110+ Spring Boot Interview Questions (Updated for 2024)
Published on May 3rd, 2024
Spring Boot is an open-source Java-based framework used to create stand-alone, production-grade Spring-based applications. It simplifies the setup and development of new Spring applications, making it a popular choice among developers. Whether you're a beginner or an experienced developer, preparing for a Spring Boot interview can be challenging. This guide provides 110+ questions and answers to help you master Spring Boot interview questions and stand out in your next job interview.
Table of Contents:
- Basic Questions
- Core Concepts
- Advanced Topics
- Spring Boot Features
- Practical Scenarios
- Integration and Deployment
- Security and Testing
- Best Practices and Performance
- Real-World Examples
- Additional Resources
1. Basic Questions
Q1: What is Spring Boot?
A1: Spring Boot is an open-source Java-based framework used to create stand-alone, production-grade Spring-based applications. It provides a simplified approach to developing applications with minimal configuration.
Q2: What are the main features of Spring Boot? A2: The main features of Spring Boot include:
- Auto-Configuration: Automatically configures your application based on the dependencies you have added.
- Spring Initializr: A web-based tool to create Spring Boot projects quickly.
- Actuator: Provides production-ready features like metrics, health checks, and monitoring.
- Embedded Servers: Built-in support for Tomcat, Jetty, and Undertow.
Q3: Explain the concept of auto-configuration. A3: Auto-configuration is a feature in Spring Boot that automatically configures the necessary components and settings for your application based on the dependencies present on the classpath. It reduces the need for manual configuration.
Q4: Describe the Spring Boot starter projects. A4: Spring Boot starter projects are a set of convenient dependency descriptors you can include in your application. They simplify the process of building an application by providing a one-stop-shop for all necessary dependencies. Examples include spring-boot-starter-web, spring-boot-starter-data-jpa, and spring-boot-starter-security.
Q5: What is the difference between Spring and Spring Boot? A5: Spring is a comprehensive framework for Java-based applications, providing a wide range of features. Spring Boot, on the other hand, is a project built on top of the Spring framework, designed to simplify the development process by providing default configurations and an opinionated approach to building applications.
Q6: Explain the purpose of the Spring Boot CLI. A6: The Spring Boot CLI (Command Line Interface) is a tool that allows you to quickly develop Spring applications using Groovy scripts. It helps in prototyping and simplifies the development process by eliminating boilerplate code.
Q7: What is the Spring Initializr? A7: The Spring Initializr is a web-based tool provided by Spring to generate Spring Boot project structures. It allows you to select the project dependencies, packaging type, and Java version, and then generates a ready-to-use Spring Boot project.
Q8: How does Spring Boot simplify dependency management? A8: Spring Boot simplifies dependency management by providing a set of starter POMs. These starter POMs are dependency descriptors that include the commonly used dependencies for a particular feature or functionality, reducing the need for manually specifying each dependency.
Q9: What are the advantages of using Spring Boot? A9: The advantages of using Spring Boot include:
- Rapid Development: Simplifies setup and reduces development time.
- Embedded Servers: Eliminates the need to deploy WAR files.
- Production-Ready Features: Includes monitoring, health checks, and metrics out-of-the-box.
- Microservices Support: Ideal for building and deploying microservices.
Q10: How to create a simple Spring Boot application? A10:
- Set Up the Project: Use Spring Initializr to generate a new project with the required dependencies.
- Add Dependencies: Include necessary dependencies in pom.xml or build.gradle.
- Create the Main Class: Annotate the main class with @SpringBootApplication.
- Run the Application: Use the Spring Boot Maven or Gradle plugin to run the application.
2. Core Concepts
Q11: Explain the @SpringBootApplication annotation. A11: The @SpringBootApplication annotation is a convenience annotation that combines @Configuration, @EnableAutoConfiguration, and @ComponentScan annotations. It marks the main class of a Spring Boot application and triggers auto-configuration, component scanning, and configuration properties.
Q12: Describe the role of application.properties and application.yml. A12: application.properties and application.yml are configuration files used in Spring Boot to externalize application settings. They allow you to define configuration properties in a centralized place, making it easier to manage and change configurations without modifying the application code.
Q13: What is Spring Boot Actuator? A13: Spring Boot Actuator provides production-ready features such as monitoring and managing applications. It includes endpoints for metrics, health checks, environment properties, and more, allowing you to monitor and manage your application in real-time.
Q14: How do you configure logging in Spring Boot? A14: Spring Boot uses the default logging framework (Logback). You can configure logging by creating a logback.xml or logback-spring.xml file and defining log levels, appenders, and log formats. Additionally, you can set logging properties in application.properties or application.yml.
Q15: Explain the @RestController annotation. A15: The @RestController annotation is a convenience annotation in Spring Boot that combines @Controller and @ResponseBody. It is used to create RESTful web services and automatically serializes the return objects to JSON or XML.
Q16: Describe the purpose of the CommandLineRunner interface. A16: The CommandLineRunner interface in Spring Boot is used to execute code after the Spring Boot application has started. It provides a run method that you can implement to execute any initialization logic or run specific tasks.
Q17: What is Spring Boot DevTools? A17: Spring Boot DevTools is a module that provides additional development-time features such as automatic restart, live reload, and configurations for improving the developer experience. It helps in faster development and testing by reducing the need for manual restarts.
Q18: How to configure a data source in Spring Boot? A18: To configure a data source in Spring Boot, you need to:
- Add Dependencies: Include the necessary database and JPA dependencies in pom.xml or build.gradle.
