50+ React Interview Questions
Published on May 3rd, 2024
Are you preparing for a React developer interview? As one of the most popular JavaScript libraries for building user interfaces, React is a critical skill to master in today's front-end development landscape. Thorough preparation is key to confidently tackling React interview questions and impressing potential employers with your knowledge.
In this comprehensive blog post, we've compiled 120 essential React interview questions and answers to help you succeed. Our curated list covers a wide range of topics, from basic React concepts to advanced techniques, providing you with the foundation needed to excel in your next React interview.
Whether you're a junior developer looking to land your first React role or a seasoned developer seeking to advance your career, understanding common React interview questions is crucial. Interviewers assess your problem-solving skills, familiarity with React best practices, and ability to articulate complex topics. By practicing these questions and understanding the underlying concepts, you'll be well-prepared to showcase your expertise.
To help you navigate this extensive collection, we've organized the React interview questions and answers into several key categories:
- Basic React Concepts
- JSX and Components
- Props and State
- Component Lifecycle
- Event Handling
- Lists and Keys
- Forms and Controlled Components
- Styling and CSS
- React Router
- React Hooks
- Context and State Management
- Performance Optimization
- Testing and Debugging
- Advanced Topics
Each section delves into specific aspects of React development, with interview questions of varying difficulty levels. Whether you're tackling fundamental concepts like component structure and JSX syntax or exploring advanced topics such as performance optimization and testing strategies, this blog post has you covered.
In addition to providing the React interview questions, we've included detailed answers and code examples to clarify key points. By studying these explanations, you'll reinforce your understanding of React's inner workings and best practices, equipping you with the knowledge needed to impress interviewers and stand out from other candidates.
Preparing for React interview questions requires consistent practice and hands-on experience. We encourage you to not only read through the questions and answers but also to code along and experiment with the provided examples. Building your own React projects and actively applying these concepts will deepen your understanding and help you develop the problem-solving skills interviewers seek.
Remember, the goal is not just to memorize answers but to truly grasp the underlying concepts and principles behind React development. By dedicating time to thoroughly preparing for these React interview questions, you'll enhance your skills, boost your confidence, and increase your chances of acing your next React interview.
So, let's dive in and explore the 120 essential React interview questions and answers that will set you on the path to success in your React developer career!
React Interview Questions: Basic React Concepts
1. What is React, and what are its key features?
Answer: React is a JavaScript library for building user interfaces. Its key features include:
- Component-based architecture: React allows you to break down the UI into reusable components.
- Virtual DOM: React uses a virtual representation of the DOM for efficient updates and rendering.
- Declarative syntax: React uses a declarative approach, making code more readable and maintainable.
- Unidirectional data flow: React follows a one-way data flow, ensuring a predictable and manageable application state
2. What are the differences between a class component and a functional component in React?
Answer: The main differences between class components and functional components are:
- Syntax: Class components are defined using ES6 classes, while functional components are simple JavaScript functions.
- State and lifecycle: Class components have their own state and lifecycle methods, whereas functional components rely on hooks (starting from React 16.8) for state management and lifecycle-like functionality.
this keyword: Class components use the this keyword to access props and state, while functional components receive props as function arguments.
3. Explain the concept of virtual DOM in React.
Answer: The virtual DOM is a lightweight JavaScript representation of the actual DOM. When changes occur in a React component, the virtual DOM is updated first. React then compares the updated virtual DOM with the previous version to determine the minimal set of changes needed in the actual DOM. This process is called "reconciliation." By minimizing direct manipulations of the real DOM, React achieves better performance and provides a more efficient way to update the UI.
4. What is JSX, and why is it used in React?
Answer: JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files. It provides a concise and intuitive way to describe the structure and appearance of React components. JSX is compiled into regular JavaScript function calls that create React elements. By using JSX, you can keep your component's logic and markup together, making your code more readable and maintainable.
5, What is the significance of the "key" prop when rendering lists in React?
Answer: When rendering a list of elements in React, it's important to provide a unique "key" prop for each element. The "key" helps React identify which items have been added, removed, or modified in the list. It allows React to efficiently update and reorder the elements without unnecessarily re-rendering the entire list. The "key" should be a stable identifier, such as a unique ID from your data. Using proper keys improves the performance and maintainability of your React application.
React Interview Questions: JSX and Components
6. What are the differences between rendering a React component as an element and as a function?
Answer: When rendering a React component, you have two options:
- Rendering as an element: You can render a component as a JSX element using the component's name as a tag, like <MyComponent />. This creates an instance of the component and renders it in the specified location.
- Rendering as a function: You can also render a component by calling it as a function, like {MyComponent()}. This approach is useful when you want to pass the result of the component directly to another component or when you need to conditionally render a component.7How do you pass data between React components?
There are several ways to pass data between React components:
- Props: Props are the most common way to pass data from a parent component to its child components. You can pass data as attributes when rendering the child component, and the child component can access those props through its props object.
- Context: Context provides a way to pass data through the component tree without having to pass props manually at every level. It allows you to share data that is considered global for a tree of React components.
- State management libraries: Libraries like Redux or MobX can be used to manage the application state in a centralized store. Components can access and update the state through defined actions and selectors.
- Callbacks: You can pass callback functions as props from a parent component to its child components. The child components can then invoke those callbacks to pass data back to the parent component.
7. What is the difference between props and state in React?
Answer: Props and state are both used to store and manage data in React components, but they serve different purposes:
- Props (short for "properties") are read-only data that are passed from a parent component to a child component. They are used to configure and customize the behavior and appearance of a component. Props cannot be modified by the child component itself.
- State, on the other hand, is mutable data that is managed within a component. It represents the internal state of a component and can change over time. When the state changes, React automatically re-renders the component to reflect the updated state. State is typically used for interactivity, user input, or data that changes dynamically.
8. How do you conditionally render components in React?
Answer: In React, you can conditionally render components using JavaScript conditional statements or ternary operators. Here are a few approaches:
- If-else statements: You can use traditional if-else statements to conditionally render components based on certain conditions.
- Ternary operator: The ternary operator is a concise way to conditionally render components. It follows the syntax condition ? trueComponent : falseComponent.
- Logical AND operator: You can use the logical AND operator (&&) to conditionally render a component. If the condition before && is true, the component after && will be rendered.
- Returning null: If you want to conditionally hide a component, you can return null instead of rendering the component.
9. What is the purpose of the "children" prop in React components?
Answer: The "children" prop is a special prop in React that allows you to pass content between the opening and closing tags of a component. It enables you to compose components by nesting them inside each other. The "children" prop can be a string, a React element, or multiple React elements. It provides a way to create reusable and flexible components that can accept and render dynamic content.
10. How do you handle events in React components?
Answer: In React, you can handle events using event handlers. Event handlers are functions that are triggered when a specific event occurs, such as a button click or form submission. To handle events in React:
- Define an event handler function in your component.
- Bind the event handler to the desired event using the appropriate event attribute, such as onClick, onSubmit, onChange, etc.
- Pass the event handler function as the value of the event attribute.
- Inside the event handler function, you can access the event object and perform the necessary actions.
11. What are the benefits of using React fragments?
Answer: React fragments allow you to group multiple elements without adding an extra DOM node. They provide a way to return multiple elements from a component's render method without wrapping them in a parent element. The benefits of using fragments include:
- Improved performance: Fragments don't create an additional DOM node, reducing the memory usage and rendering overhead.
- Cleaner and more semantic markup: Fragments help keep the HTML structure clean and meaningful, especially when returning multiple elements.
- Flexibility in component composition: Fragments enable you to compose components more flexibly without the need for unnecessary wrapper elements.
12. How do you handle form inputs in React?
Answer: In React, form inputs can be handled using controlled components or uncontrolled components.
- Controlled components: In a controlled component, the value of the form input is controlled by React state. You define the state for the input value and update it using an event handler. The input's value is always in sync with the component's state.
- Uncontrolled components: In an uncontrolled component, the form input's value is managed by the DOM itself. You can access the input's value using a ref and retrieve it when needed, such as on form submission. Controlled components provide more control and validation capabilities, while uncontrolled components can be simpler to implement for basic form inputs.
13. What are the differences between controlled and uncontrolled components in React?
Answer: The main differences between controlled and uncontrolled components are:
- Value management: In controlled components, the value of the form input is managed by React state, while in uncontrolled components, the value is managed by the DOM.
- Event handling: Controlled components require event handlers to update the state whenever the input value changes, whereas uncontrolled components rely on refs to retrieve the input value.
- Validation and control: Controlled components provide more control over form inputs, allowing for real-time validation and updates. Uncontrolled components are simpler but offer less control and validation capabilities.
14. How do you communicate between sibling components in React?
