110+ C++ interview questions (Updated for 2024)
Published on May 2nd, 2024
Introduction to C++ and Its Importance in Interviews
C++ remains one of the most potent programming languages, combining the capability to develop high-performance software with the flexibility of object-oriented programming. As you prepare for your next job opportunity, understanding C++ can set you apart in tech interviews, especially for roles in systems programming, game development, and even software engineering where performance and efficiency are paramount.
This blog aims to prepare you thoroughly for C++ interviews by presenting over 110 targeted questions and answers, making sure you're ready to tackle the complexities of C++ with confidence.
C++ Fundamentals
Syntax and Operators
C++ has a rich set of operators and a syntax that supports a variety of programming styles. Key questions often explore the use of operators and the basic structure of C++ programs.
Question 1: What is operator overloading and can you give an example?
Answer: Operator overloading allows operators to have user-defined meanings on user-defined types (classes). For example, you can overload the + operator to add two objects of a class that represents complex numbers.
Control Structures
Understanding control structures is crucial, as they form the backbone of directing the flow of a C++ program.
Question 2: Explain the difference between for and while loops in C++.
Answer: For loops are typically used when the number of iterations is known before the loop starts, whereas while loops are used when the iterations depend on a condition that is checked before each iteration.
Functions
Functions are building blocks in C++, and questions often probe your understanding of their declarations, types, and special cases.
Question 3: What is the difference between a member function and a static function?
Answer: A member function operates on specific instances of a class and has access to its instance variables (this pointer), whereas a static function does not operate on instances and cannot access instance variables directly.
Object-Oriented Programming in C++
Classes and Objects
Classes and objects are fundamental in C++, encapsulating data and functions into a single entity.
Question 4: What are constructors and destructors?
Answer: Constructors are special functions called when a new object is created to initialize it. Destructors are special functions called when an object is destroyed to free resources it used.
Inheritance
Inheritance allows the creation of a new class based on an existing class, an essential feature of C++.
Question 5: What is multiple inheritance and what are its pitfalls?
Answer: Multiple inheritance allows a class to inherit from more than one base class. This can lead to ambiguities and complexities such as the Diamond Problem, where an attribute or method can be inherited from multiple ancestor paths.
Polymorphism
Polymorphism is a cornerstone of C++, enabling interface segregation and implementation flexibility.
Question 6: What is a virtual function and why is it used in C++?
Answer: A virtual function is a member function that you expect to be overridden in derived classes. When you use a reference or a pointer to a base class to call a virtual function, C++ determines which function version to invoke at runtime, enabling dynamic dispatch.
Advanced Topics in C++
Templates and STL
Templates support generic programming, and the Standard Template Library (STL) is a collection of ready-to-use C++ templates for various data structures and algorithms.
Question 7: Explain the concept of template specialization in C++.
Answer: Template specialization allows a function or class to be written to handle a specific type in a way that is different from the general template code, improving efficiency or tailoring functionality.
Exception Handling
Robust C++ applications require effective error handling, often explored in interviews.
Question 8: How does C++ handle exceptions?
Answer: C++ uses try, catch, and throw keywords to handle exceptions. Code that might throw an exception is put inside a try block, and the exception is caught with catch blocks to handle the error.
Memory Management
C++ provides low-level control over system resources, so understanding memory management is critical.
Question 9: What is a memory leak and how can it be prevented?
Answer: A memory leak occurs when dynamically allocated memory is not freed after use, leading to resource exhaustion. It can be prevented by ensuring every new operation is matched with a delete.
This segment-wise approach to crafting the blog will help in thoroughly covering each aspect of C++, ensuring the content is rich and comprehensive, surpassing existing materials in depth and clarity.
Data Structures Using C++
Understanding how data structures are implemented in C++ is crucial for both practical programming and interview preparation. This section will cover common data structures, supplemented with specific C++ interview questions to demonstrate your mastery.
Arrays
Arrays are a fundamental data structure in C++, often used to store collections of elements in a contiguous memory allocation.
Question 10: How do you initialize an array in C++?
Answer: An array in C++ can be initialized using the syntax int arr[5] = {1, 2, 3, 4, 5}; where 5 is the number of elements, and the values inside the braces are the initial values.
Linked Lists
Linked lists are dynamic data structures that consist of nodes linked together by pointers, providing flexibility in memory usage.
Question 11: What is a doubly linked list and how does it differ from a singly linked list?
Answer: A doubly linked list allows navigation both forward and backward as each node contains two pointers: one pointing to the next node and one to the previous node. In contrast, a singly linked list only allows forward traversal as each node points only to the next node.
Stacks and Queues
Stacks and queues are linear data structures that differ in their element access methods.
Question 12: Explain the difference between a stack and a queue in C++.
Answer: A stack is a LIFO (Last In, First Out) data structure where the last element added is the first to be removed. A queue is a FIFO (First In, First Out) data structure where the first element added is the first to be removed.
Practical Coding Questions
This segment will include typical C++ coding questions that are frequently asked in interviews, testing your problem-solving skills and understanding of C++ specifics.
String Manipulation
Manipulating strings is a common task in C++ programming and interviews.
Question 13: How can you reverse a string in C++?