- Set Configuration Properties: Define the data source properties (URL, username, password, driver class) in application.properties or application.yml.
- Use @Entity and @Repository Annotations: Annotate your entity classes and repository interfaces.
Q19: Explain the difference between @Component, @Service, and @Repository. A19: @Component, @Service, and @Repository are stereotype annotations in Spring:
- @Component: Generic stereotype for any Spring-managed component.
- @Service: Specialization of @Component for service layer beans.
- @Repository: Specialization of @Component for DAO (Data Access Object) beans, also providing exception translation.
Q20: How do you handle exceptions in Spring Boot? A20: Exceptions in Spring Boot can be handled using:
- @ControllerAdvice: Global exception handling for controllers.
- @ExceptionHandler: Method-level exception handling within controllers.
- ResponseEntityExceptionHandler: Customize the response for different exception types.
3. Advanced Topics
Q21: Explain Spring Boot Profiles. A21: Spring Boot Profiles allow you to create and manage different configurations for various environments (e.g., development, testing, production). You can define properties for each profile in separate application-{profile}.properties or application-{profile}.yml files and activate a profile using the spring.profiles.active property.
Q22: How do you configure multiple data sources in Spring Boot? A22: To configure multiple data sources in Spring Boot, you need to:
- Define each data source in the application.properties file.
- Create separate configuration classes for each data source using @Configuration and @Primary annotations.
- Configure LocalContainerEntityManagerFactoryBean and PlatformTransactionManager beans for each data source.
Q23: Describe how to use Spring Boot with Kafka. A23: To use Spring Boot with Kafka:
- Add spring-kafka dependency to your project.
- Configure Kafka properties in application.properties.
- Create producer and consumer configurations using @Configuration classes.
- Implement producer and consumer classes to handle Kafka messages.
Q24: What is Spring Boot WebFlux? A24: Spring Boot WebFlux is a module for building reactive web applications in Spring Boot. It provides a reactive programming model based on the Reactor project and supports fully non-blocking communication, making it suitable for applications requiring high concurrency and low latency.
Q25: Explain the concept of reactive programming in Spring Boot. A25: Reactive programming in Spring Boot involves using the Spring WebFlux module to create non-blocking, event-driven applications. It leverages Project Reactor's Mono and Flux types to handle asynchronous data streams, enabling efficient use of system resources and improved application performance.
Q26: How to use Spring Boot with Docker? A26:
- Create a Dockerfile: Define the image with the base image, add application JAR, and specify entry point.
- Build the Docker Image: Use docker build command to create the image.
- Run the Docker Container: Use docker run command to start the container.
Q27: Describe how to implement caching in Spring Boot. A27: Implement caching in Spring Boot by:
- Adding spring-boot-starter-cache dependency.
- Enabling caching with @EnableCaching annotation.
- Configuring cache properties in application.properties.
- Using @Cacheable, @CachePut, and @CacheEvict annotations on methods to manage cache operations.
Q28: Explain the use of @ConfigurationProperties. A28: @ConfigurationProperties is used to bind external configuration properties to a Java object. You can define a class with fields corresponding to the properties, annotate it with @ConfigurationProperties, and load the properties from application.properties or application.yml.
Q29: How do you create a custom starter in Spring Boot? A29:
- Create a new project: Add dependencies and define the necessary beans and configurations.
- Define Auto-Configuration: Create an auto-configuration class annotated with @Configuration and @EnableAutoConfiguration.
- Package and Publish: Package the starter as a JAR and publish it to a Maven repository for reuse.
Q30: Describe Spring Boot’s support for JPA. A30: Spring Boot provides strong support for JPA through spring-boot-starter-data-jpa. It includes Hibernate as the default JPA implementation and simplifies database interactions with Spring Data repositories. Configuration is handled via application.properties, and entity classes are annotated with @Entity.
Q31: Explain the role of Spring Data REST. A31: Spring Data REST builds on Spring Data repositories and exposes them as RESTful web services. It automatically generates CRUD endpoints for repository entities and provides features like pagination, sorting, and HATEOAS links.
Q32: How to configure Spring Boot for asynchronous processing? A32: To configure asynchronous processing in Spring Boot:
- Enable async support with @EnableAsync.
- Define an Executor bean in a configuration class.
- Annotate methods to be executed asynchronously with @Async.
Q33: Describe the use of @Conditional annotations. A33: @Conditional annotations are used to conditionally enable or disable beans based on specific conditions. Examples include @ConditionalOnProperty, @ConditionalOnClass, and @ConditionalOnMissingBean. These annotations help create flexible and configurable applications.
Q34: Explain the process of configuring a custom banner. A34: To configure a custom banner in Spring Boot:
- Create a banner.txt file with your custom ASCII art or text.
- Place the file in the src/main/resources directory.
- Spring Boot will automatically display the custom banner on application startup.
Q35: How do you handle circular dependencies in Spring Boot? A35: Circular dependencies in Spring Boot can be handled by:
- Refactoring the code to avoid circular references.
- Using setter injection instead of constructor injection.
- Leveraging the @Lazy annotation to delay bean initialization until it is actually needed.
Q36: What is Spring Boot DevTools, and how can it enhance development? A21: Spring Boot DevTools provides features to improve the development experience, such as automatic restart, live reload, and configurations for efficient development. By enabling these features, developers can see the effects of their changes immediately without restarting the application manually.
Q37: Explain Spring Boot Profiles and their importance in configuration management. A22: Spring Boot Profiles allow developers to create different configurations for various environments like development, testing, and production. By using profile-specific properties files (e.g., application-dev.properties), you can tailor configurations to suit each environment, which simplifies deployment and ensures consistency across environments.
