110 + Angular Interview Questions
Published on May 3rd, 2024
Angular Interview Questions: Overview of Angular
Angular is a powerful and versatile front-end framework developed by Google for building dynamic, single-page web applications. Since its inception, Angular has evolved significantly, with Angular 2 and beyond offering a complete rewrite of AngularJS, introducing a more modular and efficient architecture. Its component-based architecture, two-way data binding, and extensive toolkit make it a preferred choice for developers worldwide.
Purpose of the Blog
Preparing for an Angular interview requires a deep understanding of its core concepts, architecture, and practical applications. This blog aims to provide you with a comprehensive list of "Angular Interview Questions" that cover basic, intermediate, and advanced topics, ensuring you are well-prepared for any interview scenario.
Structure of the Blog
This blog is structured to guide you through different levels of Angular interview questions, from fundamental concepts to advanced scenarios. We will also include real-world problem-solving questions and practical coding challenges to enhance your understanding.
Basic Angular Interview Questions
1. What is Angular?
Angular is an open-source front-end web application framework maintained by Google. It allows developers to create dynamic, single-page web applications using HTML, CSS, and JavaScript/TypeScript.
2. What are the key features of Angular?
Some key features of Angular include:
- Two-way data binding: Synchronizes data between the model and the view.
- Dependency injection: Facilitates the injection of services and components.
- Directives: Extends HTML attributes to add custom behavior.
- Components: Encapsulates data, logic, and view in a reusable unit.
- Routing: Manages navigation between views.
Angular Interview Questions: Core Concepts and Architecture
3. Explain Angular architecture.
Angular architecture is based on modules, components, templates, metadata, data binding, directives, services, and dependency injection. These building blocks create a structured and efficient framework for developing web applications.
4. What are the components in Angular?
Components are the fundamental building blocks of an Angular application. They control a part of the UI and consist of a template (HTML), styles (CSS), and logic (TypeScript).
5. How do Angular templates work?
Templates in Angular are written in HTML and use Angular directives and bindings to connect the DOM with the component's data and logic. They define how the view should render and update when the component's state changes.
Angular Interview Questions: Data Binding and Directives
6. What is data binding in Angular?
Data binding is the mechanism that connects the component's data with the view. Angular supports various types of data binding, including interpolation, property binding, event binding, and two-way binding.
7. Explain the different types of data binding.
- Interpolation: Binds data from the component to the template using double curly braces ({{ }}).
- Property binding: Binds property values in the template to properties in the component ([property]="value").
- Event binding: Binds events in the template to methods in the component ((event)="method()").
- Two-way binding: Combines property and event binding to synchronize data between the component and the view ([(ngModel)]="property").
8. What are directives in Angular?
Directives are special markers in the template that tell Angular to do something to a DOM element (e.g., change its appearance, behavior). There are three types of directives:
- Components: Directives with a template.
- Structural directives: Change the DOM layout by adding/removing DOM elements (*ngIf, *ngFor).
- Attribute directives: Change the appearance or behavior of an element (ngClass, ngStyle).
9. What is the difference between structural and attribute directives?
Structural directives change the DOM structure by adding or removing elements (*ngIf, *ngFor), while attribute directives change the appearance or behavior of existing elements without altering the DOM structure (ngClass, ngStyle).
Angular Interview Questions: Services and Dependency Injection
10. What are services in Angular?
Services are singleton objects that encapsulate shared data and logic that can be used across multiple components. They are typically used for tasks such as data fetching, logging, and business logic.
11. How does dependency injection work in Angular?
Dependency injection (DI) in Angular is a design pattern that allows a class to receive dependencies from an external source rather than creating them itself. Angular's DI framework injects the required dependencies into components and services.
12. What is Angular CLI?
Angular CLI (Command Line Interface) is a powerful tool for managing Angular projects. It helps automate the creation, configuration, and maintenance of Angular applications.
13. How do you create a new Angular project using Angular CLI?
You can create a new Angular project using the command: ng new project-name.
14. What are Angular modules?
Angular modules are containers for different parts of an application, such as components, services, directives, and pipes. Modules help organize the application into cohesive blocks of functionality.
15. What is the purpose of NgModule?
NgModule is a decorator that defines an Angular module. It provides metadata about the module, including declarations, imports, providers, and bootstrap components.