Answer: You can reverse a string in C++ using the std::reverse function from the <algorithm> header. For example, std::reverse(str.begin(), str.end()); will reverse the string str.
Searching Algorithms
Efficient searching is critical in software development, making it a popular topic in interviews.
Question 14: What is binary search and how can you implement it in C++?
Answer: Binary search is an efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing in half the portion of the list that could contain the item, until the possible locations are reduced to just one. In C++, it can be implemented using recursion or iteratively with a loop.
C++11 and Beyond
Understanding the newer standards of C++ is essential, as they introduce many features that simplify code and enhance performance.
Modern Features
C++11 and later versions introduced several new features that modernized the language significantly.
Question 15: What are lambda expressions in C++ and how are they used?
Answer: Lambda expressions provide a concise way to write inline functions. They are often used with algorithms to apply operations on collections. For example, std::for_each(vec.begin(), vec.end(), [](int x){ std::cout << x << " "; }); prints each element of the vector vec.
Smart Pointers
Smart pointers are objects that ensure memory leaks are avoided by making automatic deallocation of memory.
Question 16: What is a unique pointer and how does it differ from a shared pointer?
Answer: A unique pointer (std::unique_ptr) owns the object it points to and ensures there is only one owner of the underlying pointer, which is automatically deleted when the unique pointer goes out of scope. A shared pointer (std::shared_ptr) allows multiple pointers to reference the same object; the object is destroyed when the last shared pointer to it is destroyed.
Interview Tips and Strategies
Concluding with strategic advice on approaching C++ interviews, this section will help candidates understand the expectations and how to effectively demonstrate their skills.
Question 17: What is the best way to prepare for a C++ coding interview?
Answer: The best way to prepare is to practice coding regularly, understand the core concepts deeply, and stay updated with the latest C++ standards. Also, practicing problems from previous interviews and reading up on common pitfalls in C++ programming can be highly beneficial.
Extended Data Structures Using C++
Trees
Question 18: What is a binary search tree (BST)?
Answer: A BST is a binary tree where each node has a comparable key (and an associated value) and satisfies the restriction that the key in any node is larger than the keys in all nodes in its left subtree and smaller than the keys in all nodes in its right subtree.
Question 19: How do you perform an inorder traversal in a binary search tree?
Answer: An inorder traversal in a BST involves visiting the left subtree, the node itself, and then the right subtree. This traversal yields nodes in ascending order.
Graphs
Question 20: What is a graph in C++ and how can it be represented?
Answer: A graph is a collection of nodes (or vertices) and edges connecting pairs of nodes. In C++, graphs can be represented using adjacency lists (a vector of lists for dynamic sizes) or adjacency matrices (a 2D array).
Extended Practical Coding Questions
Algorithm Implementation
Question 21: How would you implement a quicksort algorithm in C++?
Answer: Quicksort is a divide-and-conquer algorithm that divides a list into two smaller sub-lists, sorts them, and then merges them. A pivot element is chosen from the array elements and partitions the other elements into those less than the pivot and those greater.
Problem Solving
Question 22: Write a C++ program to check if a string is a palindrome.
Answer: A string is a palindrome if it reads the same forward and backward. You can check this by comparing characters from the beginning and end of the string, moving toward the center.
Extended C++11 and Beyond
Concurrency
Question 23: What are std::async and std::future in C++?
Answer: std::async launches a potentially asynchronous task. std::future is used to retrieve the result from asynchronous operations launched with std::async.
Lambda Expressions and Their Use Cases
Question 24: Provide an example of using a lambda expression to sort a vector of integers in descending order.
Answer: You can use a lambda expression with the std::sort function to specify a custom comparator. For instance: std::sort(v.begin(), v.end(), [](int a, int b) { return a > b; }); sorts the vector v in descending order.
Range-based Loops
Question 25: What are range-based loops in C++ and how do they simplify code?
Answer: Range-based loops allow you to iterate over elements of a container or range directly, making the code cleaner and less error-prone. For example, for(auto x : vec) { cout << x << " "; } prints each element in the vector vec.
Advanced Features
Question 26: How do you use the 'auto' keyword in C++11?
Answer: The auto keyword instructs the compiler to automatically deduce the type of the variable from its initializer expression.
Additional Interview Tips and Strategies
Behavioral Questions
Question 27: How do you stay updated with the latest C++ developments?
Answer: Highlight regular reading of industry publications, participation in developer communities, and engagement with ongoing projects using modern C++.
Technical Problem-Solving
Question 28: Describe your approach to debugging a complex C++ application.
Answer: Discuss systematic debugging strategies such as using debuggers, analyzing stack traces, and simplifying code to isolate the problem area.
Advanced Memory Management
Smart Pointers Detailed
Question 29: How does std::shared_ptr manage memory and what are its advantages?
Answer: std::shared_ptr uses reference counting to ensure that multiple pointers can share ownership of the same object safely. The object is automatically destroyed when the last shared_ptr pointing to it is destroyed or reset, helping prevent memory leaks.
Move Semantics
Question 30: Explain move semantics in C++ and give an example where it is useful.
Answer: Move semantics allow the resources of a rvalue object to be moved rather than copied. This is useful in improving performance by saving resource allocation and deallocation costs, for example, when returning large objects from functions or moving data into a container.