Q38: How do you configure multiple data sources in a Spring Boot application? A23: To configure multiple data sources:
- Define properties for each data source in application.properties.
- Create configuration classes annotated with @Configuration for each data source.
- Use @Primary to designate the default data source.
- Configure LocalContainerEntityManagerFactoryBean and PlatformTransactionManager for each data source.
Q39: What are Spring Boot starters, and how do they simplify dependency management? A24: Spring Boot starters are a set of convenient dependency descriptors that you can include in your application. They provide a curated set of dependencies for a particular functionality, such as spring-boot-starter-web for web applications or spring-boot-starter-data-jpa for JPA-based data access, simplifying the setup and maintenance of dependencies.
Q40: Describe how to use Spring Boot with Docker. A25:
- Create a Dockerfile in the root of your Spring Boot project.
- Use a base image like openjdk:11-jre-slim.
- Copy the JAR file into the container.
- Set the entry point to run the JAR file.
- Build the Docker image using docker build.
- Run the container using docker run.
Q41: How does Spring Boot support reactive programming with WebFlux? A26: Spring Boot WebFlux provides support for reactive programming, allowing for non-blocking, event-driven applications. It uses the Reactor library, and core components include Mono and Flux, which represent single or multiple asynchronous sequences of data. This model is beneficial for applications requiring high concurrency and low latency.
Q42: Explain the concept and benefits of Spring Boot Actuator. A27: Spring Boot Actuator provides production-ready features such as monitoring and management. It includes various endpoints that expose information about the application’s health, metrics, environment, and more. This helps in maintaining and monitoring the application effectively.
Q43: What are the advantages of using Spring Boot with GraphQL? A28: Using Spring Boot with GraphQL allows developers to build efficient and flexible APIs. GraphQL provides a single endpoint for querying and mutating data, enabling clients to specify the exact data they need. This reduces over-fetching and under-fetching of data, improves performance, and enhances the client-server interaction.
Q44: How do you handle exception management in Spring Boot? A29: Exception management in Spring Boot can be handled using:
- @ControllerAdvice for global exception handling.
- @ExceptionHandler for specific exception types within controllers.
- Customizing error responses with ResponseEntityExceptionHandler.
- Returning meaningful error messages and HTTP status codes.
Q45: Describe the use and benefits of Spring Boot’s @ConfigurationProperties. A30: @ConfigurationProperties allows for type-safe configuration binding, mapping properties defined in application.properties or application.yml to Java objects. This simplifies managing complex configurations, promotes cleaner code, and makes configuration more manageable and readable.
Q46: How do you configure and use Spring Boot’s embedded servers? A31: Spring Boot supports embedded servers like Tomcat, Jetty, and Undertow. By including the appropriate starter dependency, Spring Boot automatically configures the embedded server. This allows for easy setup and eliminates the need for external server configuration, simplifying deployment and testing.
Q47: What is Spring Cloud, and how does it enhance Spring Boot applications? A32: Spring Cloud provides tools for building distributed systems and microservices. It enhances Spring Boot applications by offering features such as service discovery (Eureka), configuration management (Config Server), circuit breakers (Hystrix), intelligent routing (Zuul), and more. These tools help in creating scalable, resilient, and manageable microservices architectures.
Q33: How do you implement a custom starter in Spring Boot? A33:
- Create a new project with the necessary dependencies.
- Define auto-configuration classes annotated with @Configuration.
- Use META-INF/spring.factories to register auto-configuration classes.
- Package and publish the starter to a Maven repository for reuse.
Q34: Explain the purpose and usage of Spring Boot’s @SpringBootApplication annotation. A34: @SpringBootApplication is a convenience annotation that combines @Configuration, @EnableAutoConfiguration, and @ComponentScan. It marks the main class of a Spring Boot application and triggers auto-configuration and component scanning, simplifying the application setup process.
Q35: How do you use Spring Boot with Kubernetes for microservices deployment? A35:
- Containerize your Spring Boot application using Docker.
- Deploy the Docker image to a Kubernetes cluster.
- Define Kubernetes deployment and service YAML files.
- Use kubectl to apply the deployment and service configurations.
- Manage and scale the microservices using Kubernetes features.
4. Spring Boot Features
Q36: What is Spring Boot Actuator? How is it used? A36: Spring Boot Actuator provides production-ready features such as monitoring and managing applications. It includes various endpoints like /health, /info, and /metrics, which can be accessed to get information about the application's state. You can customize Actuator endpoints in application.properties.
Q37: Describe the Spring Boot DevTools module. A37: Spring Boot DevTools provides features that enhance the development experience, such as automatic restart, live reload, and configurations for efficient development. It helps developers by speeding up the development cycle and reducing the need for manual restarts.
Q38: Explain Spring Boot’s support for embedded servers. A38: Spring Boot supports embedded servers like Tomcat, Jetty, and Undertow. This allows developers to package their applications as standalone JAR files that include the web server, making it easy to deploy and run the application without needing an external server.
Q39: How do you enable SSL in a Spring Boot application? A39:
- Obtain SSL Certificate: Get a certificate from a Certificate Authority (CA).
- Configure SSL Properties: Set up SSL properties in application.properties, including keystore path, password, and key alias.
- Enable HTTPS: Ensure the server is configured to use HTTPS by setting the appropriate properties.