Answer: To communicate between sibling components in React, you can use the following approaches:
- Lifting the state up: Move the shared state to the nearest common parent component and pass it down as props to the sibling components. The parent component can also pass down callback functions to update the state.
- Context: Use React's Context API to create a shared context that can be accessed by the sibling components. The context can hold the data and functions required for communication between the siblings.
- State management libraries: Utilize state management libraries like Redux or MobX to create a global store that can be accessed by any component in the application. The sibling components can dispatch actions and subscribe to the store to communicate with each other.
React Interview Questions: Props and State
15. What is the purpose of props in React?
Answer: Props (short for "properties") in React are used to pass data from a parent component to its child components. They allow you to configure and customize the behavior and appearance of a component. Props are read-only and cannot be modified by the child component itself. They provide a way to make components reusable and modular by separating the component's configuration from its implementation.
16. How do you pass props to a component in React?
Answer: To pass props to a component in React, you can use the following syntax:
- When rendering a component, specify the prop name and its value as an attribute: <MyComponent propName="propValue" />.
- In the component definition, access the props using the props object: function MyComponent(props) { ... }.
- Use the dot notation to access individual props: props.propName.
- If you are using class components, access props using this.props: this.props.propName.
17. What are the different ways to initialize state in a React component?
Answer: There are two main ways to initialize state in a React component:
- Class components:
- Define the initial state in the constructor method using this.state: constructor(props) { super(props); this.state = { ... }; }.
- Use the class field syntax to define the initial state: state = { ... }.
- Functional components with hooks:
- Use the useState hook to initialize state: const [stateName, setStateName] = useState(initialValue);.
- You can initialize state with any JavaScript value, such as strings, numbers, objects, or arrays.
18. How do you update state in a React component?
Answer: To update state in a React component, you should use the setState method or the state updater function provided by the useState hook:
- Class components:
- Use this.setState({ ... }) to update the state. Pass an object with the updated state properties.
- setState merges the provided object with the current state, triggering a re-render of the component.
- Functional components with hooks:
- Use the state updater function returned by the useState hook: setStateName(newValue).
- The state updater function replaces the previous state value with the new value. It's important to note that you should never modify the state directly (e.g., this.state.property = value) as it won't trigger a re-render and can lead to unexpected behavior.
19. What is the difference between state and props?
Answer: The main differences between state and props are:
- Ownership: State is owned and managed by the component itself, while props are passed down from a parent component.
- Mutability: State can be changed within the component using setState or the state updater function, whereas props are read-only and cannot be modified by the component receiving them.
- Usage: State is used to store and manage data that can change within a component, while props are used to pass data and configuration from a parent component to its child components.
- Initialization: State is initialized within the component, either in the constructor (for class components) or using the useState hook (for functional components). Props are passed to the component from its parent.
20. How do you pass data from a child component to its parent in React?
Answer: To pass data from a child component to its parent in React, you can use the following approach:
- Define a callback function in the parent component that accepts the data as a parameter.
- Pass the callback function as a prop to the child component.
- In the child component, invoke the callback function with the relevant data whenever needed (e.g., on a button click or form submission).
- The parent component receives the data through the callback function and can update its own state or perform any other necessary actions.
21. What is the purpose of the "key" prop when rendering lists in React?
Answer: The "key" prop is used when rendering lists of elements in React to help identify each element uniquely. It allows React to efficiently update and reorder the list elements without unnecessarily re-rendering the entire list. The "key" should be a stable identifier, such as a unique ID from your data. The significance of using proper keys in lists includes:
- Performance optimization: React uses keys to determine which elements have changed, been added, or removed. By using unique and stable keys, React can efficiently update only the necessary elements.
- Maintaining element state: Keys help preserve the state of individual elements across re-renders. Without proper keys, React may incorrectly update or lose the state of elements.
- Avoiding unexpected behavior: Using unstable or non-unique keys can lead to unexpected behavior, such as elements being duplicated or incorrectly reordered.
22. How do you handle form submissions in React?
Answer: To handle form submissions in React, you can follow these steps:
- Create a form component with the necessary input fields.
- Use controlled components for the input fields by managing their values through state.
- Define an event handler for the form submission event (e.g., onSubmit).
- In the event handler, prevent the default form submission behavior using event.preventDefault().
- Collect the form data from the component's state or refs.
- Perform any necessary data validation or processing.
- Submit the form data to a server or handle it within the application as needed.
- Optionally, reset the form fields after successful submission.
23. What are the benefits of using PropTypes in React?
Answer: PropTypes is a built-in type-checking feature in React that allows you to specify the type and shape of props expected by a component. The benefits of using PropTypes include:
- Documentation: PropTypes serve as a form of documentation, clearly defining the expected props and their types for a component. This makes it easier for other developers to understand and use the component correctly.
- Runtime type checking: PropTypes perform runtime type checking on the props passed to a component. If a prop doesn't match the specified type, React will generate a warning in the console, helping you catch potential bugs early in the development process.
- Improved code quality: By explicitly defining the expected props and their types, PropTypes encourages you to write cleaner and more maintainable code. It helps prevent passing incorrect or missing props to components.
- Better development experience: IDEs and code editors can provide autocompletion and type hinting based on the defined PropTypes, enhancing the development experience and reducing the chances of making typing mistakes.
24. How do you handle asynchronous data fetching in React components?
Answer: There are several approaches to handle asynchronous data fetching in React components:
- ComponentDidMount lifecycle method (for class components):
- Fetch data in the componentDidMount method using APIs like fetch or libraries like Axios.
- Update the component's state with the fetched data using setState.
- Render the component based on the updated state.
- useEffect hook (for functional components):
- Use the useEffect hook to perform side effects, such as data fetching, after the component has rendered.
- Specify an empty dependency array [] to ensure the effect runs only once, similar to componentDidMount.
- Update the component's state using the state updater function returned by the useState hook.
- Libraries and tools:
- Use libraries like React Query or SWR to simplify data fetching and caching in React components.
- These libraries provide hooks and utilities for handling asynchronous data, managing loading states, and caching results. Regardless of the approach, it's important to handle loading states, error states, and data updates gracefully in your components to provide a good user experience.
React Interview Questions: Component Lifecycle
25. What is the component lifecycle in React?
Answer: The component lifecycle in React refers to the series of methods that are invoked in a specific order during the lifetime of a component. The lifecycle methods allow you to hook into different stages of a component's existence and perform actions accordingly. The main lifecycle phases are:
- Mounting: When a component is being added to the DOM.
- constructor(): Initializes the component's state and binds event handlers.
- render(): Returns the JSX to be rendered.
- componentDidMount(): Runs after the component has been mounted to the DOM. Used for side effects like data fetching or subscriptions.
- Updating: When a component's props or state change.
- render(): Re-renders the component with the updated props or state.
- componentDidUpdate(): Runs after the component has updated. Used for performing side effects based on prop or state changes.
- Unmounting: When a component is being removed from the DOM.
- componentWillUnmount(): Runs before the component is unmounted. Used for cleanup tasks like canceling subscriptions or timers.
26. What is the purpose of the componentDidMount lifecycle method?
Answer: The componentDidMount lifecycle method is invoked immediately after a component is mounted to the DOM. It is commonly used for performing side effects or initialization tasks that require the component to be fully rendered. Some common use cases for componentDidMount include: - Fetching data from an API or server. - Setting up subscriptions or event listeners. - Initializing third-party libraries or plugins. - Measuring DOM elements or interacting with the browser API. It's important to note that the component's state and props are available in componentDidMount, making it a suitable place to perform actions that depend on the component's initial state or props.
27. What is the purpose of the componentDidUpdate lifecycle method?
Answer: The componentDidUpdate lifecycle method is invoked immediately after a component's updates are flushed to the DOM. It is triggered when the component's props or state change, but not during the initial render. The componentDidUpdate method receives the previous props and state as arguments, allowing you to compare them with the current props and state. Common use cases for componentDidUpdate include:
- Performing side effects based on prop or state changes.
- Updating the DOM or interacting with browser APIs in response to prop or state changes.
- Fetching new data when specific props change.
- Triggering animations or other visual updates. It's important to include a condition to check if the relevant props or state have actually changed before performing any side effects to avoid unnecessary operations.
28. What is the purpose of the componentWillUnmount lifecycle method?
Answer: The componentWillUnmount lifecycle method is invoked immediately before a component is unmounted and destroyed. It is used for performing cleanup tasks to prevent memory leaks or unexpected behavior.
Common use cases for componentWillUnmount include:
- Canceling any active network requests or subscriptions.
- Removing event listeners or timers.
- Cleaning up any manually created DOM elements or references.
- Resetting any global state or cache that the component may have modified. By performing necessary cleanup in componentWillUnmount, you ensure that your component doesn't leave behind any unnecessary operations or resources when it is no longer in use.
29. How do you conditionally render components based on state or props?
Answer: You can conditionally render components in React based on state or props using conditional statements or ternary operators within the render method or JSX. Here are a few approaches:
- If-else statements:
JSX