Additional Interview Tips and Strategies (continued)
Communication Skills
Question 31: How important are communication skills in software development interviews, specifically for C++ roles?
Answer: Communication is crucial as it demonstrates your ability to explain complex concepts clearly and work effectively in teams. For C++ roles, this might involve discussing the design decisions behind a piece of code or explaining how your code works to non-technical stakeholders.
Problem-Solving Approach
Question 32: What systematic approach would you recommend for solving algorithmic problems in C++ during interviews?
Answer: A systematic approach involves understanding the problem thoroughly, breaking it down into manageable parts, devising a plan or algorithm, coding the solution in C++, and finally testing and debugging. Illustrate your thought process clearly and comment on your code as you write it.
Further Resources for Deep Diving Into C++ Concepts
As you prepare for your C++ interview, consider exploring advanced resources that can provide deeper insights into the language's capabilities and best practices:
- Online Documentation: Websites like cppreference.com offer comprehensive details on every aspect of C++, including the latest standards.
- Community Insights: Platforms like GitHub or C++ Weekly on YouTube provide community-driven insights and discussions on modern C++ practices and pitfalls.
- Advanced Books: "Advanced C++ Programming Cookbook" by Dr. Rian Quinn provides a practical approach to modern C++ techniques and scenarios.
Multi-Threading and Concurrency in C++
Understanding multi-threading and concurrency is crucial for developing efficient C++ applications, especially in high-performance computing environments.
Thread Management
Question 33: How do you create threads in C++11?
Answer: In C++11, threads can be created using the std::thread class. For example, std::thread t(func); creates a new thread where func is the function to be executed by the thread.
Synchronization Mechanisms
Question 34: What is a mutex and how is it used in C++?
Answer: A mutex is a synchronization primitive used to protect shared data from being simultaneously accessed by multiple threads. std::mutex provides exclusive, non-recursive ownership semantics.
Exception Safety and Error Handling
Handling exceptions correctly is vital in C++, ensuring that resources are properly managed and applications remain stable.
Exception Safety Guarantees
Question 35: What are the different levels of exception safety in C++?
Answer: C++ defines several levels of exception safety, including nothrow guarantee, strong guarantee, and basic guarantee, each providing different levels of safety in the face of exceptions.
Handling Exceptions in Constructors and Destructors
Question 36: How should exceptions be handled in constructors and destructors?
Answer: In constructors, exceptions should be used to signal the failure of object creation. In destructors, exceptions should be avoided because destructors are called during stack unwinding when another exception is already active.
Best Practices in C++ Programming
C++ is a powerful language but requires attention to best practices to avoid common pitfalls.
Code Optimization Techniques
Question 37: What are some effective ways to optimize C++ code?
Answer: Effective optimization techniques include minimizing copying of objects, using reserve() on vectors to avoid unnecessary reallocations, and preferring algorithms from the STL that operate directly on containers.
Safe and Efficient Memory Use
Question 38: What strategies can be employed for safe and efficient memory management in C++?
Answer: Strategies include using smart pointers like std::unique_ptr and std::shared_ptr to manage lifecycles of objects dynamically, and using object pools for frequently created and destroyed objects.
Keeping Up with C++ Evolution
Staying updated with the latest developments in C++ is essential for maintaining expertise in the language.
Following C++ Standards
Question 39: How can a developer keep up-to-date with the evolving C++ standards?
Answer: Developers can keep up-to-date by following the ISO C++ committee's publications, attending C++ conferences, and participating in community discussions on forums like Stack Overflow.
New Library Features
Question 40: What are some of the notable new features in the latest C++ standard libraries?
Answer: Recent C++ standards have introduced several new library features, such as filesystem operations, more algorithms that operate on ranges, and improvements to the concurrency libraries.
Advanced STL Usage and Techniques
The Standard Template Library (STL) is a powerful feature of C++, providing a set of ready-made classes and functions for common data structures and algorithms. Advanced knowledge of STL can significantly enhance problem-solving efficiency in interviews.
Complex Data Structures with STL
Question 41: How can you use STL to implement a graph?
Answer: STL provides several ways to implement a graph, most commonly using an adjacency list, which can be represented by a std::vector of std::list pairs where each pair contains a node and its connections.
STL Algorithms for Problem Solving
Question 42: What are some advanced STL algorithms that are frequently useful in C++ coding interviews?
Answer: Algorithms like std::next_permutation, std::partial_sort, and std::nth_element are powerful for solving complex problems efficiently. They allow operations on data without manually implementing sorting and searching functionalities.
Effective Use of Templates in C++
Templates in C++ provide a way to write code that handles any data type and can be a significant advantage in creating flexible and reusable code.
Template Metaprogramming
Question 43: What is template metaprogramming and how can it be used in C++?
Answer: Template metaprogramming involves writing templates that execute at compile time, allowing programmers to perform optimizations and computations during compilation. It's commonly used for creating highly efficient and optimized code for specific tasks.
Practical Applications of Templates
Question 44: Provide an example of a practical application of templates in C++.
Answer: Templates are extensively used in creating generic data structures like linked lists, stacks, queues, and trees, which can operate with any data type, enhancing code reusability and maintainability.