Q40: What are Spring Boot starters? Name a few commonly used starters. A40: Spring Boot starters are a set of convenient dependency descriptors that you can include in your application. They simplify dependency management by providing a curated list of dependencies for a specific functionality. Commonly used starters include spring-boot-starter-web, spring-boot-starter-data-jpa, spring-boot-starter-security, and spring-boot-starter-test.
Q41: Describe the Spring Boot testing features. A41: Spring Boot provides extensive testing support, including:
- @SpringBootTest: Loads the full application context for integration tests.
- @WebMvcTest: Loads only the web layer for testing controllers.
- @DataJpaTest: Configures an in-memory database for testing JPA repositories.
- Embedded servers for testing web applications without needing a real server.
Q42: How do you use Spring Boot with Thymeleaf? A42: To use Spring Boot with Thymeleaf:
- Add spring-boot-starter-thymeleaf dependency.
- Create HTML templates in the src/main/resources/templates directory.
- Use Thymeleaf-specific tags and expressions in your templates to render dynamic content.
Q43: Explain the Spring Boot configuration properties. A43: Spring Boot configuration properties allow you to externalize application settings into application.properties or application.yml files. These properties can be injected into beans using @Value or @ConfigurationProperties annotations, providing a flexible way to configure the application.
Q44: How to set up a Spring Boot project with Gradle? A44:
- Initialize the Project: Use Spring Initializr to generate a Gradle-based project.
- Configure Build File: Define dependencies and plugins in build.gradle.
- Run the Project: Use ./gradlew bootRun to start the application.
Q45: Describe Spring Boot’s integration with Spring Cloud. A45: Spring Boot integrates seamlessly with Spring Cloud to build cloud-native applications. Spring Cloud provides tools for service discovery, configuration management, circuit breakers, intelligent routing, and more, enabling the development of resilient and scalable microservices.
5. Practical Scenarios
Q46: How to handle file uploads in Spring Boot? A46:
- Add Dependencies: Include spring-boot-starter-web.
- Configure Multipart Properties: Set properties in application.properties.
- Create Controller: Use @PostMapping with @RequestParam to handle file uploads.
- Save File: Implement logic to save the uploaded file to the server.
Q47: Describe the process of sending emails in a Spring Boot application. A47:
- Add Dependencies: Include spring-boot-starter-mail.
- Configure Mail Properties: Define mail server settings in application.properties.
- Create Mail Service: Use JavaMailSender to send emails.
- Send Email: Implement methods to construct and send emails.
Q48: Explain how to schedule tasks in Spring Boot. A48:
- Enable Scheduling: Annotate a configuration class with @EnableScheduling.
- Create Scheduled Tasks: Use @Scheduled on methods to define task intervals.
- Configure Task Properties: Set scheduling properties in application.properties.
Q49: How do you implement pagination in Spring Boot? A49:
- Add Dependencies: Include spring-boot-starter-data-jpa.
- Define Pageable Method: Create repository methods that accept Pageable parameter.
- Create Controller: Implement endpoints to handle pagination requests.
Q50: Describe the process of integrating Spring Boot with a NoSQL database. A50:
- Add Dependencies: Include NoSQL database dependencies (e.g., MongoDB).
- Configure Data Source: Define NoSQL database properties in application.properties.
- Create Entities and Repositories: Annotate entity classes and define repository interfaces.
- Use Repositories: Implement service and controller layers to interact with the database.
Q51: How to implement security in a Spring Boot application? A51:
- Add Dependencies: Include spring-boot-starter-security.
- Configure Security Settings: Define security properties in application.properties.
- Create Security Configuration: Extend WebSecurityConfigurerAdapter and configure authentication and authorization.
Q52: Explain the steps to create a RESTful API with Spring Boot. A52:
- Add Dependencies: Include spring-boot-starter-web.
- Define Entity Classes: Annotate with @Entity.
- Create Repositories: Extend JpaRepository.
- Create Controllers: Use @RestController and define API endpoints with @GetMapping, @PostMapping, etc.
Q53: How do you use Spring Boot with WebSockets? A53:
- Add Dependencies: Include spring-boot-starter-websocket.
- Enable WebSocket: Annotate a configuration class with @EnableWebSocketMessageBroker.
- Create WebSocket Configuration: Implement WebSocketConfigurer and register handlers.
Q54: Describe the process of monitoring a Spring Boot application. A54: Use Spring Boot Actuator to monitor application metrics and health. Configure Actuator endpoints in application.properties and access metrics through predefined endpoints like /actuator/metrics.
Q55: How to create a microservice with Spring Boot? A55:
- Define Service: Use Spring Initializr to create a new Spring Boot project.
- Add Dependencies: Include necessary dependencies for RESTful services.
- Implement Service Logic: Create controllers, services, and repositories.
- Deploy: Use Docker or a cloud platform to deploy the microservice.
6. Integration and Deployment
Q56: How do you deploy a Spring Boot application to AWS? A56:
- Prepare Application: Package as a JAR or WAR.
- Set Up AWS: Create an EC2 instance or use Elastic Beanstalk.
- Deploy: Transfer the application package and run it on the server.
Q57: Explain the process of deploying a Spring Boot application to Docker. A57:
- Create Dockerfile: Define base image, copy JAR file, and set entry point.
- Build Docker Image: Use docker build command.
- Run Docker Container: Use docker run command.
Q58: Describe how to integrate Spring Boot with Jenkins for CI/CD. A58:
- Set Up Jenkins: Install and configure Jenkins.
- Create Pipeline: Define a Jenkins pipeline to build and deploy the application.
- Integrate SCM: Connect to source code repository (e.g., Git).