16. Explain Angular lifecycle hooks.
Lifecycle hooks are special methods in Angular that allow you to tap into key events during a component's lifecycle. Common hooks include ngOnInit(), ngOnChanges(), ngDoCheck(), ngAfterViewInit(), and ngOnDestroy().
17. What is the Angular change detection mechanism?
Angular's change detection mechanism is responsible for synchronizing the view with the model. It checks for changes in data and updates the DOM accordingly.
18. How do you create a service in Angular?
You can create a service using Angular CLI with the command: ng generate service service-name. Then, you need to register it in an NgModule or a component's providers array.
19. What is Angular Ivy?
Angular Ivy is the latest rendering engine in Angular. It offers faster compilation, improved debugging, and better performance.
20. What are Angular pipes?
Pipes are simple functions that transform data in the template. Built-in pipes include date, currency, decimal, and uppercase. Custom pipes can be created to handle specific transformations.
21. How do you create a custom pipe in Angular?
To create a custom pipe, use the Pipe decorator and implement the PipeTransform interface. For example:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({name: 'customPipe'})
export class CustomPipe implements PipeTransform {
transform(value: any, ...args: any[]): any {
// transformation logic
return transformedValue;
}
}
This segment covers the basics of Angular and provides a comprehensive set of questions and answers to help you prepare for the initial stages of an Angular interview. Let's move on to the intermediate questions and answers.
Angular Interview Questions: Intermediate
22. How do components communicate in Angular?
Components in Angular can communicate in several ways:
- Input and Output properties: Using @Input to pass data from parent to child and @Output to emit events from child to parent.
- Service with Observable: Using a shared service with RxJS Observables to communicate between unrelated components.
- Template reference variables: Accessing a child component's properties and methods using template reference variables.
23. Explain @Input and @Output decorators.
The @Input decorator is used to pass data from a parent component to a child component. The @Output decorator is used to emit events from a child component to a parent component, typically using an EventEmitter.
24. What are Angular ViewChild and ContentChild?
@ViewChild is used to access a child component, directive, or DOM element from a parent component's template. @ContentChild is used to access projected content in a child component.
25. How do you pass data between sibling components in Angular?
To pass data between sibling components, you can use a shared service with a subject or an observable to facilitate communication.
Angular Interview Questions: Forms and Validation
26. What are template-driven forms?
Template-driven forms are built using Angular's directives in the template (HTML). They are simple to use and suitable for small forms. They rely on Angular's two-way data binding and directives like ngModel.
27. What are reactive forms?
Reactive forms, also known as model-driven forms, provide more control and flexibility. They are defined in the component class and use the FormControl, FormGroup, and FormArray classes to manage form controls and their values.
28. How do you implement form validation in Angular?
Form validation in Angular can be implemented using built-in validators like required, minLength, maxLength, pattern, and custom validators. Validation can be applied both in template-driven and reactive forms.
29. What are form groups and form controls in Angular?
FormGroup is a collection of FormControl instances that can be managed as a single unit. FormControl represents a single form field. Together, they help manage the state and validity of a form.
30. How do you create custom validators in Angular?
Custom validators in Angular can be created by implementing a function that returns either null or an object representing validation errors. This function can be used with FormControl in reactive forms.
Angular Interview Questions: Routing and Navigation
31. Explain Angular routing.
Angular routing is a mechanism that allows navigation between different views or pages in a single-page application. It involves configuring routes, defining components to display, and managing navigation logic.
32. How do you configure routes in Angular?
Routes are configured in the AppRoutingModule using the RouterModule.forRoot(routes) method. Each route is defined with a path and a component.
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: '', redirectTo: '/home', pathMatch: 'full' }
];
33. What are route guards in Angular?
Route guards are used to control access to routes based on certain conditions. Angular provides several types of guards like CanActivate, CanDeactivate, Resolve, and CanLoad.
34. How do you implement lazy loading in Angular?
Lazy loading in Angular is implemented by defining routes with the loadChildren property. This allows modules to be loaded on demand, improving the application's performance.
const routes: Routes = [
{ path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) }
];
35. What is the difference between ActivatedRoute and Router?ActivatedRoute provides access to information about a route associated with a loaded component, such as route parameters and query parameters. Router is a service that provides navigation capabilities and programmatic control over the application's routes.