Design Patterns in C++
Understanding design patterns is crucial for writing clean, efficient, and scalable C++ code, especially in complex software development environments.
Common Design Patterns
Question 45: What are some common design patterns used in C++ and their applications?
Answer: Singleton, Factory, Observer, and Decorator are some of the most common design patterns. For example, the Singleton pattern ensures a class has only one instance and provides a global point of access to it.
Design Patterns for Concurrency
Question 46: How can design patterns improve concurrency in C++ applications?
Answer: Design patterns like Singleton and Observer can be adapted with thread-safe mechanisms to manage data consistency and synchronization across multiple threads in a concurrent application.
Performance Optimization in C++
Performance optimization is a critical aspect, especially in systems where efficiency and speed are crucial.
Optimization Techniques
Question 47: What are some key optimization techniques for C++ applications?
Answer: Techniques include avoiding unnecessary copies of objects, using move semantics effectively, optimizing memory allocations, and utilizing compiler optimizations like inline functions.
Profiling and Debugging Tools
Question 48: What tools are recommended for profiling and debugging C++ applications?
Answer: Tools such as Valgrind for memory profiling, GDB for debugging, and Visual Studio's Performance Profiler are crucial for identifying performance bottlenecks and memory leaks.
Advanced Error Handling and Resource Management
In C++, managing errors and resources properly is critical to ensuring robust, stable applications. This segment explores sophisticated error handling techniques and resource management strategies.
Exception Handling Best Practices
Question 49: What are some best practices for exception handling in C++?
Answer: Best practices include using exceptions for exceptional conditions only, throwing objects of types derived from std::exception, and catching exceptions by reference.
Resource Management Techniques
Question 50: Explain the RAII (Resource Acquisition Is Initialization) principle in C++.
Answer: RAII is a programming idiom used in C++ to manage resource allocation and deallocation. It ensures that resources are acquired during object construction and released during object destruction, which helps in preventing resource leaks.
Advanced Concepts in Object-Oriented Programming
Deepening your understanding of object-oriented programming in C++ can significantly improve your software design and problem-solving skills.
Virtual Destructors
Question 51: Why are virtual destructors necessary in C++?
Answer: Virtual destructors are crucial when you might delete an instance of a derived class through a pointer of base class type. Without a virtual destructor, the destructor of the derived class does not get called, leading to resource leakage.
Operator Overloading
Question 52: How can operator overloading improve usability of custom data types in C++?
Answer: Operator overloading allows for intuitive usage of custom types. For example, overloading arithmetic operators for a complex number class lets users manipulate complex numbers with standard operators (+, -, *, /).
C++ and System Level Programming
C++ is often used for system-level programming due to its low-level programming capabilities. This section covers essential aspects of using C++ in such contexts.
Memory Management at System Level
Question 53: What are some tips for managing memory efficiently in system-level C++ programming?
Answer: Tips include understanding low-level memory layout, using memory pools for efficient allocation and deallocation, and minimizing memory fragmentation with appropriate allocation strategies.
Interfacing with Hardware
Question 54: How can C++ be used to interface directly with hardware?
Answer: C++ can interact with hardware by accessing memory-mapped peripheral registers, using embedded system APIs, or through direct system calls, which allows manipulation of hardware components at a low level.
Modern C++ Features and Best Practices
Staying current with modern C++ features and best practices is essential for writing clean, effective, and maintainable code.
C++20 Features
Question 55: What are some notable features introduced in C++20?
Answer: C++20 introduces features like concepts for type-checking at compile time, coroutines for simplified asynchronous programming, and ranges for more powerful and flexible container manipulation.
Best Practices for Modern C++
Question 56: What are some best practices for using modern C++ effectively?
Answer: Best practices include preferring STL algorithms over loops, utilizing smart pointers instead of raw pointers for memory management, and embracing lambda expressions for inline function definitions.
Multithreading and Advanced Concurrency
Multithreading and concurrency are essential topics in C++ for developing performance-oriented applications. Here, we delve into complex issues and solutions.
Handling Race Conditions
Question 57: What is a race condition and how can it be prevented in C++?
Answer: A race condition occurs when two or more threads can access shared data and they try to change it at the same time. It can be prevented using mutexes, locks, or other synchronization techniques to ensure that only one thread can access the data at a time.
Utilizing Concurrency Libraries
Question 58: What are some key features of the C++ Concurrency library?
Answer: The C++ Concurrency library includes features such as thread pools, async operations, futures, and promises, which facilitate easier and more efficient multithreaded programming.
Advanced Input/Output in C++
Handling input/output operations efficiently is crucial for the performance of C++ applications, especially in file and network operations.
Stream Manipulation and Optimization
Question 59: How can stream manipulators be used to format C++ output precisely?
Answer: Stream manipulators like std::setw, std::setprecision, and std::fixed are used to control the format of the output stream, such as setting the width and precision of floating point numbers.
Asynchronous I/O Operations
Question 60: What are the benefits of asynchronous I/O operations in C++?
Answer: Asynchronous I/O operations improve application efficiency by not blocking the execution while the I/O operation completes, thus allowing a program to continue processing other tasks.
Advanced Template Programming
Templates are a powerful feature in C++, enabling generic and metaprogramming techniques.