- Automate Deployment: Use Jenkins to trigger builds and deployments on code changes.
Q59: What are the steps to deploy a Spring Boot application to Kubernetes? A59:
- Containerize Application: Create Docker image.
- Set Up Kubernetes Cluster: Use a managed service like GKE, EKS, or AKS.
- Deploy to Kubernetes: Create deployment and service YAML files, and apply them using kubectl.
Q60: How do you use Spring Boot with Kafka? A60: Add spring-kafka dependency, configure Kafka properties in application.properties, and create producer and consumer classes to handle Kafka messages.
Q61: Describe the process of using Spring Boot with RabbitMQ. A61: Add spring-boot-starter-amqp dependency, configure RabbitMQ properties in application.properties, and create message listener and sender classes.
Q62: Explain how to integrate Spring Boot with Elasticsearch. A62: Add spring-data-elasticsearch dependency, configure Elasticsearch properties in application.properties, and create repository interfaces extending ElasticsearchRepository.
Q63: How do you configure a Spring Boot application for Azure? A63: Use Azure Spring Cloud or deploy to an Azure App Service. Configure application settings and deploy the Spring Boot application using Azure CLI or the Azure portal.
Q64: Describe the process of deploying a Spring Boot application to Google Cloud. A64: Use Google Cloud App Engine or Kubernetes Engine. Configure application properties and deploy using Google Cloud SDK or the Google Cloud Console.
Q65: How to deploy a Spring Boot application as a WAR file? A65:
- Modify POM File: Set packaging to war and add Spring Boot starter Tomcat.
- Extend SpringBootServletInitializer: Create a class extending SpringBootServletInitializer.
- Deploy WAR File: Deploy the WAR file to an external server like Tomcat.
7. Security and Testing
Q66: Explain Spring Boot Security and its features. A66: Spring Boot Security provides authentication and authorization features for securing applications. It supports various authentication mechanisms, CSRF protection, and method-level security annotations.
Q67: How do you implement OAuth2 in Spring Boot? A67:
- Add Dependencies: Include spring-boot-starter-oauth2-client and spring-boot-starter-security.
- Configure OAuth2 Properties: Set OAuth2 client details in application.properties.
- Create Security Configuration: Configure OAuth2 login and resource server settings.
Q68: Describe the process of configuring JWT authentication. A68: Add JWT dependencies, create filters for JWT validation, configure security settings, and generate tokens upon successful authentication.
Q69: How to secure a Spring Boot application with Spring Security? A69:
- Add Dependencies: Include spring-boot-starter-security.
- Create Security Configuration: Extend WebSecurityConfigurerAdapter and define security rules.
- Configure Authentication: Implement user details service and configure authentication providers.
Q70: Explain how to perform unit testing in Spring Boot. A70:
- Add Dependencies: Include spring-boot-starter-test.
- Create Test Classes: Use @SpringBootTest, @MockBean, and other testing annotations.
- Run Tests: Use JUnit or another testing framework to execute tests.
Q71: Describe the process of integration testing in Spring Boot. A71:
- Add Dependencies: Include spring-boot-starter-test.
- Create Test Classes: Use @SpringBootTest to load the application context.
- Write Test Cases: Implement tests to verify interactions between components.
Q72: How do you test RESTful services in Spring Boot? A72:
- Add Dependencies: Include spring-boot-starter-test and spring-boot-starter-web.
- Create Test Classes: Use @WebMvcTest for controller tests.
- Use MockMvc: Mock HTTP requests and verify responses.
Q73: Explain how to use MockMvc in Spring Boot tests. A73: MockMvc is used to simulate HTTP requests in Spring Boot tests. Configure MockMvc in your test classes and use it to perform requests and verify responses without starting a full server.
Q74: Describe the process of testing Spring Boot applications with Testcontainers. A74: Testcontainers provides Docker-based test environments. Add Testcontainers dependencies, configure containers in test classes, and use containers for integration tests with real dependencies.
Q75: How to test Spring Boot applications with JUnit 5? A75: Add JUnit 5 dependencies, create test classes annotated with @SpringBootTest, and write test methods using JUnit 5 annotations like @Test, @BeforeEach, and @AfterEach. Utilize assertions to verify the expected outcomes of your tests.
Q76: Explain how to use Mockito for testing Spring Boot applications. A76: Mockito is used for mocking dependencies in tests. Add Mockito dependencies, annotate your test class with @ExtendWith(MockitoExtension.class), and use @Mock to create mock objects. Use when and thenReturn methods to define behavior for mocked methods.
Q77: Describe the process of performance testing in Spring Boot. A77: Performance testing in Spring Boot involves using tools like JMeter or Gatling to simulate load and measure response times. Profile the application using Actuator endpoints and analyze metrics to identify bottlenecks.
Q78: How do you use Spring Boot with Selenium for end-to-end testing? A78: Selenium is used for automating browser interactions. Add Selenium dependencies, configure WebDriver, and write tests to simulate user interactions with the web application. Use assertions to verify the correctness of the application's behavior.
Q79: Explain how to implement role-based access control in Spring Boot. A79: Implement role-based access control by defining roles and permissions in the security configuration. Use @PreAuthorize and @Secured annotations to secure methods based on roles. Configure role hierarchies if needed.
Q80: How to handle CSRF protection in Spring Boot? A80: CSRF protection is enabled by default in Spring Security. It ensures that state-changing requests are accompanied by a valid CSRF token. Configure CSRF settings in the security configuration and include the CSRF token in forms or AJAX requests.