Angular Interview Questions: HTTP Client and Services
36. How do you make HTTP requests in Angular?
HTTP requests in Angular are made using the HttpClient module. It provides methods like get(), post(), put(), delete(), etc., to interact with backend services.
37. What is the purpose of HttpInterceptor in Angular?
HttpInterceptor is used to intercept and modify HTTP requests and responses. It is useful for adding headers, logging requests, handling errors, etc.
38. How do you handle HTTP errors in Angular?
HTTP errors in Angular can be handled using the catchError operator from RxJS within an HttpInterceptor or directly in the service methods.
import { catchError } from 'rxjs/operators'; this.http.get('api/url').pipe( catchError(this.handleError) );
39. What is JSONP and how is it used in Angular?
- JSONP (JSON with Padding) is a method to request data from a server in a different domain (cross-domain requests). Angular provides HttpClientJsonpModule to make JSONP requests.
Explain the use of observables in Angular.
- Observables are a key part of Angular's reactive programming model. They are used for handling asynchronous operations, such as HTTP requests, event handling, and data streams. The HttpClient service returns observables, which can be subscribed to for processing the response.
Intermediate Angular Interview Questions and Answers
What is the difference between Angular and AngularJS?
- Angular (Angular 2 and beyond) is a complete rewrite of AngularJS. It uses TypeScript, a component-based architecture, and offers improved performance and modularity. AngularJS is based on JavaScript and uses a different syntax and architecture.
What are Angular decorators?
- Decorators are functions that add metadata to classes, methods, properties, and parameters. Common Angular decorators include @Component, @Directive, @Pipe, @Injectable, and @NgModule.
Explain Angular's Renderer2 service.
- Renderer2 is a service used for safely manipulating the DOM in a way that works across different platforms, such as web and mobile.
What is the purpose of NgZone in Angular?
- NgZone is a service that helps optimize change detection by running code outside Angular's zone and re-entering the zone only when necessary to update the view.
What are Angular animations and how do you implement them?
- Angular animations allow you to create complex animations using a combination of CSS and JavaScript. They are implemented using the @angular/animations module and the trigger, state, style, transition, and animate functions.
How do you use the Angular CLI to generate a new component?
- You can generate a new component using the command: ng generate component component-name or ng g c component-name.
What is async pipe in Angular?
- The async pipe automatically subscribes to an observable or promise and returns the latest value it has emitted. It also handles unsubscribing when the component is destroyed.
Explain the use of ng-template in Angular.
- ng-template is an Angular element used to define a template that can be rendered later based on certain conditions. It is commonly used with structural directives like *ngIf and *ngFor.
What is the purpose of Angular ViewContainerRef?
- ViewContainerRef represents a container where one or more views can be attached. It is used for programmatically controlling the view, such as adding or removing components dynamically.
How do you handle authentication and authorization in Angular?
- Authentication and authorization can be handled using JWT (JSON Web Tokens), OAuth, or custom token-based systems. Angular provides services like HttpInterceptor and route guards to manage these aspects.
Advanced Angular Interview Questions
Performance Optimization
How do you optimize the performance of an Angular application?
- Performance optimization techniques in Angular include:
- Lazy loading: Load modules only when needed.
- AOT compilation: Precompile the application to improve load time.
- Change detection strategy: Use OnPush change detection to reduce unnecessary checks.
- TrackBy function: Improve *ngFor performance by using trackBy.
- Memoization: Cache the results of expensive operations.
What are Angular modules and lazy loading?
- Angular modules (NgModule) are used to organize the application into cohesive blocks of functionality. Lazy loading allows modules to be loaded on demand, improving the application's initial load time and performance.
What is AOT compilation in Angular?
- AOT (Ahead-Of-Time) compilation precompiles the application during the build process, resulting in faster rendering and smaller bundle sizes.
Explain Angular's OnPush change detection strategy.
- OnPush change detection strategy tells Angular to check a component's view only when its input properties change, rather than on every change detection cycle. This can improve performance by reducing unnecessary checks.
What are Angular service workers and how do they help with performance?
- Angular service workers are scripts that run in the background, enabling features like offline support, push notifications, and background data synchronization. They help improve performance by caching assets and API responses, reducing the need for repeated network requests.