render() {
if (this.state.condition) {
return <ComponentA />;
} else {
return <ComponentB />;
}
}
- Ternary operator:
JSX

render() {
return this.props.condition ? <ComponentA /> : <ComponentB />;
}
- Logical AND operator:
JSX

render() {
return this.state.condition && <ComponentA />;
}
Variables:
JSX

render() {
const componentToRender = this.props.condition ? <ComponentA /> : <ComponentB />;
return <div>{componentToRender}</div>;
}
30. How do you optimize the performance of a React component? Answer: There are several techniques to optimize the performance of a React component:
- Use React.memo or PureComponent: Wrap functional components with React.memo or extend class components from PureComponent to avoid unnecessary re-renders when the props haven't changed.
- Memoize expensive computations: Use memoization techniques like useMemo or useCallback hooks to cache expensive computations or callback functions and avoid redundant calculations.
- Lazy loading components: Use React.lazy and Suspense to lazily load components that are not immediately needed, reducing the initial bundle size and improving the application's loading time.
- Virtualize long lists: Implement virtualization techniques like react-window or react-virtualized to efficiently render large lists or grids by only rendering the visible items.
- Avoid excessive prop drilling: Minimize passing props through multiple levels of components by using composition, context, or state management libraries like Redux or MobX.
- Optimize images and assets: Compress and optimize images, CSS, and other static assets to reduce the overall bundle size and improve loading times.
- Use production build: Make sure to use the production build of your application, which applies various optimizations like minification and dead code elimination.
- Profiling and measuring: Use React DevTools and profiling tools to identify performance bottlenecks and optimize the critical paths of your application.
31. What is the purpose of shouldComponentUpdate lifecycle method?
Answer: The shouldComponentUpdate lifecycle method is used to optimize the performance of a React component by determining whether the component needs to re-render when its props or state change. It receives the next props and next state as arguments and returns a boolean value indicating whether the component should update or not. By default, React components will re-render whenever their props or state change. However, in some cases, the changes may not affect the component's output, and re-rendering can be unnecessary. The shouldComponentUpdate method allows you to manually control the re-rendering behavior based on specific conditions. Here's an example of how shouldComponentUpdate can be used:In this example, the shouldComponentUpdate method compares the current props and state with the next props and state. If the value prop or the count state has changed, it returns true, indicating that the component should re-render. Otherwise, it returns false, preventing unnecessary re-renders. By implementing shouldComponentUpdate and carefully comparing the relevant props and state, you can optimize the performance of your React components by avoiding redundant re-renders.
JSX

shouldComponentUpdate(nextProps, nextState) {
// Compare the current props and state with the next props and state
// Return true if the component should update, false otherwise
return nextProps.value !== this.props.value || nextState.count !== this.state.count;
}
32. How do you handle errors in React components?
Answer: Error handling in React components can be done using error boundaries. Error boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed. To create an error boundary, you define a class component with either the static getDerivedStateFromError lifecycle method or the componentDidCatch lifecycle method, or both.
Here's an example:
JSX

class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// You can log the error or send it to an error reporting service.
console.error('Error:', error);
console.error('Error Info:', errorInfo);
}
render() {
if (this.state.hasError) {
// Render fallback UI when an error occurs.
return <h1>Something went wrong.</h1>;
}
// Render the child components when there are no errors.
return this.props.children;
}
}
In this example, the ErrorBoundary component defines the getDerivedStateFromError method to update its state when an error is caught, and the componentDidCatch method to log the error or send it to an error reporting service. You can wrap the ErrorBoundary component around other components to catch and handle errors that occur within their child component tree:
JSX

<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
By using error boundaries, you can gracefully handle errors in your React components, display a fallback UI, and prevent the entire application from crashing due to an error in a specific component.
33. What is the purpose of the key prop in React lists?
Answer: The key prop is a special string attribute that you should include when rendering arrays of elements in React. It helps React identify which items in a list have changed, been added, or been removed, allowing for efficient updates and reconciliation of the component tree. When rendering a list of elements, each element should have a unique key prop. The key should be a stable identifier that uniquely identifies each item in the list. Commonly, you can use the item's ID or a combination of the item's properties as the key. Here's an example of rendering a list with keys:In this example, the index of each item is used as the key. However, using the index as the key is not recommended if the order of items may change, as it can lead to issues with component state and performance. The significance of using keys in React lists includes:
- Efficient updates: React uses keys to determine which items have changed, been added, or been removed, allowing for optimized updates and re-rendering of only the necessary components.
- Preserving component state: Keys help React preserve the state of individual components when their position in the list changes.
- Avoiding unexpected behavior: Using proper keys ensures that React correctly matches the components with their corresponding DOM elements, preventing unexpected behavior or loss of state. It's important to note that keys should be unique among siblings in a list, but they don't need to be globally unique. Keys are not passed as props to the components themselves; they are only used by React internally for efficient list rendering and reconciliation.
JSX