8. Best Practices and Performance
Q81: What are the best practices for building a Spring Boot application? A81: Best practices include:
- Using Spring Boot starters for dependency management.
- Externalizing configuration with application.properties or application.yml.
- Implementing logging and monitoring with Actuator.
- Writing unit and integration tests.
- Following coding standards and clean code principles.
Q82: How to improve the performance of a Spring Boot application? A82: Improve performance by:
- Caching frequently accessed data.
- Optimizing database queries.
- Using asynchronous processing.
- Profiling the application to identify bottlenecks.
- Tuning JVM settings and garbage collection.
Q83: Describe the process of optimizing Spring Boot application startup time. A83: Optimize startup time by:
- Reducing the number of beans loaded.
- Using lazy initialization.
- Disabling unused auto-configurations.
- Analyzing startup logs to identify slow components.
Q84: How do you handle memory management in Spring Boot? A84: Handle memory management by:
- Monitoring memory usage with Actuator endpoints.
- Configuring heap size and garbage collection settings.
- Identifying memory leaks using profiling tools.
- Optimizing code to reduce memory footprint.
Q85: Explain the best practices for logging in Spring Boot. A85: Best practices for logging include:
- Using a consistent logging format.
- Logging at appropriate levels (INFO, WARN, ERROR).
- Externalizing log configurations.
- Using structured logging for better analysis.
- Avoiding logging sensitive information.
Q86: Describe how to monitor a Spring Boot application in production. A86: Monitor a Spring Boot application using:
- Spring Boot Actuator for health checks and metrics.
- External monitoring tools like Prometheus and Grafana.
- Setting up alerts for critical metrics.
- Logging important events and errors.
Q87: How do you manage configuration properties in Spring Boot? A87: Manage configuration properties by:
- Using application.properties or application.yml.
- Externalizing sensitive information with environment variables.
- Using @ConfigurationProperties for type-safe configuration.
- Creating separate profiles for different environments.
Q88: Explain the process of using Spring Boot with a service registry. A88: Use Spring Boot with a service registry like Eureka by:
- Adding spring-cloud-starter-netflix-eureka-client dependency.
- Configuring Eureka server URL in application.properties.
- Annotating the main application class with @EnableEurekaClient.
- Registering services with Eureka and discovering them using Feign or RestTemplate.
Q89: What are the best practices for securing a Spring Boot application? A89: Best practices for securing a Spring Boot application include:
- Implementing strong authentication and authorization.
- Using HTTPS for secure communication.
- Applying CSRF protection.
- Validating and sanitizing user inputs.
- Regularly updating dependencies to fix vulnerabilities.
Q90: How to implement exception handling best practices in Spring Boot? A90: Implement exception handling best practices by:
- Using @ControllerAdvice and @ExceptionHandler for global exception handling.
- Returning meaningful error messages with appropriate HTTP status codes.
- Logging exceptions for troubleshooting.
- Creating custom exception classes for specific error scenarios.
9. Real-World Examples
Q91: How to create a CRUD application with Spring Boot? A91:
- Set Up Project: Use Spring Initializr to create a new project with Web and JPA dependencies.
- Define Entity Classes: Annotate classes with @Entity and @Table.
- Create Repositories: Extend JpaRepository.
- Implement Controllers: Use @RestController to define CRUD endpoints.
- Run Application: Start the application and test CRUD operations.
Q92: Describe the process of building a RESTful API with Spring Boot. A92:
- Set Up Project: Use Spring Initializr to create a new project with Web dependency.
- Define Entity Classes: Annotate with @Entity.
- Create Repositories: Extend JpaRepository.
- Create Controllers: Use @RestController and define API endpoints.
- Run Application: Start the application and test the API using tools like Postman.
Q93: Explain how to integrate Spring Boot with Angular. A93:
- Set Up Spring Boot Project: Create a new Spring Boot project with Web dependency.
- Set Up Angular Project: Create a new Angular project.
- Develop RESTful Services: Implement REST endpoints in Spring Boot.
- Consume RESTful Services: Use Angular's HttpClient to call REST endpoints.
- Deploy Application: Deploy both applications to a web server.
Q94: How to build a microservice with Spring Boot and Spring Cloud? A94:
- Set Up Project: Create a new Spring Boot project with necessary dependencies.
- Implement Service Logic: Create controllers, services, and repositories.
- Configure Service Registry: Use Eureka for service discovery.
- Implement API Gateway: Use Spring Cloud Gateway for routing.
- Deploy Services: Deploy microservices independently.
Q95: Describe the process of creating a Spring Boot application with MySQL. A95:
- Set Up Project: Use Spring Initializr to create a new project with Web and JPA dependencies.
- Configure Data Source: Define MySQL properties in application.properties.
- Define Entity Classes: Annotate with @Entity.
- Create Repositories: Extend JpaRepository.
- Run Application: Start the application and test database operations.
Q96: How do you use Spring Boot with Redis? A96:
- Add Dependencies: Include spring-boot-starter-data-redis.
- Configure Redis: Define Redis properties in application.properties.
- Create Redis Repositories: Use @Repository and RedisTemplate.
- Implement Caching: Use @Cacheable, @CachePut, and @CacheEvict annotations.
Q97: Explain how to implement a messaging system with Spring Boot and RabbitMQ. A97:
- Add Dependencies: Include spring-boot-starter-amqp.
- Configure RabbitMQ: Define RabbitMQ properties in application.properties.
- Create Message Listener: Use @RabbitListener to handle messages.
- Send Messages: Use RabbitTemplate to send messages to RabbitMQ.
