50 Essential C# Interview Questions
Published on May 1st, 2024
C# (pronounced "C sharp") is a powerful and versatile programming language developed by Microsoft. It is widely used for building web applications, desktop applications, games, and more. As demand for skilled C# developers continues to rise, employers must conduct thorough interviews to identify the best candidates for their teams. This guide provides a comprehensive list of C# interview questions categorized by experience level and scenario, helping employers assess candidates' knowledge, skills, and problem-solving abilities effectively.
Basic C# Interview Questions for Freshers
1. What is C#?
Answer: C# is a high-level, object-oriented programming language developed by Microsoft as part of the .NET framework. It is designed to build robust and scalable applications for the Microsoft ecosystem.
2. What are the key features of C#?
Answer: Key features of C# include type safety, garbage collection, automatic memory management, scalability, and support for object-oriented programming concepts like inheritance, polymorphism, and encapsulation.
3. Explain the difference between value types and reference types in C#.
Answer: Value types store data directly in memory, while reference types store a reference to the memory location where the data is stored. Value types include simple types like int, float, and bool, while reference types include classes, interfaces, arrays, and strings.
4. What is a namespace in C#?
Answer: A namespace is a way to organize and group related classes, interfaces, structs, enums, and delegates in C#. It helps prevent naming conflicts and makes it easier to manage and maintain code.
5. What is the difference between an abstract class and an interface?
Answer: An abstract class can contain both abstract (unimplemented) and concrete (implemented) members, while an interface can only contain method signatures, properties, events, and indexers (without implementation). A class can inherit from multiple interfaces but only one abstract class.
6. What is the purpose of the Main method in a C# application?
Answer: The Main method is the entry point of a C# application. It is a static method that serves as the starting point for program execution and is called automatically by the runtime when the program is started.
7. What are constructors in C#?
Answer: Constructors are special methods in a class used to initialize the object's state. They have the same name as the class and are called automatically when an object of the class is created.
8. Explain the difference between the stack and the heap in C#.
Answer: The stack is used to store value types and method calls, while the heap is used to store reference types and objects. The runtime automatically manages stack memory and is faster but limited in size, while heap memory requires explicit memory management and is larger but slower.
9. What is inheritance in C#?
Answer: Inheritance is a mechanism in C# that allows a class (derived class) to inherit properties and behavior from another class (base class). It promotes code reuse and supports the "is-a" relationship between classes.
10. What is encapsulation in C#?
Answer: Encapsulation is the process of bundling data (attributes) and methods (behavior) that operate on the data into a single unit, called a class. It hides the internal implementation details of a class and exposes only the necessary functionality through methods and properties.
11. What is polymorphism in C#?
Answer: Polymorphism is the ability of a method to behave differently based on the object it is called on. It allows a single method to have multiple implementations, depending on the type of object it operates on.
12. What is the difference between a struct and a class in C#?
Answer: A struct is a value type, while a class is a reference type. Structs are typically used for lightweight objects that have value semantics, while classes are used for more complex objects that require reference semantics.
13. What are access modifiers in C#?
Answer: Access modifiers control the visibility and accessibility of classes, methods, properties, and other members in C#. The common access modifiers are public, private, protected, internal, and protected internal.
14. What is method overloading in C#?
Answer: Method overloading is a feature in C# that allows a class to have multiple methods with the same name but different parameter lists. It enables developers to create methods that perform similar tasks but with different input parameters.
15. What is the difference between an instance variable and a static variable in C#?
Answer: An instance variable (also known as a member variable) is associated with an instance of a class and has a separate copy for each object, while a static variable (also known as a class variable) is shared among all instances of a class and has only one copy per class.
16. What is the purpose of using directives in C#?
Answer: The directive is used to import namespaces into a C# file, allowing you to reference types defined in those namespaces without fully qualifying their names.
17. What is method overriding in C#?
Answer: Method overriding is a feature in C# that allows a derived class to provide a specific implementation of a method that is already defined in its base class. It enables polymorphic behavior, where a method call on a base class reference can invoke the overridden method in the derived class.
18. What is a delegate in C#?
Answer: A delegate in C# is a type that represents a reference to a method. It provides a way to encapsulate and pass around methods as objects, enabling callback mechanisms, event handling, and functional programming concepts like lambda expressions and LINQ.
19. What is an event in C#?
Answer: An event in C# is a mechanism for communication between objects, where one object (the publisher) notifies other objects (subscribers) when a particular action or state change occurs. Events are typically implemented using delegates and are widely used in GUI programming and asynchronous programming.
20. What is the difference between == and Equals() method in C#?
Answer: The == operator in C# compares the reference equality of objects for reference types and value equality for value types. The Equals() method, on the other hand, is used to compare the contents or values of objects and can be overridden to provide custom comparison logic.
Intermediate C# Interview Questions
21. Explain the concept of boxing and unboxing in C#.
Answer: Boxing is the process of converting a value type to a reference type, while unboxing is the process of converting a reference type back to a value type. Boxing occurs implicitly when a value type is assigned to an object variable, and unboxing requires an explicit cast from the object to the original value type.