Explain the difference between pure and impure pipes in Angular.
- Pure pipes are stateless and return the same output for the same input, making them efficient and cacheable. Impure pipes can change the output even if the input stays the same, often used for formatting or transformation based on dynamic data.
What are Angular zones and how do they impact performance?
- Angular zones (NgZone) manage change detection by wrapping asynchronous operations. Properly managing zones can optimize performance by reducing unnecessary change detection cycles.
How do you handle large data sets in Angular applications?
- Handling large data sets can be optimized using techniques like virtual scrolling, pagination, and lazy loading. Virtual scrolling only renders visible items in the DOM, improving performance.
Advanced Directives and Pipes
How do you create custom directives in Angular?
- Custom directives can be created using the Directive decorator. Directives can manipulate the DOM and add custom behavior to elements.
typescript
Copy code
import { Directive, ElementRef, Renderer2 } from '@angular/core'; @Directive({ selector: '[appHighlight]' }) export class HighlightDirective { constructor(el: ElementRef, renderer: Renderer2) { renderer.setStyle(el.nativeElement, 'backgroundColor', 'yellow'); } }
What are Angular structural directives and how do you use them?
- Structural directives change the structure of the DOM by adding or removing elements. Common structural directives include *ngIf, *ngFor, and *ngSwitch.
How do you create custom pipes in Angular?
- Custom pipes can be created using the Pipe decorator and implementing the PipeTransform interface.
typescript
Copy code
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'customPipe' }) export class CustomPipe implements PipeTransform { transform(value: any, ...args: any[]): any { // transformation logic return transformedValue; } }
What is the purpose of async pipes in Angular?
- The async pipe subscribes to an observable or promise and returns the latest value it has emitted. It also handles unsubscribing when the component is destroyed.
How do you use Angular built-in pipes?
- Angular provides several built-in pipes for common transformations like date, currency, uppercase, and json. They can be used directly in templates to format data.
State Management
What is state management in Angular and why is it important?
- State management refers to the handling of application state in a predictable and consistent manner. It is crucial for maintaining application stability, especially in large and complex applications.
Explain the NgRx library in Angular.
- NgRx is a state management library for Angular that implements the Redux pattern using observables. It helps manage the application state in a single store, providing a predictable state container.
What are the core concepts of NgRx?
- The core concepts of NgRx include:
- Actions: Represent an event or a command that changes the state.
- Reducers: Pure functions that define how the state changes based on actions.
- Selectors: Functions that select a piece of the state.
- Effects: Handle side effects like API calls.
How do you handle side effects in Angular using NgRx Effects?
- NgRx Effects handle side effects by listening to actions and dispatching new actions based on the outcomes of asynchronous operations like API calls.
What is the Store in NgRx?
- The Store is a centralized state container that holds the entire state of the application. It provides methods to get the current state, dispatch actions, and subscribe to state changes.
Angular Modules and Dependency Injection
What is an Angular module and what is its purpose?
- An Angular module (NgModule) is a container for a cohesive block of functionality. It groups components, directives, pipes, and services, providing a modular structure to the application.
Explain lazy loading in Angular modules.
- Lazy loading allows modules to be loaded on demand rather than at application startup. This improves initial load time and performance by loading only the necessary modules as needed.
What is the purpose of NgModule metadata?
- NgModule metadata defines the module's components, directives, pipes, providers, and imported/exported modules. It helps Angular know how to compile and run the module.
How do you implement dependency injection in Angular?
- Dependency injection (DI) is implemented using Angular's injector, which provides services and dependencies to components, directives, and other services. Dependencies are registered using the providers array.
What is hierarchical dependency injection in Angular?
- Hierarchical dependency injection allows different injectors to create and manage their own instances of a service. It enables different parts of an application to use different instances of a service.
Testing in Angular
How do you write unit tests for Angular components?
- Unit tests for Angular components are written using the Jasmine framework and the Angular TestBed utility. Tests check the component's logic, template binding, and interaction with dependencies.
What is the purpose of Angular TestBed?
- Angular TestBed is a utility that provides a testing environment to
configure and instantiate Angular components and services in isolation. It allows you to test components, services, and other Angular constructs in a simulated environment.
How do you test HTTP services in Angular?