const items = ['Apple', 'Banana', 'Orange'];
const listItems = items.map((item, index) =>
<li key={index}>{item}</li>
);
34. How do you use refs in React?
Answer: Refs in React provide a way to access and manipulate DOM elements or React components directly. They are commonly used in scenarios where you need to perform imperative operations, such as focusing an input field, triggering animations, or integrating with third-party libraries. To use refs in React, you can follow these steps:
- Create a ref using the useRef hook (for functional components) or the React.createRef() method (for class components).
- Attach the ref to a DOM element or a React component using the ref attribute.
- Access the DOM element or component instance through the current property of the ref. Here's an example of using refs in a functional component:
React Interview Questions: Event Handling
35. How do you handle events in React?
Answer: In React, you handle events by attaching event handlers to elements. Event handlers are functions that are called when a specific event occurs, such as a button click or a form submission. React uses a synthetic event system that wraps the native browser events, providing a consistent interface across different browsers. Here are the steps to handle events in React:
- Define an event handler function in your component.
- Attach the event handler to the desired element using an event prop.
- Pass the event handler function as the value of the event prop. Here's an example of handling a button click event in a functional component:
JSX

import React from 'react';
function MyComponent() {
const handleClick = () => {
console.log('Button clicked!');
};
return <button onClick={handleClick}>Click Me</button>;
}
In this example, we define an event handler function called handleClick. We attach the handleClick function to the button element using the onClick event prop. When the button is clicked, the handleClick function will be called, and the message "Button clicked!" will be logged to the console.
For class components, you can define event handlers as class methods and bind them to the component instance using the bind method or arrow function syntax. Here's an example:

import React from 'react';
function MyComponent() {
const handleClick = () => {
console.log('Button clicked!');
};
return <button onClick={handleClick}>Click Me</button>;
}
36. How do you pass arguments to event handlers in React?
Answer: In React, you can pass arguments to event handlers in a few different ways. Here are the common approaches:
1. Using Arrow Functions: You can use an arrow function to wrap the event handler and pass arguments to it. The arrow function will be called when the event occurs, and it will invoke the actual event handler with the desired arguments.
JSX

<button onClick={() => handleClick(id)}>Click Me</button>
In this example, handleClick is the event handler function, and id is the argument you want to pass to it. The arrow function () => handleClick(id) will be called when the button is clicked, and it will invoke handleClick with the id argument.
2. Using the bind Method: You can use the bind method to partially apply arguments to the event handler function. The bind method returns a new function that has the specified arguments preset.
JSX

<button onClick={handleClick.bind(this, id)}>Click Me</button>
In this case, handleClick is the event handler function, and id is the argument you want to pass to it. The bind method is used to create a new function that has this set to the current component instance and id as the first argument.
3. Passing Arguments in the Event Handler: If you need access to the event object along with additional arguments, you can define the event handler function with the event as the first parameter and the additional arguments as subsequent parameters.
JSX

<button onClick={(event) => handleClick(event, id)}>Click Me</button>
In this example, handleClick is the event handler function that receives the event object as the first argument and id as the second argument. The arrow function (event) => handleClick(event, id) will be called when the button is clicked, and it will invoke handleClick with the event and id arguments. It's important to note that when using arrow functions or the bind method, a new function is created each time the component renders. If the event handler is passed as a prop to child components, it can cause unnecessary re-renders. In such cases, it's recommended to use class methods or memoize the event handler using hooks like useCallback to optimize performance.
36. What is the difference between controlled and uncontrolled components in React?
Answer: In React, the terms "controlled" and "uncontrolled" refer to how form inputs are handled in a component.
Controlled Components:
- In a controlled component, the value of the form input is controlled by React through the component's state.
- The component renders the form input with the value from its state and updates the state when the input value changes.
- The component has full control over the input's value and can perform validations, transformations, or other operations on the input data.
- The value of the input is always in sync with the component's state.
- Example:
- In an uncontrolled component, the form input's value is handled by the DOM itself, and React does not have direct control over it.
- The component uses a ref to access the input's value when needed, such as on form submission.
- The input's value is not stored in the component's state, and the component does not track changes to the input's value.
- Example:
JSX

import React, { useState } from 'react';
function ControlledInput() {
const [value, setValue] = useState('');
const handleChange = (event) => {
setValue(event.target.value);
};
return <input type="text" value={value} onChange={handleChange} />;
}
Uncontrolled Components:
- In an uncontrolled component, the form input's value is handled by the DOM itself, and React does not have direct control over it.
- The component uses a ref to access the input's value when needed, such as on form submission.
- The input's value is not stored in the component's state, and the component does not track changes to the input's value.
- Example:
JSX

The choice between controlled and uncontrolled components depends on the specific requirements of your form and how much control you need over the form inputs. Controlled components provide more control and allow for easier validation and manipulation of input data, while uncontrolled components can be simpler to implement for basic form inputs.
37. How do you prevent default behavior in event handlers?
Answer: In React, you can prevent the default behavior of an event by calling the preventDefault() method on the event object. This is commonly used when handling form submissions or preventing link navigation. Here's an example of preventing the default form submission behavior:In this example, when the form is submitted, the handleSubmit event handler is called. Inside the event handler, we call event.preventDefault() to prevent the default form submission behavior, which would typically refresh the page. Similarly, you can prevent the default behavior of a link click event to handle custom navigation logic:In this case, when the link is clicked, the handleClick event handler is called. By calling event.preventDefault(), we prevent the default behavior of navigating to the specified URL. Instead, we can handle custom navigation logic or perform any other desired actions. It's important to note that not all events have a default behavior that needs to be prevented. The preventDefault() method is primarily used for events that have a default action associated with them, such as form submissions, link clicks, or checkbox toggles.
JSX

import React from 'react';
function MyForm() {
const handleSubmit = (event) => {
event.preventDefault();
// Handle form submission logic here
console.log('Form submitted!');
};
return (
<form onSubmit={handleSubmit}>
<input type="text" />
<button type="submit">Submit</button>
</form>
);
}
In this example, when the form is submitted, the handleSubmit event handler is called. Inside the event handler, we call event.preventDefault() to prevent the default form submission behavior, which would typically refresh the page. Similarly, you can prevent the default behavior of a link click event to handle custom navigation logic:

import React from 'react';
function MyLink() {
const handleClick = (event) => {
event.preventDefault();
// Handle custom navigation logic here
console.log('Link clicked!');
};
return <a href="/some-link" onClick={handleClick}>Click Me</a>;
}
In this case, when the link is clicked, the handleClick event handler is called. By calling event.preventDefault(), we prevent the default behavior of navigating to the specified URL. Instead, we can handle custom navigation logic or perform any other desired actions. It's important to note that not all events have a default behavior that needs to be prevented. The preventDefault() method is primarily used for events that have a default action associated with them, such as form submissions, link clicks, or checkbox toggles.
38. How do you handle multiple inputs in a form in React?
Answer: When handling multiple inputs in a form in React, you can create a single event handler function that updates the component's state based on the input's name and value. Here's an example of how you can handle multiple inputs in a form using a controlled component approach:

import React, { useState } from 'react';
function MyForm() {
const [formData, setFormData] = useState({
name: '',
email: '',
message: '',
});
const handleChange = (event) => {
const { name, value } = event.target;
setFormData((prevFormData) => ({
...prevFormData,
[name]: value,
}));
};
const handleSubmit = (event) => {
event.preventDefault();
// Handle form submission logic here
console.log(formData);
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
placeholder="Name"
/>
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
placeholder="Email"
/>
<textarea
name="message"
value={formData.message}
onChange={handleChange}
placeholder="Message"
/>
<button type="submit">Submit</button>
</form>
);
}
In this example, we create a state object called formData using the useState hook to store the values of the form inputs. The initial state is an object with properties corresponding to each input's name (name, email, message). We define a single event handler function called handleChange that is attached to the onChange event of each input. When an input value changes, the handleChange function is called with the event object. Inside the handleChange function, we destructure the name and value properties from the event target (input element). We then update the formData state using the setFormData function and the spread operator (...). We create a new object that spreads the previous form data (prevFormData) and updates the specific property based on the input's name using the computed property syntax ([name]: value). By using the name attribute on each input element and matching it with the corresponding property in the formData state, we can dynamically update the state based on the input that triggered the change event. Finally, when the form is submitted, the handleSubmit function is called. We prevent the default form submission behavior using event.preventDefault() and can access the form data from the formData state object to perform any necessary actions, such as sending the data to a server or performing validations. This approach allows you to handle multiple inputs in a form efficiently by maintaining a single state object and using a single event handler function.
39. What is the purpose of the onSubmit event in forms?
Answer: The onSubmit event in forms is used to handle the form submission event in React. It is triggered when the user submits the form, typically by clicking a submit button or pressing the Enter key while focusing on an input field within the form. The onSubmit event allows you to define a custom event handler function that will be called when the form is submitted. In this event handler, you can perform various actions such as:
- Collecting and validating form data
- Sending form data to a server or API
- Performing client-side validation
- Updating the component's state or triggering other actions based on the form submission Here's an example of using the onSubmit event in a form:
JSX

import React from 'react';
function MyForm() {
const handleSubmit = (event) => {
event.preventDefault();
// Collect form data
const formData = new FormData(event.target);
const name = formData.get('name');
const email = formData.get('email');
// Perform form submission logic here
console.log('Form submitted!');
console.log('Name:', name);
console.log('Email:', email);
};
return (
<form onSubmit={handleSubmit}>
<input type="text" name="name" placeholder="Name" />
<input type="email" name="email" placeholder="Email" />
<button type="submit">Submit</button>
</form>
);
}
In this example, we define a handleSubmit function as the event handler for the onSubmit event. When the form is submitted, the handleSubmit function is called. Inside the handleSubmit function, we first call event.preventDefault() to prevent the default form submission behavior, which would typically refresh the page. We then collect the form data using the FormData API. We create a new FormData object by passing the event.target (the form element) to its constructor. We can then retrieve individual form field values using the get method of the FormData object, specifying the name attribute of each input field. After collecting the form data, you can perform any necessary form submission logic, such as sending the data to a server, validating the inputs, or updating the component's state. By using the onSubmit event and defining a custom event handler, you have full control over the form submission process and can handle the submitted data according to your application's requirements.
40. How do you handle form validation in React?
Answer: Form validation in React can be handled in different ways depending on your specific requirements and the complexity of your form. Here are a few common approaches to form validation in React:
Controlled Components with State:
- Use controlled components to manage the form inputs' values through the component's state.
- Validate the form inputs whenever the state changes or on form submission.
- Display validation error messages to the user based on the validation results.
- Example:

import React, { useState } from 'react';
function MyForm() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const [errors, setErrors] = useState({});
const handleChange = (event) => {
const { name, value } = event.target;
if (name === 'name') {
setName(value);
} else if (name === 'email') {
setEmail(value);
}
};
const handleSubmit = (event) => {
event.preventDefault();
const validationErrors = {};
if (!name) {
validationErrors.name = 'Name is required';
}
if (!email) {
validationErrors.email = 'Email is required';
}
setErrors(validationErrors);
if (Object.keys(validationErrors).length === 0) {
// Submit the form
console.log('Form submitted!');
}
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
name="name"
value={name}
onChange={handleChange}
placeholder="Name"
/>
{errors.name && <span>{errors.name}</span>}
<input
type="email"
name="email"
value={email}
onChange={handleChange}
placeholder="Email"
/>
{errors.email && <span>{errors.email}</span>}
<button type="submit">Submit</button>
</form>
);
}
Using a Validation Library:
- Utilize a form validation library such as Formik, Yup, or React Hook Form to handle form validation.
- These libraries provide a declarative way to define form validation rules and handle form state management.
- They often come with built-in validation functions and error handling mechanisms.
- Example using Formik:

import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
function MyForm() {
const initialValues = {
name: '',
email: '',
};
const validationSchema = Yup.object().shape({
name: Yup.string().required('Name is required'),
email: Yup.string().email('Invalid email').required('Email is required'),
});
const handleSubmit = (values, { setSubmitting }) => {
// Submit the form
console.log('Form submitted!', values);
setSubmitting(false);
};
return (
<Formik
initialValues={initialValues}
validationSchema={validationSchema}
onSubmit={handleSubmit}
>
{({ isSubmitting }) => (
<Form>
<Field type="text" name="name" placeholder="Name" />
<ErrorMessage name="name" component="div" />
<Field type="email" name="email" placeholder="Email" />
<ErrorMessage name="email" component="div" />
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
);
}
Custom Validation Functions:
- Create custom validation functions to validate form inputs based on specific criteria.
- Call these validation functions on form submission or whenever the form inputs change.
- Display validation error messages based on the validation results.
- Example:
JSX

import React, { useState } from 'react';
function validateEmail(email) {
// Custom email validation logic
const re = /\S+@\S+\.\S+/;
return re.test(email);
}
function MyForm() {
const [email, setEmail] = useState('');
const [emailError, setEmailError] = useState('');
const handleChange = (event) => {
setEmail(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
if (!validateEmail(email)) {
setEmailError('Invalid email');
} else {
setEmailError('');
// Submit the form
console.log('Form submitted!');
}
};
return (
<form onSubmit={handleSubmit}>
<input
type="email"
value={email}
onChange={handleChange}
placeholder="Email"
/>
{emailError && <span>{emailError}</span>}
<button type="submit">Submit</button>
</form>
);
}
These are just a few examples of how you can handle form validation in React. The approach you choose depends on the complexity of your form, the specific validation requirements, and your personal preference. Regardless of the approach, the key is to validate user input, provide meaningful error messages, and ensure a smooth user experience during form submission.
41. What is the purpose of the onChange event in form inputs?
Answer: The onChange event in form inputs is used to handle the change event that occurs whenever the value of an input element changes. It allows you to capture and respond to user input in real-time as they interact with the form. In React, the onChange event is commonly used in controlled components to update the component's state with the latest value of the input element. By attaching an onChange event handler to an input, you can track the changes made by the user and keep the component's state in sync with the input's value. Here's an example of using the onChange event in a controlled input component:In this example, we create a controlled input component called MyInput. We use the useState hook to manage the component's state, specifically the value state variable. We attach an onChange event handler to the input element using the onChange prop. The handleChange function is called whenever the value of the input changes, such as when the user types or deletes characters. Inside the handleChange function, we receive the event object as a parameter, which contains information about the change event. We access the current value of the input using event.target.value and update the `
JSX

import React, { useState } from 'react';
function MyInput() {
const [value, setValue] = useState('');
const handleChange = (event) => {
setValue(event.target.value);
};
return <input type="text" value={value} onChange={handleChange} />;
}
In this example, we create a controlled input component called MyInput. We use the useState hook to manage the component's state, specifically the value state variable. We attach an onChange event handler to the input element using the onChange prop. The handleChange function is called whenever the value of the input changes, such as when the user types or deletes characters.
Inside the handleChange function, we receive the event object as a parameter, which contains information about the change event. We access the current value of the input using event.target.value and update the valuestate variable using thesetValuefunction. This ensures that the component's state always reflects the current value of the input. By using theonChangeevent and updating the component's state, you can perform various actions based on the user's input, such as: - Validating the input value - Formatting or transforming the input value - Triggering other actions or updating other parts of the component's state - Enabling or disabling form submission based on the input's validity TheonChangeevent is not limited to text inputs; it can be used with other form elements as well, such as checkboxes, radio buttons, and select dropdowns. The event object provides access to the relevant information about the changed element, allowing you to handle different types of inputs accordingly. In summary, theonChange` event in form inputs is crucial for capturing and responding to user input in real-time, enabling you to create interactive and dynamic forms in React.
42. How do you handle form submission without a page refresh?
Answer: To handle form submission without a page refresh in React, you can use the onSubmit event handler and prevent the default form submission behavior. By preventing the default behavior, you can send the form data to the server or perform other actions without triggering a page reload. Here's an example of handling form submission without a page refresh:
JSX