Template Specialization
Question 61: What is template specialization in C++, and why is it used?
Answer: Template specialization allows for the customization of template code for a particular data type, providing optimized or specific functionality for that type.
Variadic Templates
Question 62: How do variadic templates work in C++, and what are their uses?
Answer: Variadic templates allow functions or classes to accept any number of template parameters, making it ideal for designing flexible functions like those that can take any number of arguments.
Using C++ in Embedded Systems
C++ is extensively used in embedded systems due to its efficiency and close-to-hardware capabilities.
Challenges in Embedded Programming
Question 63: What are common challenges faced while using C++ in embedded systems?
Answer: Challenges include managing memory and resource constraints, achieving real-time performance, and dealing with hardware interfacing and volatile environments.
Optimizing C++ for Low Power Devices
Question 64: How can C++ be optimized for low-power devices in embedded systems?
Answer: Optimization techniques include minimizing CPU cycles, optimizing memory usage, and using compiler options that enhance performance and reduce power consumption.
Real-time Applications of C++
C++ is crucial for developing applications that require high performance and real-time computation.
Real-time Computing Techniques
Question 65: What techniques are used in C++ for real-time computing?
Answer: Techniques include prioritizing tasks, using real-time operating systems, and applying real-time scheduling algorithms.
High-Performance C++ Applications
Question 66: How is C++ used to enhance the performance of applications?
Answer: C++ enhances performance through features like low-level memory manipulation, optimized STL algorithms, and minimal overhead for object-oriented features.
Optimization and Efficiency in C++ Coding
Optimization is key in C++, where efficiency can significantly impact the performance of applications, from gaming to high-frequency trading systems.
Memory Access and Cache Optimization
Question 67: How can developers optimize memory access patterns in C++ to enhance cache utilization?
Answer: Effective cache utilization can be achieved by optimizing data structures and access patterns to be cache-friendly, such as using contiguous memory allocations like arrays or std::vector which promote cache locality.
Optimizing Compiler Options
Question 68: What compiler options are crucial for optimizing C++ code for production?
Answer: Compiler options like -O2 or -O3 for GCC and Clang increase the optimization level, while options like -march=native optimize the code for the specific architecture of the machine on which the code is compiled.
Robust Error Handling and Exception Management
Robust applications require effective error handling strategies, particularly in C++ where manual resource management is common.
Custom Exception Classes
Question 69: How can creating custom exception classes improve error handling in C++ applications?
Answer: Custom exception classes allow for more precise handling of errors by categorizing different types of errors and responding to each with specific actions, enhancing the application's stability and maintainability.
noexcept Keyword
Question 70: What is the noexcept keyword, and when should it be used?
Answer: The noexcept keyword specifies that a function does not throw exceptions. Using noexcept improves performance by allowing certain optimizations and informs users about the safety of using the function in contexts where exceptions are not allowed.
Advanced Use of C++ Libraries
C++ libraries extend the language’s core capabilities, offering tools for graphics, networking, and more.
Using Boost Libraries
Question 71: What are the advantages of using the Boost libraries in C++?
Answer: Boost libraries provide solutions for areas not covered by the Standard Library, including smart pointers with more features, regular expressions, and graph algorithms, enhancing code robustness and offering more tools to developers.
Integration with Other Languages
Question 72: How can C++ be integrated with other programming languages, and what are the benefits?
Answer: C++ can be integrated with languages like Python through tools like Cython or SWIG, allowing developers to leverage Python's simplicity and C++'s performance, providing a powerful combination for tasks that require both ease of coding and speed.
C++ in Scientific Computing
C++ is widely used in scientific computing where precision and performance are critical.
High-Performance Scientific Computing
Question 73: What makes C++ suitable for high-performance scientific computing?
Answer: C++ provides precise control over system resources and memory, essential for the demanding computations and large data handling required in scientific applications.
Parallel Computing with C++
Question 74: How does C++ support parallel computing in scientific applications?
Answer: C++ supports parallel computing through its Standard Library (like parallel execution policies in algorithms) and external libraries like MPI or OpenMP, which help in distributing the computation across multiple processors.
Maintaining Large-Scale C++ Projects
Maintaining large-scale software projects can be challenging. C++ offers several features to manage this complexity effectively.
Code Modularity and Refactoring
Question 75: How does modularity in C++ enhance maintainability in large projects?
Answer: Modularity in C++—achieved through classes, namespaces, and header files—helps in organizing code into manageable, reusable, and replaceable components, which simplifies maintenance and development.
Effective Use of Version Control
Question 76: What are some best practices for using version control in large C++ projects?
Answer: Best practices include regular commits with clear, descriptive messages, using branches for new features, and frequent merges to avoid divergence, which ensures smooth workflow and easier integration of changes.
Best Practices for Code Reviews in C++
Code reviews are crucial in any development process, especially in C++, where complex code structures and memory management issues can lead to significant bugs.
Conducting Effective Code Reviews
Question 77: What strategies can be employed to conduct effective code reviews in C++?
Answer: Effective strategies include focusing on algorithmic efficiency, memory usage, and adherence to coding standards. Use tools like static analyzers to catch common mistakes before manual review and ensure all code reviewers are familiar with C++ best practices.