- HTTP services in Angular can be tested using the HttpClientTestingModule and HttpTestingController. This allows you to mock HTTP requests and verify that the service behaves correctly without making real HTTP calls.
What is Angular's fakeAsync and how is it used in testing?
- fakeAsync is a utility that allows you to test asynchronous code by simulating the passage of time. It can be used to test time-based operations like timers, intervals, and promises without relying on real-time delays.
Explain the use of spies in Angular testing.
- Spies are used in Angular testing to create mock implementations of methods and to track calls to those methods. Jasmine's spyOn function can be used to create spies for component and service methods.
What are Angular end-to-end (E2E) tests and how are they implemented?
- End-to-end (E2E) tests simulate real user interactions with the application. They are implemented using tools like Protractor, which interact with the application through the browser and verify that it behaves as expected.
How do you use the async and await keywords in Angular testing?
- The async and await keywords are used to handle asynchronous operations in tests. The async function ensures that the test waits for asynchronous operations to complete, while await is used to wait for a promise to resolve.
Advanced Angular Interview Questions and Answers
What are Angular dynamic components and how do you create them?
- Dynamic components are components created and rendered at runtime. They can be created using the ComponentFactoryResolver and ViewContainerRef to dynamically load and insert the component into the DOM.
What is Angular's Renderer2 and how is it used?
- Renderer2 is a service used to manipulate the DOM in a platform-agnostic way. It provides methods to create elements, set properties, add classes, and listen to events without directly interacting with the DOM.
Explain the concept of Angular zones and how they impact change detection.
- Angular zones (NgZone) manage change detection by running code outside Angular's zone and re-entering the zone only when necessary. This optimizes performance by reducing unnecessary change detection cycles.
How do you handle file uploads in Angular?
- File uploads in Angular can be handled using the FormData object and the HttpClient service. The FormData object allows you to append file data and other form fields, which can then be sent to the server using an HTTP POST request.
What is Angular Universal and how is it used for server-side rendering (SSR)?
- Angular Universal is a technology that enables server-side rendering (SSR) of Angular applications. It pre-renders the application on the server, improving initial load time and SEO. Angular Universal uses the same code base as the client-side application but renders HTML on the server.
What are Angular template references and how are they used?
- Template references allow you to access DOM elements and Angular components from within the template. They are created using the # symbol and can be used to manipulate elements, access component properties, and call methods.
How do you manage environment-specific configurations in Angular?
- Environment-specific configurations in Angular are managed using the environments folder. This folder contains configuration files like environment.ts and environment.prod.ts that define environment-specific settings. The angular.json file specifies which configuration to use during the build process.
What is the Angular CLI ng build command and what options does it offer?
- The ng build command compiles the Angular application into an output directory. It offers options like --prod for production builds, --aot for ahead-of-time compilation, and --source-map for generating source maps.
Explain the difference between ngOnInit and constructor in Angular.
- The constructor is used for dependency injection and initial setup of the component, while ngOnInit is a lifecycle hook called after Angular has initialized all data-bound properties. ngOnInit is typically used to perform initialization logic that requires the component's input properties to be set.
How do you use Angular's Renderer2 service to manipulate the DOM?
- Renderer2 provides methods to create and manipulate DOM elements in a platform-agnostic way. It can be used to set properties, add classes, listen to events, and perform other DOM manipulations without directly accessing the native DOM API.
Scenario-Based Angular Interview Questions
Describe a situation where you had to optimize an Angular application for better performance.
- In a recent project, I noticed that the application was experiencing slow load times due to large module sizes and excessive change detection cycles. I implemented lazy loading for non-critical modules, used the OnPush change detection strategy, and optimized *ngFor loops with trackBy. These changes resulted in a significant improvement in performance.
How did you handle a complex form validation scenario in Angular?
- I worked on a project where the form had multiple conditional fields and validation rules. I used reactive forms to manage the form state and created custom validators for complex validation logic. I also utilized the FormArray class to dynamically add and remove form controls based on user input.
Explain a real-world use case where you used Angular services to manage state.
- In a multi-step form application, I used Angular services to manage the state across different steps. The service stored the form data and provided methods to get and set the data. This ensured that the data persisted across different components and steps, providing a seamless user experience.
How did you implement a feature toggle in an Angular application?