Q98: Describe the process of integrating Spring Boot with Apache Kafka. A98:
- Add Dependencies: Include spring-kafka.
- Configure Kafka: Define Kafka properties in application.properties.
- Create Producer and Consumer: Implement classes to produce and consume messages.
- Test Integration: Run the application and verify Kafka message handling.
Q99: How to build a secure login application with Spring Boot? A99:
- Set Up Project: Use Spring Initializr to create a new project with Web and Security dependencies.
- Implement User Entity: Define user details and roles.
- Create Security Configuration: Extend WebSecurityConfigurerAdapter and configure authentication and authorization.
- Create Login Controller: Implement endpoints for login and user management.
- Run Application: Start the application and test the login functionality.
Q100: Explain how to create a Spring Boot application with MongoDB. A100:
- Add Dependencies: Include spring-boot-starter-data-mongodb.
- Configure MongoDB: Define MongoDB properties in application.properties.
- Define Entity Classes: Annotate classes with @Document.
- Create Repositories: Extend MongoRepository.
- Implement Controllers: Use @RestController to define CRUD endpoints.
- Run Application: Start the application and interact with MongoDB.
Q101: Describe the process of building a Spring Boot application with GraphQL. A101:
- Add Dependencies: Include spring-boot-starter-graphql.
- Define Schema: Create a schema file defining the GraphQL queries and mutations.
- Create Resolvers: Implement resolver classes to handle data fetching.
- Configure GraphQL: Set up GraphQL properties in application.properties.
- Run Application: Start the application and test GraphQL endpoints.
Q102: How to integrate Spring Boot with Vue.js? A102:
- Set Up Spring Boot Project: Create a new Spring Boot project with Web dependency.
- Set Up Vue.js Project: Create a new Vue.js project.
- Develop RESTful Services: Implement REST endpoints in Spring Boot.
- Consume RESTful Services: Use Vue.js to call REST endpoints.
- Build and Deploy: Combine and deploy both applications on a web server.
Q103: Explain how to create a multi-module Spring Boot project. A103:
- Set Up Parent Project: Create a parent project using Spring Initializr.
- Create Modules: Add multiple modules for different components (e.g., web, service, repository).
- Configure Parent POM: Define parent POM to manage dependencies and plugins.
- Develop Modules: Implement functionality within each module.
- Build Project: Use Maven to build the entire project.
Q104: Describe the process of setting up a Spring Boot project with Maven. A104:
- Initialize Project: Use Spring Initializr to create a new Maven-based project.
- Configure POM File: Define dependencies and plugins in pom.xml.
- Develop Application: Implement entities, repositories, services, and controllers.
- Build Project: Use mvn clean install to build the project.
- Run Application: Use mvn spring-boot:run to start the application.
Q105: How to implement a file download feature in Spring Boot? A105:
- Create Controller: Define a REST controller with an endpoint for file download.
- Write Download Logic: Implement logic to read and return the file as a response.
- Set Response Headers: Set appropriate HTTP headers (e.g., Content-Disposition) for the file.
- Test Endpoint: Access the endpoint to download the file.
10. Additional Resources
Q106: What are some recommended books and tutorials on Spring Boot? A106: Recommended resources include:
- "Spring Boot in Action" by Craig Walls
- "Spring Boot: Up and Running" by Mark Heckler
- Online tutorials from Baeldung, Spring.io, and Pluralsight
Q107: Where can I find the official Spring Boot documentation? A107: The official Spring Boot documentation is available at Spring.io.
Q108: What are some useful community forums and discussion groups for Spring Boot? A108: Useful forums include Stack Overflow, the Spring Boot Gitter channel, and the Spring Community forum.
Q109: Are there any online courses and certifications for Spring Boot? A109: Yes, platforms like Udemy, Coursera, and Spring Academy offer online courses and certifications for Spring Boot.
Q110: What tools and libraries are helpful for Spring Boot development? A110: Helpful tools and libraries include Spring Tool Suite (STS), IntelliJ IDEA, Lombok, and MapStruct.
Mastering Spring Boot interview questions is essential for developers aiming to excel in their careers. This guide, with its extensive collection of questions and answers, ensures comprehensive preparation. Keep practicing and exploring beyond these questions to stay ahead in your interviews.
As technology continues to advance, tools like AI Answer Generators are revolutionizing how candidates prepare for interviews, including technical ones like those focusing on Spring Boot. AI Answer Generators use artificial intelligence to provide precise, real-time responses to common interview questions, making them a valuable resource for brushing up on key concepts.
Benefits of Using AI Answer Generators:
- Instant Responses: AI-powered tools can generate quick and accurate answers to a wide array of interview questions, helping you prepare faster and more efficiently.
- Personalized Learning: Based on the input you provide, AI Answer Generators can tailor responses to your specific needs, focusing on areas where you need more preparation.
- Comprehensive Coverage: Whether you're studying basic questions or delving into advanced Spring Boot concepts, AI Answer Generators offer a wide range of information across various topics.
- Interactive Learning: The interactive nature of these tools allows for a dynamic learning experience, providing additional clarifications or follow-up answers as needed.
At HireQuotient, our AI Answer Generator can help you quickly master Spring Boot interview questions by generating relevant answers based on the latest information. With over 110+ curated questions, this tool becomes an essential part of your preparation toolkit, allowing you to refine your knowledge and boost your confidence before stepping into the interview room.
Read More:
- Different types of Interview Questions
- 10 Common Job Interview Questions And What Answers To Be Expected
- The Hiring Journey: 100+ Good Interview Questions to Ask In An Interview in 2024
- Here Are The Top Interview Questions You Need To Add To Your Interview Now!