22. What are generics in C#?
Answer: Generics in C# allow you to define classes, interfaces, methods, and delegates with placeholder types that are specified at compile-time. They provide type safety and code reusability by enabling the creation of parameterized types and algorithms.
23. What is a lambda expression in C#?
Answer: A lambda expression in C# is an anonymous function that can be used to create delegates or expression trees. It provides a concise syntax for writing inline functions and is commonly used in LINQ queries, event handling, and functional programming.
24. What is the purpose of the var keyword in C#?
Answer: The var keyword in C# is used to declare implicitly typed local variables. It allows the compiler to infer the variable type based on the initialization expression, reducing verbosity and improving readability.
25. What is the difference between IEnumerable and IQueryable in C#?
Answer: IEnumerable is an interface that represents a sequence of elements that can be enumerated using for each or LINQ queries in memory. IQueryable, on the other hand, is an interface that represents a queryable data source that can be composed and executed against a database using LINQ to SQL or LINQ to Entities.
26. What is the async/await pattern in C#?
Answer: The async/await pattern in C# is a language feature introduced in C# 5.0 that simplifies asynchronous programming. It allows you to write asynchronous code that looks like synchronous code, making it easier to understand and maintain.
27. How does garbage collection work in C#?
Answer: Garbage collection in C# is a process by which the runtime automatically manages memory by reclaiming objects that are no longer in use. It works by periodically scanning the managed heap for objects that are not reachable and freeing up their memory.
28. What are extension methods in C#?
Answer: Extension methods in C# allow you to add new methods to existing types without modifying the original type or creating a new derived type. They provide a way to extend the functionality of classes, interfaces, and structures, enabling better code organization and reusability.
29. What is the purpose of the using statement in C#?
Answer: The statement in C# is used to ensure that IDisposable objects are properly disposed of when they are no longer needed. It automatically calls the Dispose() method on IDisposable objects when they go out of scope, helping to release unmanaged resources and prevent memory leaks.
30. What is dependency injection in C#?
Answer: Dependency injection in C# is a design pattern that allows you to inject dependencies into a class from outside rather than creating them internally. It promotes loose coupling, testability, and flexibility by decoupling the creation and management of dependencies from the consuming classes.
Advanced C# Interview Questions for Experienced
31. What are attributes in C#?
Answer: Attributes in C# are declarative tags that provide metadata about types, members, or assemblies. They can be used to add information, instructions, or behavior to code elements at compile-time or runtime, enabling reflection and customizing the behavior of the code.
32. What are delegates and events in C#? How are they related?
Answer: Delegates in C# are type-safe function pointers that can reference methods with a specific signature. Events are a special type of delegate that encapsulates the publish-subscribe pattern, allowing objects to subscribe to and receive notifications from other objects.
33. What is reflection in C#?
Answer: Reflection in C# is a mechanism that allows you to inspect and manipulate metadata, types, and members of assemblies at runtime. It provides a way to dynamically load types, invoke methods, access properties, and create instances, enabling powerful runtime introspection and customization.
34. What are asynchronous streams in C#?
Answer: Asynchronous streams in C# are a language feature introduced in C# 8.0 that allows you to consume asynchronous data sequences asynchronously. They combine the features of async/await and IEnumerable/IAsyncEnumerable to provide efficient and convenient handling of asynchronous streams of data.
35. What are tuples in C#?
Answer: Tuples in C# are lightweight data structures that allow you to store a fixed-size collection of heterogeneous elements. They provide a convenient way to return multiple values from a method or pass multiple values as arguments to a method.
36. What are expression-bodied members in C#?
Answer: Expression-bodied members in C# are a syntactic feature that allows you to define methods, properties, indexers, and operators using concise lambda-like syntax. They provide a more compact and readable way to define simple members, reducing boilerplate code.
37. What are pattern matching and switch expressions in C#?
Answer: Pattern matching in C# is a language feature that allows you to test the shape and structure of data against a pattern and extract information from it. Switch expressions extend traditional switch statements with pattern-matching capabilities, enabling more expressive and concise code.
38. What is the purpose of the async modifier in C#?
Answer: The async modifier in C# is used to define asynchronous methods that can perform long-running operations without blocking the calling thread. It enables the use of the await keyword within the method body to asynchronously await the completion of asynchronous operations.
39. What is the IDisposable interface, and how is it used?
Answer: The IDisposable interface in C# is used to define a method, Dispose(), that releases unmanaged resources held by an object. It is commonly used to clean up resources such as file handles, database connections, or network sockets. Implementing IDisposable allows objects to be explicitly cleaned up when they are no longer needed, improving resource management and preventing memory leaks.
40. What is parallel programming in C#?
Answer: Parallel programming in C# is a technique that involves executing multiple tasks concurrently to improve performance and scalability. It leverages features like the Task Parallel Library (TPL), Parallel LINQ (PLINQ), and async/await keywords to distribute work across multiple CPU cores and threads efficiently.
Scenario-Based C# Interview Questions
41. You have a large collection of data that needs to be processed in parallel. How would you design a solution using C#?
