Sample Answer: @Controller is used to mark a class as a Spring MVC controller, which can return view names and handle web requests. Methods in this controller typically return ModelAndView or view names.
@RestController is a specialized version of @Controller that combines @Controller and @ResponseBody. It simplifies the controller by returning objects directly as JSON or XML, making it ideal for RESTful web services.
Question 69: How do you handle file uploads in Spring MVC?
Sample Answer: File uploads in Spring MVC are handled using the MultipartFile interface. The configuration involves:
- Adding a multipart resolver bean to the configuration:
java
Copy code
@Bean public CommonsMultipartResolver multipartResolver() { CommonsMultipartResolver resolver = new CommonsMultipartResolver(); resolver.setMaxUploadSize(100000); return resolver; }
- Defining a controller method to handle the file upload:
java
Copy code
@PostMapping("/upload") public String handleFileUpload(@RequestParam("file") MultipartFile file) { if (!file.isEmpty()) { try { byte[] bytes = file.getBytes(); // Save the file to a location return "File uploaded successfully"; } catch (IOException e) { return "Failed to upload file"; } } else { return "File is empty"; } }
Question 70: How can you optimize the performance of a Spring application?
Sample Answer: To optimize the performance of a Spring application:
- Use Caching: Implement caching to reduce the load on the database and improve response times.
- Optimize Database Access: Use appropriate fetching strategies, connection pooling, and write efficient queries.
- Reduce Bean Initialization Time: Use lazy initialization for beans that are not needed at startup.
- Profile and Monitor: Use tools like Spring Boot Actuator, JMX, and APM solutions to monitor performance and identify bottlenecks.
- Optimize Configuration: Minimize XML configuration and prefer Java-based configuration for faster startup times.
Question 71: What are some common Spring interview questions to prepare for?
Sample Answer: Common Spring interview questions to prepare for include:
- Explain Dependency Injection and Inversion of Control.
- Describe the Spring Bean lifecycle.
- How does Spring handle transactions?
- What is Spring Boot and its benefits?
- How do you secure a Spring application?