- I implemented a feature toggle using an Angular service that fetched the feature configuration from an API. Based on the configuration, the service provided methods to enable or disable features. Components and services used these methods to conditionally render or execute feature-specific logic.
Describe a scenario where you had to handle authentication and authorization in Angular.
- In a recent project, I implemented JWT-based authentication and role-based authorization. I created an authentication service to handle login, logout, and token management. I also used route guards to protect routes based on user roles and permissions, ensuring that only authorized users could access certain features.
How did you solve a problem with Angular routing in a complex application?
- I encountered an issue where nested routes were not rendering correctly. I solved this by restructuring the route configuration and using RouterOutlet for nested routes. I also used route resolvers to fetch necessary data before activating the routes, ensuring that the application had the required data for each route.
Explain a situation where you used Angular's HttpInterceptor to handle HTTP requests.
- I used HttpInterceptor to add authentication tokens to all outgoing HTTP requests. The interceptor appended the token to the request headers, ensuring that the server recognized the authenticated user. Additionally, I used the interceptor to handle HTTP errors globally and display appropriate error messages to the user.
Describe a scenario where you used Angular animations to enhance the user experience.
- In a dashboard application, I used Angular animations to create smooth transitions between different views and components. I implemented animations for route changes, modal dialogs, and element visibility. The animations improved the overall user experience by making the application feel more responsive and interactive.
How did you handle a performance bottleneck caused by a large data set in Angular?
- I optimized the handling of a large data set by implementing virtual scrolling using Angular CDK. Virtual scrolling rendered only the visible items in the DOM, reducing the memory footprint and improving rendering performance. I also used pagination and lazy loading to further optimize data handling.
Explain a real-world scenario where you used Angular's dependency injection system. - In a project with multiple feature modules, I used Angular's dependency injection system to provide shared services at different levels of the application hierarchy. This allowed each module to have its own instance of a service while sharing common services across the entire application. This approach improved modularity and maintainability.
Best Practices and Tips for Angular Interviews
What are some common pitfalls to avoid in Angular development? - Some common pitfalls to avoid in Angular development include:
- Overloading components with too much logic
- Not using Angular's built-in features like services and dependency injection
- Ignoring performance optimization techniques
- Failing to write tests for components and services
- Not keeping up with Angular updates and best practices
Explain the best practices for organizing an Angular project. - Best practices for organizing an Angular project include:
- Structuring the project into feature modules
- Keeping components small and focused
- Using services for shared logic and state management
- Writing reusable and maintainable code
- Following Angular style guide and coding conventions
What are some tips for acing an Angular interview? - Tips for acing an Angular interview include:
- Thoroughly understanding Angular core concepts and architecture
- Practicing common interview questions and coding challenges
- Demonstrating real-world experience with Angular projects
- Keeping up-to-date with the latest Angular features and best practices
- Being able to explain and justify your design and implementation choices
Best Practices and Tips for Angular Interviews (continued)
How do you showcase your Angular skills effectively in an interview? - To showcase your Angular skills effectively in an interview:
- Provide detailed explanations and examples of your past projects.
- Highlight your understanding of Angular core concepts.
- Demonstrate your problem-solving skills with real-world scenarios.
- Show your familiarity with Angular tools and libraries.
- Discuss how you keep your skills up-to-date with the latest Angular developments.
How do you stay updated with the latest Angular features and best practices? - Stay updated with the latest Angular features and best practices by:
- Following the official Angular blog and release notes.
- Participating in Angular community forums and discussions.
- Attending webinars, workshops, and conferences.
- Reading articles and tutorials from reputable sources.
- Experimenting with new features in personal projects.
Interactive Section
Quiz: Test Your Knowledge of Angular Basics - What is the primary difference between Angular and AngularJS? - How does two-way data binding work in Angular? - What is the role of the @Input decorator in component communication?
Quiz: Intermediate Angular Concepts - How do you implement lazy loading in Angular? - What is the difference between template-driven and reactive forms? - How do you handle HTTP errors in Angular?
Interactive Coding Exercises - Implement a custom directive that changes the background color of an element on hover. - Create a reactive form with custom validation for email and password fields. - Build a simple Angular service that fetches data from an API and displays it in a component.