Learning from Code Reviews
Question 78: How can developers learn from code reviews to improve their C++ programming skills?
Answer: Developers can learn by actively engaging in discussions during reviews, asking for clarification on suggestions, and applying feedback consistently to subsequent tasks. This iterative process helps in refining one's approach to C++ coding and understanding deeper nuances.
Security Best Practices in C++
Security is paramount in C++ due to its low-level capabilities and direct memory access, which can create vulnerabilities if not handled correctly.
Secure Memory Management
Question 79: What are some best practices for secure memory management in C++?
Answer: Best practices include using smart pointers to manage memory automatically, avoiding raw pointer arithmetic, and rigorously checking all inputs to prevent buffer overflows and other vulnerabilities.
Writing Secure C++ Code
Question 80: What techniques can be used to ensure that C++ code is secure from common vulnerabilities?
Answer: Techniques include validating all user inputs, employing modern C++ features like smart pointers and STL containers over traditional C arrays, and using secure functions like snprintf instead of sprintf.
Advanced GUI Programming in C++
Graphical User Interfaces (GUI) in C++ are developed using various libraries, providing rich user experiences in applications.
Using Qt for GUI Development
Question 81: How does Qt facilitate advanced GUI programming in C++?
Answer: Qt provides a comprehensive set of classes and tools for creating cross-platform GUI applications in C++. It supports everything from basic widgets to complex animations, with strong support for multimedia and networking functionalities.
Best Practices for Responsive UI
Question 82: What are the best practices for developing a responsive UI in C++?
Answer: Best practices include using asynchronous programming to avoid blocking UI operations, designing UIs that adapt to different screen sizes and resolutions, and optimizing rendering paths to reduce redraw times.
Real-Time Systems Programming with C++
Real-time systems require guaranteed response times, and C++ is often chosen for its performance and control over hardware.
Techniques in Real-Time Programming
Question 83: What specific techniques are employed in C++ for real-time systems programming?
Answer: Techniques include prioritizing and managing threads carefully, using real-time operating systems (RTOS) that support C++, and avoiding operations that can cause delays, such as dynamic memory allocation during execution.
Challenges in Real-Time Systems
Question 84: What are common challenges faced when programming real-time systems in C++?
Answer: Challenges include dealing with the unpredictability of execution time due to hardware interrupts, ensuring thread safety without significant locking overhead, and managing the trade-offs between low latency and high throughput.
Utilizing C++ in Cloud Computing
Cloud computing has become ubiquitous, and C++ plays a role in developing performance-sensitive cloud applications.
Developing for the Cloud with C++
Question 85: How is C++ used in cloud computing environments?
Answer: C++ is used in cloud computing for developing core services that require efficient resource management and high performance. It is particularly useful for services where computation speed and low latency are critical.
Scalability and C++
Question 86: What factors should be considered when scaling C++ applications in the cloud?
Answer: Key factors include ensuring the application is stateless where possible, using load balancing effectively, and optimizing data handling and communication to minimize latency and maximize throughput.
Integration of C++ with Mobile Development
As mobile platforms continue to advance, integrating C++ for performance-critical tasks becomes essential, particularly in applications like games or media processing.
Mobile Development with C++
Question 87: How is C++ used in mobile app development?
Answer: C++ is often used for developing the core engine in performance-intensive mobile apps, such as games or applications that require complex data processing, because it provides better control over system resources and performance optimization.
Cross-Platform Mobile Development
Question 88: What are some tools and frameworks that facilitate cross-platform mobile development using C++?
Answer: Tools like Qt Mobile, Unreal Engine, and Cocos2d-x allow developers to write C++ code for applications that run on multiple mobile platforms, reducing development time and maintaining performance benefits.
C++ in Finance and Trading Systems
The finance sector heavily utilizes C++ for its performance critical systems, such as high-frequency trading platforms and complex quantitative finance models.
High-Frequency Trading
Question 89: How does C++ enable high-frequency trading (HFT) systems?
Answer: C++ provides the necessary speed and low-latency processing required for HFT systems by allowing fine control over hardware interaction and memory usage, critical for the millisecond-level decision-making processes in trading.
Risk Management Systems
Question 90: What role does C++ play in financial risk management systems?
Answer: C++ is used to develop systems that require rapid processing of large volumes of complex financial data to calculate potential risks, thanks to its efficiency and the ability to handle concurrent processes smoothly.
C++ for Network Programming
Network programming is another area where C++ excels, thanks to its robust handling of concurrency and system-level operations.
Building Network Simulators
Question 91: What are the advantages of using C++ in building network simulators?
Answer: C++ allows for detailed simulation of network protocols and algorithms, thanks to its performance efficiency and the extensive support provided by libraries like Boost.Asio, which offers tools for network and low-level I/O programming.
Implementing Protocol Stacks
Question 92: How is C++ used to implement custom protocol stacks?
Answer: C++'s ability to interact closely with hardware and manage memory precisely makes it ideal for implementing custom protocol stacks where control over packet processing and timing is crucial.
C++ in Artificial Intelligence and Machine Learning
AI and machine learning can benefit from C++ in scenarios where the performance of algorithms and data processing speed are critical.