import React, { useState } from 'react';
function MyForm() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
// Send form data to the server or perform other actions
console.log('Form submitted!');
console.log('Name:', name);
console.log('Email:', email);
// Reset form fields
setName('');
setEmail('');
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
placeholder="Name"
/>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
placeholder="Email"
/>
<button type="submit">Submit</button>
</form>
);
}
In this example, we create a form component called MyForm. We use the useState hook to manage the component's state for the name and email form fields. We attach an onSubmit event handler to the form element using the onSubmit prop. The handleSubmit function is called when the form is submitted. Inside the handleSubmit function, we first call event.preventDefault() to prevent the default form submission behavior, which would typically trigger a page refresh. After preventing the default behavior, we can perform any desired actions with the form data, such as sending it to a server using an API request or updating the component's state. In this example, we simply log the form data to the console. Finally, we reset the form fields by setting the name and email state variables back to empty strings using the setName and setEmail functions. This clears the form inputs after submission. By handling form submission in this way, you can process the form data without triggering a page refresh, providing a smoother user experience and allowing for more dynamic interactions within your React application. Remember to adapt the form handling logic based on your specific requirements, such as performing data validation, showing success or error messages, or redirecting the user to another page after successful submission.
43. What is the difference between onBlur and onFocus events in form inputs?
Answer: The onBlur and onFocus events in form inputs are used to handle the focus-related events of an input element.
- onBlur event:
- The onBlur event is triggered when an input element loses focus, meaning when the user moves the focus away from the input by clicking outside of it or by using the keyboard (e.g., pressing the Tab key).
- It is commonly used to perform validations or trigger actions when the user finishes interacting with an input.
- Example usage:

<input
type="text"
onBlur={(event) => {
console.log('Input lost focus');
// Perform validations or trigger actions
}}
/>
- onFocus event:
- The onFocus event is triggered when an input element receives focus, meaning when the user clicks on the input or navigates to it using the keyboard (e.g., pressing the Tab key).
- It is commonly used to perform actions when the user starts interacting with an input, such as clearing placeholder text or displaying additional information.
- Example usage:

- onBlur is triggered when the input loses focus, indicating that the user has finished interacting with the input.
- onFocus is triggered when the input receives focus, indicating that the user is about to start interacting with the input. These events can be useful in various scenarios, such as:
- Performing form validations: You can use the onBlur event to validate the input value when the user moves away from the input, providing immediate feedback on the validity of the entered data.
- Showing or hiding additional information: You can use the onFocus event to display additional instructions or hints when the user focuses on an input, and the onBlur event to hide them when the input loses focus.
- Clearing placeholder text: You can use the onFocus event to clear the placeholder text when the user starts interacting with an input, improving the user experience.
- Triggering animations or UI changes: You can use these events to trigger animations or update the UI based on the focus state of the input. It's important to note that the onBlur and onFocus events are not specific to React; they are standard JavaScript events that can be used in any web development context. However, in React, you can easily attach these event handlers to form inputs using the onBlur and onFocus props, respectively.

<input
type="text"
onFocus={(event) => {
console.log('Input received focus');
// Perform actions or update the UI
}}
/>
The main difference between onBlur and onFocus events is the timing of when they are triggered:
- onBlur is triggered when the input loses focus, indicating that the user has finished interacting with the input.
- onFocus is triggered when the input receives focus, indicating that the user is about to start interacting with the input. These events can be useful in various scenarios, such as:
- Performing form validations: You can use the onBlur event to validate the input value when the user moves away from the input, providing immediate feedback on the validity of the entered data.
- Showing or hiding additional information: You can use the onFocus event to display additional instructions or hints when the user focuses on an input, and the onBlur event to hide them when the input loses focus.
- Clearing placeholder text: You can use the onFocus event to clear the placeholder text when the user starts interacting with an input, improving the user experience.
- Triggering animations or UI changes: You can use these events to trigger animations or update the UI based on the focus state of the input. It's important to note that the onBlur and onFocus events are not specific to React; they are standard JavaScript events that can be used in any web development context. However, in React, you can easily attach these event handlers to form inputs using the onBlur and onFocus props, respectively.
React Interview Questions: Lists and Keys
44. What is the purpose of keys in React lists?
Answer: In React, when rendering a list of elements, it's important to provide a unique "key" prop for each element in the list. The purpose of keys is to help React efficiently update and reorder the list elements when there are changes to the underlying data. Here are the main reasons why keys are crucial in React lists:
- Efficient Updates:
- When a list is updated (e.g., elements are added, removed, or reordered), React uses the keys to determine which elements have changed.
- By comparing the keys of the previous and new lists, React can efficiently update only the necessary elements instead of re-rendering the entire list.
- This optimization improves performance, especially for large lists, by minimizing unnecessary DOM manipulations.
- Preserving State:
- Keys help React preserve the state of individual list elements across re-renders.
- When an element's key remains the same between renders, React knows that it represents the same component instance and can maintain its state.
- Without proper keys, React may incorrectly update or lose the state of elements when the list changes.
- Avoiding Unexpected Behavior:
- Using proper keys ensures that React correctly matches the elements between the previous and new lists.
- If keys are not provided or are not unique, React may make incorrect assumptions about which elements have changed, leading to unexpected behavior or bugs. When assigning keys to list elements, it's recommended to use a stable and unique identifier for each item in the list. Here are some guidelines for choosing keys:
- Use a unique and stable identifier from your data, such as an ID or a unique combination of properties.
- Avoid using the array index as the key, especially if the order of items may change or if items can be added or removed dynamically. Using the index as the key can lead to incorrect behavior and performance issues.
- If you don't have a stable identifier, you can generate a unique key using libraries like UUID or by combining multiple properties to create a unique string. Here's an example of rendering a list with proper keys:
- What is the difference between using index as a key and using a unique identifier? Answer: When rendering a list of elements in React, it's generally recommended to use a unique identifier as the key rather than the index of the element in the array. Here are the differences between using the index as a key and using a unique identifier:
- Stability:
- Using a unique identifier as the key ensures that the key remains stable even if the order of elements in the list changes.
- If the index is used as the key and the order of elements changes (e.g., due to sorting, filtering, or adding/removing elements), React may incorrectly reuse or reorder the elements, leading to unexpected behavior.
- Consistency:
- A unique identifier guarantees that the key remains consistent across different renders of the list.
- If the index is used as the key and elements are added or removed from the list, the keys of the remaining elements will change, causing React to unnecessarily re-render those elements.
- Performance:
- Using a unique identifier allows React to efficiently update and reorder the list elements when there are changes to the underlying data.
- If the index is used as the key and elements are added or removed in the middle of the list, React may unnecessarily re-render a large portion of the list, impacting performance.
- Predictability:
- With a unique identifier, the behavior of the list is more predictable, as each element is uniquely identified and tracked by React.
- Using the index as the key can lead to unpredictable behavior, especially when elements are dynamically added, removed, or reordered. Here's an example that demonstrates the difference:
- Stability:
45. How do you dynamically generate a list in React?
Answer: To dynamically generate a list in React, you can use the map() function to iterate over an array of data and render a component or JSX element for each item in the array. Here's an example of how to dynamically generate a list in React:In this example:

In this example:
- We have an array of data called data, which contains objects representing list items. Each object has an id and a name property.
- Inside the MyList component, we use the map() function to iterate over the data array.
- For each item in the array, we return an <li> element that displays the name property of the item.
- We assign a unique key prop to each <li> element using the id property of the item. This helps React efficiently update and reorder the list elements.
- The resulting array of <li> elements is rendered inside a <ul> element, creating an unordered list. When the MyList component is rendered, it will dynamically generate a list of <li> elements based on the data array. Each item in the array will have its own <li> element with a unique key and the corresponding name displayed. You can modify the structure and content of the list items based on your specific requirements. For example, you can include additional properties from the data objects or apply conditional rendering based on certain conditions. Here's an example that demonstrates more complex list rendering:
JSX

import React from 'react';
const data = [
{ id: 1, name: 'Item 1', description: 'Description 1', highlighted: true },
{ id: 2, name: 'Item 2', description: 'Description 2', highlighted: false },
{ id: 3, name: 'Item 3', description: 'Description 3', highlighted: true },
];
function MyList() {
return (
<ul>
{data.map((item) => (
<li
key={item.id}
className={item.highlighted ? 'highlighted' : ''}
>
<h3>{item.name}</h3>
<p>{item.description}</p>
</li>
))}
</ul>
);
}
export default MyList;
In the first example, the index is used as the key. If the order of the todos array changes or if elements are added or removed, React may incorrectly update or reorder the list elements, leading to unexpected behavior. In the second example, a unique identifier (todo.id) is used as the key. This ensures that each element is uniquely identified, and React can efficiently update and reorder the list elements based on their unique keys. It's important to note that using the index as the key is not always problematic. If the list is static and the order of elements is guaranteed to remain the same, using the index as the key may be acceptable. However, in most cases, it's safer and more reliable to use a unique identifier as the key to ensure correct behavior and optimal performance.
46. What is the purpose of the key prop when rendering lists in React?
Answer: The key prop is a special attribute that you should include when rendering lists of elements in React. It helps React identify which items in a list have changed, been added, or been removed, allowing for efficient updates and reordering of the list elements. Here are the main purposes of the key prop when rendering lists:
- Efficient Updates:
- When a list undergoes changes (e.g., items are added, removed, or reordered), React uses the key prop to determine which items have been modified.
- By comparing the key values of the previous and new lists, React can efficiently update only the necessary elements instead of re-rendering the entire list.
- This optimization improves performance, especially for large lists, by minimizing unnecessary DOM manipulations.
- Preserving State:
- The key prop helps React preserve the state of individual list items across re-renders.
- When an item's key remains the same between renders, React knows that it represents the same component instance and can maintain its state.
- Without proper key values, React may incorrectly update or lose the state of list items when the list changes.
- Avoiding Bugs and Unexpected Behavior:
- Using appropriate key values ensures that React correctly matches the list items between the previous and new lists.
- If key values are not provided or are not unique, React may make incorrect assumptions about which items have changed, leading to bugs or unexpected behavior. When assigning key values to list items, it's important to follow these guidelines:
- Use a unique and stable identifier for each item in the list, such as an ID from your data or a unique combination of properties.
- Avoid using the array index as the key, especially if the order of items can change or if items can be added or removed dynamically. Using the index as the key can lead to incorrect behavior and performance issues.
- If you don't have a stable identifier, you can generate a unique key using libraries like UUID or by combining multiple properties to create a unique string. Here's an example that demonstrates the usage of the key prop when rendering a list:
JSX

import React from 'react';
const data = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' },
];
function MyList() {
return (
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
export default MyList;
In this example, each item in the data array has a unique id property. When rendering the list, we assign the id as the key value for each <li> element using the key prop. By providing a unique and stable key for each list item, React can efficiently update and reorder the list elements when necessary, preserve the state of individual items, and avoid unexpected behavior. Remember, the key prop is not accessible within the component itself. It is only used by React internally to optimize the rendering and reconciliation process of lists.
47. What are the benefits of using React fragments when rendering lists?
Answer: React fragments provide a way to group multiple elements without adding an extra DOM node. When rendering lists, using fragments can offer several benefits:
- Cleaner and More Concise Code:
- With fragments, you can group list items without the need for a wrapping element like a <div> or <ul>.
- This results in cleaner and more concise code, as you can avoid unnecessary markup and focus on the list items themselves.
- Fragments allow you to return multiple elements from a component without a parent element.
- Improved Performance:
- Using fragments can slightly improve performance compared to using a wrapping element.
- When you wrap list items with an additional element, it creates an extra DOM node for each list, which can impact performance, especially for large lists.
- Fragments, on the other hand, do not create any additional DOM nodes, reducing the overall memory footprint and rendering overhead.
- Flexibility in Component Composition:
- Fragments provide flexibility when composing components that render lists.
- You can easily combine and nest fragments within other components without introducing unnecessary levels of DOM hierarchy.
- This can make your component structure more modular and easier to manage.
- Semantic Markup:
- Using fragments allows you to maintain a semantically correct HTML structure.
- In some cases, wrapping list items with an extra element may not be semantically accurate or may conflict with the desired HTML structure.
- Fragments enable you to render list items directly without compromising the semantic meaning of your markup. Here's an example that demonstrates the use of React fragments when rendering a list:
React Interview Questions: Forms and Controlled Components
48. What are controlled components in React?
Answer: In React, controlled components are form elements (such as <input>, <textarea>, or <select>) whose values are controlled by React state. Instead of relying on the browser's internal state management, controlled components allow React to have full control over the form data. Here are the key characteristics of controlled components:
- Value Binding:
- The value of the form element is bound to a state variable in the React component.
- Whenever the state variable changes, the value of the form element is updated accordingly.
- This ensures that the form element always reflects the current state of the component.
- Change Handling:
- The component defines an event handler function (e.g., onChange) that is triggered whenever the user interacts with the form element.
- The event handler function updates the corresponding state variable based on the user's input.
- By updating the state, the component re-renders, and the form element's value is updated to reflect the new state.
- Single Source of Truth:
- With controlled components, the React component becomes the single source of truth for the form data.
- The state of the form is managed within the component, and any changes to the form data are handled through state updates.
- This centralized state management makes it easier to perform form validation, data manipulation, and submission. Here's an example of a controlled component for an input field:
JSX