Answer: I would use the Task Parallel Library (TPL) to divide the data into smaller chunks and process them concurrently using parallel loops or parallel LINQ queries. I would also consider using async/await keywords for asynchronous processing to avoid blocking the calling thread.
42. Your application needs to interact with a RESTful API. How would you implement HTTP requests and handle responses in C#?
Answer: I would use the HttpClient class in the System.Net.Http namespace to send HTTP requests and receive responses from the API. I would use asynchronous methods like SendAsync() and ReadAsStringAsync() to perform non-blocking I/O operations and handle the responses asynchronously.
43. Your company wants to implement logging and error handling in its C# applications. How would you design a reusable logging framework?
Answer: I would create a custom logging library or use an existing logging framework like Serilog or NLog. I would define interfaces for logging providers and implement different logging providers for logging to various targets such as files, databases, or cloud services. I would also implement error-handling mechanisms using try-catch blocks and log exceptions with relevant contextual information.
44. You are tasked with optimizing the performance of a database query in a C# application. What strategies would you employ?
Answer: I would start by analyzing the query execution plan and identifying any inefficiencies or bottlenecks. I would optimize the query by adding appropriate indexes, rewriting the query to use more efficient joins or filters, or partitioning large tables. I would also consider caching frequently accessed data or implementing data denormalization to reduce the number of database round-trips.
45. Your application needs to process a large number of files asynchronously. How would you design a solution using C#?
Answer: I would use asynchronous file I/O operations provided by the System.IO namespace to read and write files asynchronously. I would use async/await keywords to perform non-blocking file operations and parallelize file processing using tasks or parallel loops. I would also handle exceptions and implement error-handling mechanisms to ensure robustness.
46. Your team is developing a real-time chat application in C#. How would you implement real-time communication between clients and the server?
Answer: I would use technologies like SignalR or WebSockets to establish a persistent connection between clients and the server for real-time communication. I would implement server-side hubs or endpoints to handle incoming messages and broadcast them to connected clients asynchronously. I would also implement client-side logic to send and receive messages in real time and update the UI accordingly.
47. You need to implement data validation and sanitization in a C# web application. What approach would you take?
Answer: I would use data annotation attributes or the FluentValidation library to define validation rules for model properties. I would validate incoming data in the controller actions using ModelState.IsValid property or FluentValidation's validation methods. I would sanitize user input to prevent SQL injection, cross-site scripting (XSS), and other security vulnerabilities using parameterized queries or input validation techniques.
48. Your application needs to perform long-running background tasks without blocking the main thread. How would you implement asynchronous processing in C#?
Answer: I would use asynchronous programming techniques like async/await keywords and the Task Parallel Library (TPL) to execute long-running tasks asynchronously without blocking the main thread. I would use async methods to perform I/O-bound operations and parallelize CPU-bound operations using tasks or parallel loops. I would also handle task cancellation and error handling to ensure robustness and reliability.
49. You are developing a multi-threaded application in C#. How would you synchronize access to shared resources and prevent race conditions?
Answer: I would use synchronization mechanisms like locks, mutexes, semaphores, or monitors to ensure exclusive access to shared resources and prevent race conditions. I would also use thread-safe collections like ConcurrentDictionary or ReaderWriterLockSlim to safely access shared data from multiple threads. I would carefully design the threading model and use synchronization primitives judiciously to avoid deadlocks and performance bottlenecks.
50. Your company wants to implement unit testing and test-driven development (TDD) practices in its C# projects. How would you approach this?
Answer: I would use a unit testing framework like NUnit, MSTest, or xUnit.net to write automated tests for individual units of code (e.g., classes, and methods). I would follow the Arrange-Act-Assert (AAA) pattern to structure test methods and use mocking frameworks like Moq or NSubstitute to isolate dependencies and simulate behavior. I would also adopt test-driven development (TDD) practices by writing failing tests before writing production code and iteratively refactoring code to pass tests. I would strive for high test coverage and incorporate testing into the continuous integration (CI) pipeline to ensure code quality and reliability.
Authors
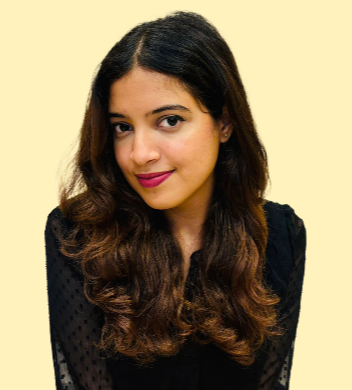
Vinaya Birkodi
Vinaya is a Social Media Strategist and Content Writer with a passion for content that connects. She blends data, trends, and storytelling to craft content that doesn’t just perform it resonates. When she’s not decoding algorithms or building brand love online, you’ll find her exploring creative outlets like photography and short-form video. Always online, always on-brand.
Hire the best without stress
Ask us how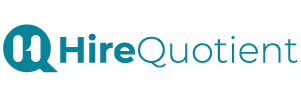
Never Miss The Updates
We cover all recruitment, talent analytics, L&D, DEI, pre-employment, candidate screening, and hiring tools. Join our force & subscribe now!
Stay On Top Of Everything In HR