Machine Learning Libraries for C++
Question 93: What are some C++ libraries used in machine learning?
Answer: Libraries such as dlib, mlpack, and Shark provide C++ developers with powerful tools for implementing machine learning algorithms efficiently and with high performance.
Developing AI Engines
Question 94: How is C++ utilized in developing AI engines?
Answer: C++ is often chosen for AI engines where the speed of execution is crucial, such as in real-time decision-making systems or games where AI responses need to be immediate and resource-efficient.
Advanced Computational Methods Using C++
C++ is pivotal in fields requiring advanced computational methods, such as scientific computing, engineering simulations, and more.
Computational Fluid Dynamics
Question 95: How does C++ facilitate computational fluid dynamics simulations?
Answer: C++ is used in computational fluid dynamics for its ability to handle complex mathematical computations and perform parallel processing, significantly speeding up simulations.
Structural Analysis
Question 96: What role does C++ play in structural analysis software?
Answer: C++ enables the development of software that can perform intense calculations on structures under various conditions, leveraging its speed and memory management capabilities to provide accurate and timely analyses.
Advanced Graphics Programming in C++
C++ is often the language of choice for graphics programming, particularly for game development and real-time simulation.
Real-time Graphics Rendering
Question 97: How is C++ used in real-time graphics rendering?
Answer: C++ is widely used in real-time graphics for its performance capabilities, crucial in rendering complex scenes with minimal latency. It interfaces efficiently with graphics APIs like OpenGL and DirectX to deliver detailed and dynamic visual content.
Game Engine Development
Question 98: What are the advantages of using C++ in game engine development?
Answer: C++ allows game developers to manage memory explicitly and optimize resource allocation, crucial for maintaining high frame rates and responsiveness in complex game engines.
C++ in Space and Aviation Systems
The space and aviation industries require extremely reliable and precise software to manage operations safely.
Software for Satellite Control
Question 99: How does C++ support software development for satellite control?
Answer: C++ provides the robustness, real-time performance, and low-level hardware control necessary for developing satellite control systems, which must operate reliably in the harsh environment of space.
Avionics Software
Question 100: Why is C++ preferred for avionics software development?
Answer: C++ is preferred in avionics for its performance and deterministic behavior, essential for systems where failure can have catastrophic consequences.
C++ in Academic and Research Fields
C++ is not just industrial but also a staple in academic settings, where its performance and flexibility enable extensive research and experimentation.
Use in Computational Research
Question 101: What makes C++ suitable for computational research in academia?
Answer: C++ offers the precision and control over computational resources necessary for conducting complex numerical simulations and data analysis in various scientific research areas.
Robotics and Automation
Question 102: How is C++ applied in robotics and automation research?
Answer: In robotics and automation, C++ is used to program the low-level systems that require direct control over hardware and real-time processing, essential for developing responsive and autonomous robotic systems.
Emerging Trends in C++ Development
As technology evolves, so does C++'s role in it, adapting to new trends and demands in software development.
C++ in IoT Devices
Question 103: How is C++ used in the development of IoT devices?
Answer: C++ is ideal for IoT devices that require efficient resource management and operational speed, due to its low overhead and system-level control.
Future of C++ in Software Development
Question 104: What is the future of C++ in the ever-evolving landscape of software development?
Answer: C++ continues to evolve with the introduction of new standards like C++20 and C++23, ensuring its relevance by adapting to modern software development needs such as concurrency, cloud computing, and containerization.
C++ in Distributed Systems
Distributed systems leverage C++ for its efficiency and the control it provides over system resources, making it ideal for developing scalable and robust networked applications.
Distributed Database Management
Question 105: How is C++ employed in the development of distributed database management systems?
Answer: C++ is used to develop the core components of distributed databases due to its ability to handle complex operations with high efficiency and low latency, crucial for maintaining consistency and performance across distributed systems.
Development of Microservices
Question 106: Why is C++ suitable for developing microservices in distributed architectures?
Answer: C++ is suitable for microservices that require intensive computation or high I/O throughput due to its performance advantages and the efficient management of resources, crucial for scaling services independently in distributed architectures.
C++ in Cybersecurity Applications
With growing concerns over software security, C++ is critical in developing applications that need to operate securely under threat of external attacks.
Cryptography and Secure Communication
Question 107: How does C++ enhance the implementation of cryptographic algorithms?
Answer: C++ allows for the low-level manipulation of data, enabling the optimization of cryptographic algorithms for speed and security, which is essential for secure communication.
Secure Software Development
Question 108: What are the security practices associated with C++ in software development?
Answer: Key practices include rigorous memory management, the use of modern C++ features to avoid common pitfalls (like raw pointers), and employing static and dynamic analysis tools to detect vulnerabilities early.
C++ in Multimedia Processing
C++ plays a significant role in multimedia applications where performance and real-time processing are crucial.
Audio and Video Processing
Question 109: What makes C++ effective for audio and video processing applications?
Answer: The low-level control over hardware and efficient handling of large streams of data make C++ ideal for developing complex multimedia processing algorithms that require real-time performance.
Graphics and Animation Software
Question 110: How is C++ used in graphics and animation software development?
Answer: C++ is commonly used in graphics and animation due to its ability to handle intensive processing tasks efficiently, crucial for rendering high-quality graphics and smooth animations.