- Informational Interview Questions
- 11+ Unique Interview Questions To Ask Employer During The Interview
- A Complete Guide on the Best Interview Questions to Ask Candidates
- Market Executive Interview Questions and Answers
- 30+ Second Interview Questions To Ask Candidates
- Employee Satisfaction Sample Questions
- How to Answer Interview Questions? [By Mastering the Employer's Perspective]
- Video Interview Questions: The Different Categories and Types
- Common Exit Interview Questions
- Customer Success Trainer Interview Questions
- Data Analyst Interview Questions: Learn to hire the perfect data analyst
- 50+ Content Marketing Expert Interview Questions and Answers
- Enterprise Customer Success Manager Interview Questions and Answers
- Online Advertising Expert Interview Questions & Answers
- Questions to Ask When Hiring SEM Experts
- Top 110+ Selenium Interview Questions and Answers
Authors
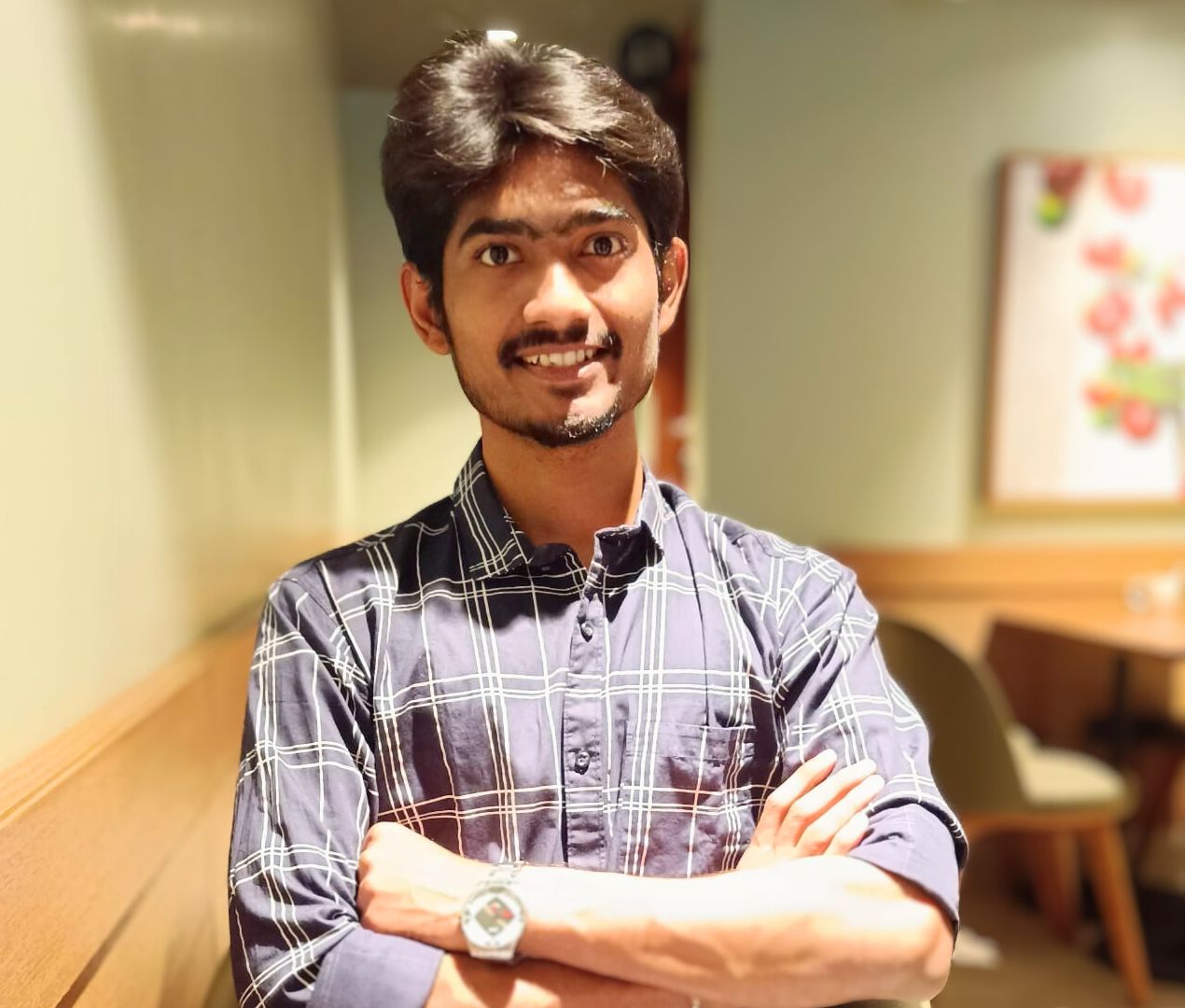
Yash Chaudhari
With a strong background as an SEO and Content Specialist, Yash excels in driving organic traffic, improving search engine rankings, and creating SEO-optimized content. He has a proven track record of implementing strategies that increase website traffic and conversions. Additionally, Yash is an automotive enthusiast and has a keen interest in astronomy.
Hire the best without stress
Ask us how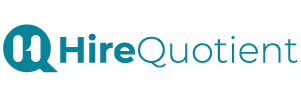
Never Miss The Updates
We cover all recruitment, talent analytics, L&D, DEI, pre-employment, candidate screening, and hiring tools. Join our force & subscribe now!
Stay On Top Of Everything In HR