import React, { useState } from 'react';
function MyForm() {
const [name, setName] = useState('');
const handleChange = (event) => {
setName(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
console.log('Submitted name:', name);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={name} onChange={handleChange} />
</label>
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
In this example:
- The name state variable is initialized using the useState hook.
- The value prop of the input field is bound to the name state variable.
- The onChange event handler is defined to update the name state variable whenever the user types in the input field.
- The handleSubmit function is called when the form is submitted, preventing the default form submission behavior and logging the current value of name. By using controlled components, you have complete control over the form data and can easily perform validation, manipulation, and submission tasks within the React component. It provides a more predictable and manageable way to handle form inputs compared to uncontrolled components, where the form data is managed by the browser's internal state. Controlled components are widely used in React applications for managing form inputs, as they provide a cleaner and more declarative approach to handling form data.
49. What are the advantages of using controlled components?
Answer: Using controlled components in React offers several advantages over uncontrolled components. Here are some key advantages of using controlled components:
- Single Source of Truth:
- With controlled components, the React component becomes the single source of truth for the form data.
- The form data is managed within the component's state, and any changes to the form are handled through state updates.
- This centralized state management makes it easier to keep the form data in sync and maintain consistency throughout the component.
- Instant Input Validation:
- Controlled components allow you to perform instant input validation as the user types.
- Since the form data is controlled by the component's state, you can easily validate the input values on every change event.
- You can provide real-time feedback to the user about the validity of their input, enhancing the user experience and preventing invalid data submission.
- Conditional Rendering:
- With controlled components, you have full control over the rendering of form elements based on the component's state.
- You can conditionally render form elements, disable or enable inputs, or show/hide certain sections of the form based on the current state.
- This flexibility allows you to create dynamic and interactive forms that respond to user input and application logic.
- Data Manipulation:
- Controlled components make it easy to manipulate and transform the form data before submitting it to a server or performing further actions.
- Since the form data is stored in the component's state, you can apply any necessary data transformations, such as formatting, normalization, or calculations, before sending the data to the server.
- This ensures that the data is in the desired format and meets the application's requirements.
- Simplified Form Submission:
- With controlled components, handling form submission becomes straightforward.
- When the form is submitted, you have access to the latest form data in the component's state.
- You can easily retrieve the form data, perform any final validations, and send it to the server or perform other actions without relying on DOM manipulation or accessing form element values directly.
- Testability:
- Controlled components make it easier to write unit tests for form-related functionality.
- Since the form data is managed by the component's state, you can simulate user interactions and assert the expected behavior of the form by manipulating the state directly in your tests.
- This improves the testability of your React components and ensures that the form logic works as expected.
- Reusability and Composability:
- Controlled components promote reusability and composability in React applications.
- By encapsulating the form logic and state within a controlled component, you can easily reuse that component across different parts of your application.
- Controlled components can be composed together to create more complex forms, allowing for modular and maintainable code. Overall, controlled components provide a more declarative, predictable, and maintainable approach to handling form data in React applications. They offer better control over form behavior, validation, and submission, making it easier to create robust and interactive forms. However, it's important to note that controlled components may require more code and state management compared to uncontrolled components. The choice between controlled and uncontrolled components depends on the specific requirements and complexity of your form.
50. How do you handle form submission in React?
Answer: Handling form submission in React typically involves using controlled components and defining a submit handler function. Here's a step-by-step guide on how to handle form submission in React:
- Create a controlled component for the form:
- Define state variables for each form input using the useState hook.
- Bind the value of each form input to its corresponding state variable.
- Define event handlers (e.g., onChange) to update the state variables when the user interacts with the form inputs.
- Define a submit handler function:
- Create a function that will be called when the form is submitted.
- This function will receive the form event as a parameter.
- Attach the submit handler to the form's onSubmit event:
- Add an onSubmit event handler to the <form> element.
- Pass the submit handler function as the value of onSubmit.
- Prevent the default form submission behavior:
- Inside the submit handler function, call event.preventDefault() to prevent the default form submission behavior, which would normally refresh the page.
- Perform form validation (optional):
- Before processing the form data, you can perform any necessary form validation.
- Check if the form inputs meet the required criteria and display appropriate error messages if needed.
- Process the form data:
- Access the form data from the component's state variables.
- Perform any required data transformations or calculations.
- Send the form data to a server or perform any other necessary actions.
- Reset the form (optional):
- After successfully processing the form data, you may want to reset the form to its initial state.
- Clear the form inputs by resetting the state variables to their initial values.
Here's an example of handling form submission in a React component:

import React, { useState } from 'react';
function MyForm() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
// Perform form validation
if (!name || !email) {
alert('Please fill in all fields');
return;
}
// Process form data
console.log('Submitted:', { name, email });
// Reset form
setName('');
setEmail('');
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Name:</label>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
<div>
<label>Email:</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
</div>
<button type="submit">Submit</button>
</form>
);
}
export default MyForm;
In this example:
- The component uses the useState hook to manage the state variables name and email for the form inputs.
- The handleSubmit function is defined to handle the form submission. It prevents the default form submission behavior, performs form validation, processes the form data (in this case, logging it to the console), and resets the form.
- The onSubmit event handler is attached to the <form> element, invoking the handleSubmit function when the form is submitted.
- The form inputs are bound to their respective state variables using the value and onChange props, ensuring that the component remains the single source of truth for the form data. By following this approach, you can handle form submission in a controlled and predictable manner, allowing you to easily validate, process, and reset the form data as needed.
51. What is the difference between controlled and uncontrolled components?
Answer: The main difference between controlled and uncontrolled components in React lies in how they handle form data and user input. Here's a comparison of controlled and uncontrolled components: Controlled Components:
- In controlled components, the form data is managed by the React component's state.
- The value of each form input is bound to a state variable, and any changes to the input are handled through state updates.
- The component becomes the single source of truth for the form data.
- Whenever the user interacts with a form input, an event handler is triggered to update the corresponding state variable.
- The component re-renders whenever the state is updated, keeping the form inputs in sync with the component's state.
- Controlled components provide more control and predictability over form behavior and data management.
- They allow for instant input validation, conditional rendering, and easy data manipulation before submission. Uncontrolled Components:
- In uncontrolled components, the form data is managed by the DOM itself, not by the React component's state.
- The form inputs are not bound to any state variables, and their values are accessed directly from the DOM using refs.
- The component does not have complete control over the form data, and the browser handles the form submission natively.
- Uncontrolled components rely on the DOM to store and manage the form data.
- To access the form data, you need to use refs to get the current values of the form inputs when needed (e.g., on form submission).
- Uncontrolled components are simpler to implement for basic forms that don't require real-time validation or complex data management.
Here's an example that demonstrates the difference between controlled and uncontrolled components: Controlled Component:
import React, { useState } from 'react';
function ControlledForm() {
const [name, setName] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
console.log('Submitted name:', name);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</label>
<button type="submit">Submit</button>
</form>
);
}
Uncontrolled Component:
React, { useRef } from 'react';
function UncontrolledForm() { const nameInput = useRef(null);
const handleSubmit = (event) => { event.preventDefault(); console.log('Submitted name:', nameInput.current.value); };
return ( <form onSubmit={handleSubmit}> <label> Name: <input type="text" ref={nameInput} /> </label> <button type="submit">Submit</button> </form> ); }
In the controlled component example, the `name` state variable is bound to the input's value, and the `onChange` event handler updates the state whenever the input value changes. The form submission is handled by the `handleSubmit` function, which accesses the form data from the component's state.
In the uncontrolled component example, the input's value is not bound to any state variable. Instead, a ref (`nameInput`) is used to access the input's value directly from the DOM when the form is submitted. The `handleSubmit` function retrieves the input's value using `nameInput.current.value`.
The choice between controlled and uncontrolled components depends on the specific requirements of your form and the level of control and validation you need. Controlled components provide more fine-grained control and real-time validation, while uncontrolled components are simpler to set up for basic forms that don't require extensive data management.
Authors
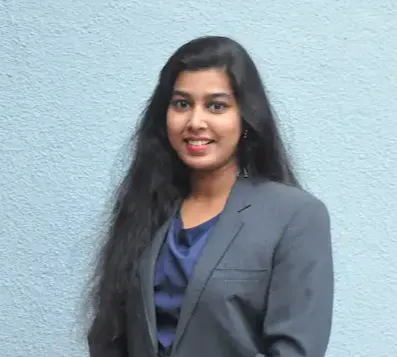
Soujanya Varada
As a technical content writer and social media strategist, Soujanya develops and manages strategies at HireQuotient. With strong technical background and years of experience in content management, she looks for opportunities to flourish in the digital space. Soujanya is also a dance fanatic and believes in spreading light!
Hire the best without stress
Ask us how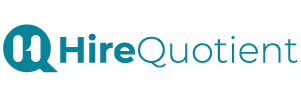
Never Miss The Updates
We cover all recruitment, talent analytics, L&D, DEI, pre-employment, candidate screening, and hiring tools. Join our force & subscribe now!
Stay On Top Of Everything In HR