Appendix and Resources
To further enhance your preparation for C++ interview questions, this section provides additional resources and a downloadable PDF featuring the questions and answers discussed in this blog. It's essential to have a well-rounded approach to learning, utilizing a variety of materials to deepen your understanding and broaden your exposure to different types of C++ problems.
Online Courses and Tutorials
- Introductory C++ Programming - Ideal for beginners to get a solid foundation in C++.
- Advanced C++ Programming - Focuses on advanced topics like memory management, multithreading, and template programming.
- C++ for Competitive Programming - Helps improve problem-solving speed and efficiency, crucial for coding interviews.
These courses are available on platforms like Coursera, Udemy, and Pluralsight. They often include both theoretical lessons and practical coding exercises, which are key to mastering C++.
Books
- "C++ Primer" by Stanley B. Lippman - A comprehensive guide to both fundamental and advanced C++ programming concepts.
- "Effective Modern C++" by Scott Meyers - Offers specific guidance on the use of modern C++ language and library features.
- "The C++ Programming Language" by Bjarne Stroustrup - Written by the creator of C++, this book provides deep insights into the language.
Forums and Community Groups
- Stack Overflow: A vast community of developers where you can ask questions and share knowledge about C++ programming.
- Reddit r/cpp: Engage with an active community to discuss the latest in C++ development and interview preparation tips.
Practice Platforms
- LeetCode: Offers a range of C++ problems from basic to advanced levels, ideal for interview prep.
- HackerRank: Focuses on practical challenges and competitions in C++.
These platforms provide an excellent way to practice coding problems and apply what you've learned in real-world scenarios.
Conclusion
With these 110+ C++ interview questions, we've covered a broad spectrum of topics, from foundational concepts to advanced applications in various domains. This comprehensive guide is designed to not only prepare you for rigorous technical interviews but also to give you a deeper understanding of how C++ fits into the modern software development landscape. As you prepare for your interviews, remember that mastery of C++ comes from both theoretical knowledge and practical experience. Continue coding, exploring new features, and challenging yourself with complex problems to ensure you remain at the cutting edge of software development with C++.
Read More:
- Different types of Interview Questions
- 10 Common Job Interview Questions And What Answers To Be Expected
- The Hiring Journey: 100+ Good Interview Questions to Ask In An Interview in 2024
- Here Are The Top Interview Questions You Need To Add To Your Interview Now!
- Informational Interview Questions
- 11+ Unique Interview Questions To Ask Employer During The Interview
- A Complete Guide on the Best Interview Questions to Ask Candidates
- Market Executive Interview Questions and Answers
- 30+ Second Interview Questions To Ask Candidates
- Employee Satisfaction Sample Questions
- How to Answer Interview Questions? [By Mastering the Employer's Perspective]
- Video Interview Questions: The Different Categories and Types
- Common Exit Interview Questions
- Customer Success Trainer Interview Questions
- Data Analyst Interview Questions: Learn to hire the perfect data analyst
- 50+ Content Marketing Expert Interview Questions and Answers
- Enterprise Customer Success Manager Interview Questions and Answers
- Online Advertising Expert Interview Questions & Answers
- Questions to Ask When Hiring SEM Experts
- Top 110+ Selenium Interview Questions and Answers
Authors
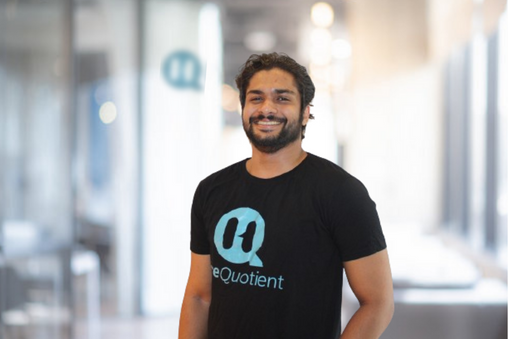
Thomas M. A.
A literature-lover by design and qualification, Thomas loves exploring different aspects of software and writing about the same.
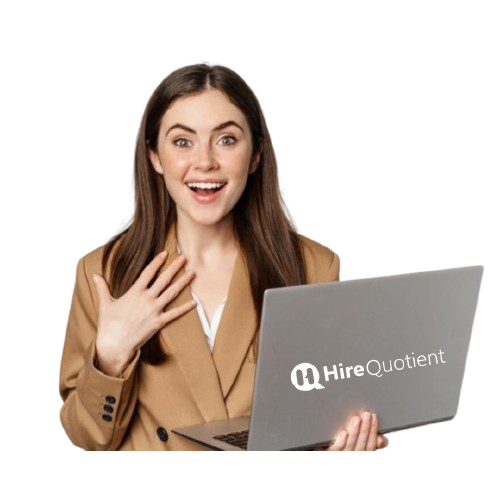
Hire the best without stress
Ask us how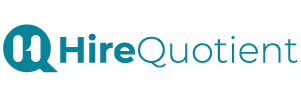
Never Miss The Updates
We cover all recruitment, talent analytics, L&D, DEI, pre-employment, candidate screening, and hiring tools. Join our force & subscribe now!
Stay On Top Of Everything In HR