- What is Spring AOP and how does it work?
- Explain the different types of bean scopes in Spring.
- What are some new features in Spring 5?
- How does Spring support reactive programming?
- Describe the differences between @Controller and @RestController.
Category: Best Practices and Tips for Spring Interviews
These additional questions and answers cover a broad range of topics within the Spring Framework, helping candidates prepare comprehensively for their interviews.
Read More:
- Different types of Interview Questions
- 10 Common Job Interview Questions And What Answers To Be Expected
- The Hiring Journey: 100+ Good Interview Questions to Ask In An Interview in 2024
- Here Are The Top Interview Questions You Need To Add To Your Interview Now!
- Informational Interview Questions
- 11+ Unique Interview Questions To Ask Employer During The Interview
- A Complete Guide on the Best Interview Questions to Ask Candidates
- Market Executive Interview Questions and Answers
- 30+ Second Interview Questions To Ask Candidates
- Employee Satisfaction Sample Questions
- How to Answer Interview Questions? [By Mastering the Employer's Perspective]
- Video Interview Questions: The Different Categories and Types
- Common Exit Interview Questions
- Customer Success Trainer Interview Questions
- Data Analyst Interview Questions: Learn to hire the perfect data analyst
- 50+ Content Marketing Expert Interview Questions and Answers
- Enterprise Customer Success Manager Interview Questions and Answers
- Online Advertising Expert Interview Questions & Answers
- Top 110+ Selenium Interview Questions and Answers
Authors
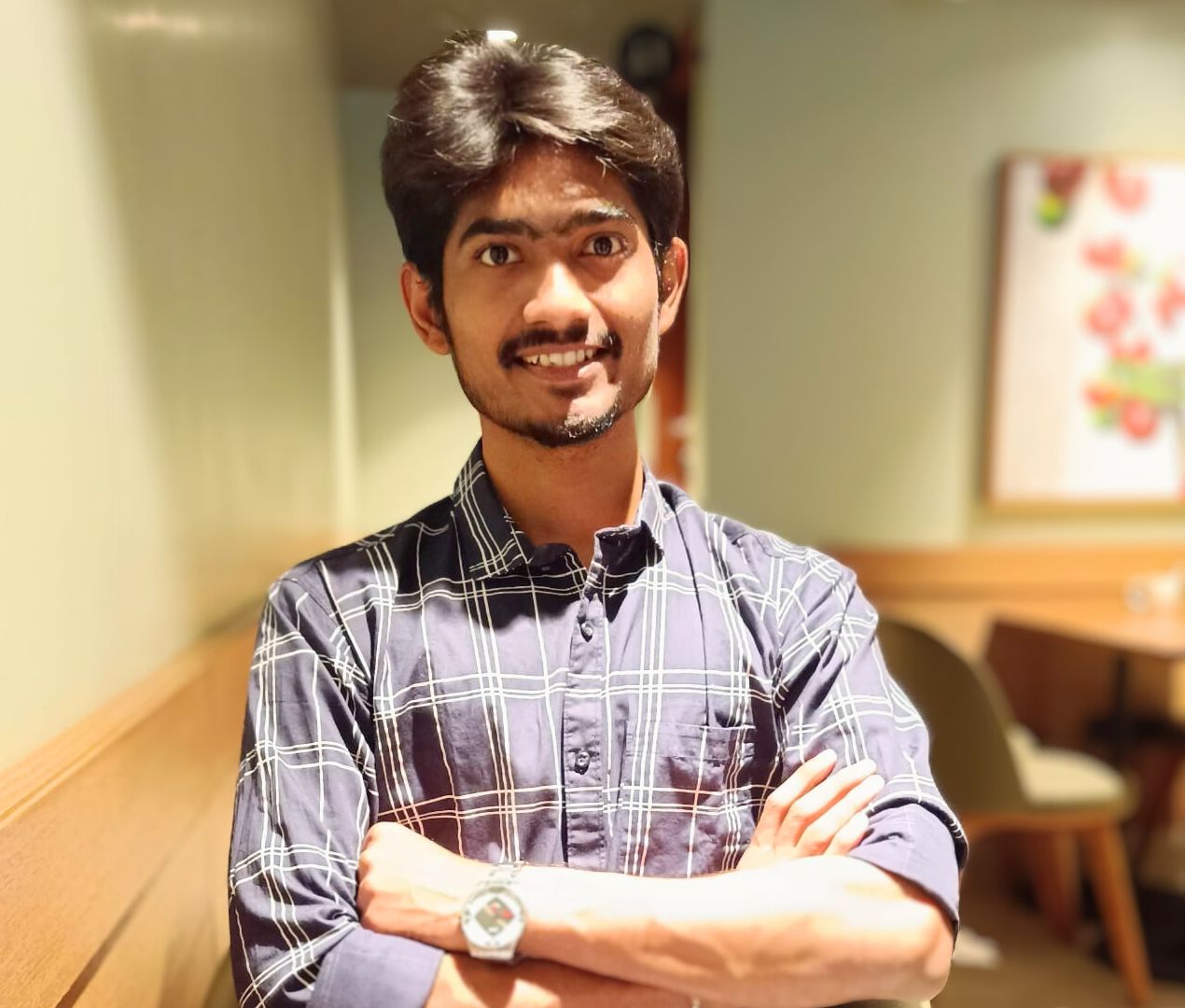
Yash Chaudhari
With a strong background as an SEO and Content Specialist, Yash excels in driving organic traffic, improving search engine rankings, and creating SEO-optimized content. He has a proven track record of implementing strategies that increase website traffic and conversions. Additionally, Yash is an automotive enthusiast and has a keen interest in astronomy.
Hire the best without stress
Ask us how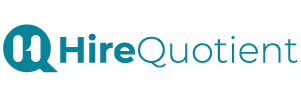
Never Miss The Updates
We cover all recruitment, talent analytics, L&D, DEI, pre-employment, candidate screening, and hiring tools. Join our force & subscribe now!
Stay On Top Of Everything In HR