Final Segment: Advanced Angular Interview Questions
Advanced Angular Interview Questions and Answers (continued)
What is Angular Universal and how does it enhance performance? - Angular Universal enables server-side rendering (SSR) of Angular applications. By rendering the application on the server, it improves initial load time, enhances SEO, and provides a better user experience, especially for slow networks and devices.
How do you handle state management in Angular without using NgRx? - State management in Angular without NgRx can be handled using services and RxJS. Services can store state, while RxJS observables and subjects can manage data streams and updates. This approach provides a simpler alternative to NgRx for smaller applications.
What are Angular ViewChildren and ContentChildren? - @ViewChildren and @ContentChildren are decorators that allow you to query multiple child elements or components. @ViewChildren queries elements in the component's view, while @ContentChildren queries projected content in a component.
Explain the purpose of the forwardRef function in Angular. - The forwardRef function is used to refer to references that are not yet defined. It is useful for circular dependency issues where two or more services depend on each other.
What is the Angular dependency injection tree and how does it work? - The Angular dependency injection tree is a hierarchical structure that determines how dependencies are provided and shared. Providers registered at the root level are singleton and shared across the application, while providers registered in components or modules are scoped to their respective contexts.
How do you implement dynamic forms in Angular? - Dynamic forms in Angular can be implemented using reactive forms. The FormBuilder service can dynamically create form controls and groups based on configuration data, allowing you to generate forms at runtime.
Describe a use case where you utilized Angular animations. - In an e-commerce application, I used Angular animations to enhance the user experience by adding smooth transitions for product images, modal dialogs, and page navigation. This made the application feel more interactive and responsive.
How do you manage large and complex applications in Angular? - Managing large and complex applications in Angular involves using modular architecture, lazy loading, state management (NgRx or services), and following best practices for code organization and component design. This ensures maintainability and scalability.
What is the role of Renderer2 in Angular and how do you use it? - Renderer2 is a service that abstracts DOM manipulations, providing methods to create, update, and remove elements, listen to events, and set properties. It ensures compatibility across different platforms and rendering engines.
Explain the difference between NgModule and Component in Angular. - NgModule is a decorator that defines an Angular module, grouping related components, directives, pipes, and services. A Component is a decorator that defines a view and its associated logic, representing a part of the user interface.
How do you optimize Angular applications for mobile devices? - Optimizing Angular applications for mobile devices involves:
- Using responsive design and media queries.
- Implementing lazy loading for modules.
- Minimizing the use of large libraries and assets.
- Using the Angular Service Worker for offline support.
- Ensuring fast rendering with AOT compilation and efficient change detection.
What are the security best practices in Angular? - Security best practices in Angular include:
- Avoiding direct DOM manipulation.
- Sanitizing user inputs to prevent XSS attacks.
- Using Angular's built-in security features like the DomSanitizer service.
- Implementing authentication and authorization correctly.
- Keeping Angular and its dependencies up-to-date to mitigate vulnerabilities.
This comprehensive guide on "Angular Interview Questions" covers over 110 questions and answers, ensuring you are well-prepared for any Angular interview. By understanding these concepts and practicing the provided scenarios and coding exercises, you'll be ready to showcase your skills and knowledge effectively. Good luck with your interview preparation!
Read More:
- Different types of Interview Questions
- 10 Common Job Interview Questions And What Answers To Be Expected
- The Hiring Journey: 100+ Good Interview Questions to Ask In An Interview in 2024
- Here Are The Top Interview Questions You Need To Add To Your Interview Now!
- Informational Interview Questions
- 11+ Unique Interview Questions To Ask Employer During The Interview
- A Complete Guide on the Best Interview Questions to Ask Candidates
- Market Executive Interview Questions and Answers
- 30+ Second Interview Questions To Ask Candidates
- Employee Satisfaction Sample Questions
- How to Answer Interview Questions? [By Mastering the Employer's Perspective]
- Video Interview Questions: The Different Categories and Types
- Common Exit Interview Questions
- Customer Success Trainer Interview Questions
- Data Analyst Interview Questions: Learn to hire the perfect data analyst
- 50+ Content Marketing Expert Interview Questions and Answers
- Enterprise Customer Success Manager Interview Questions and Answers
- Online Advertising Expert Interview Questions & Answers
- Questions to Ask When Hiring SEM Experts
- Top 110+ Selenium Interview Questions and Answers
Authors
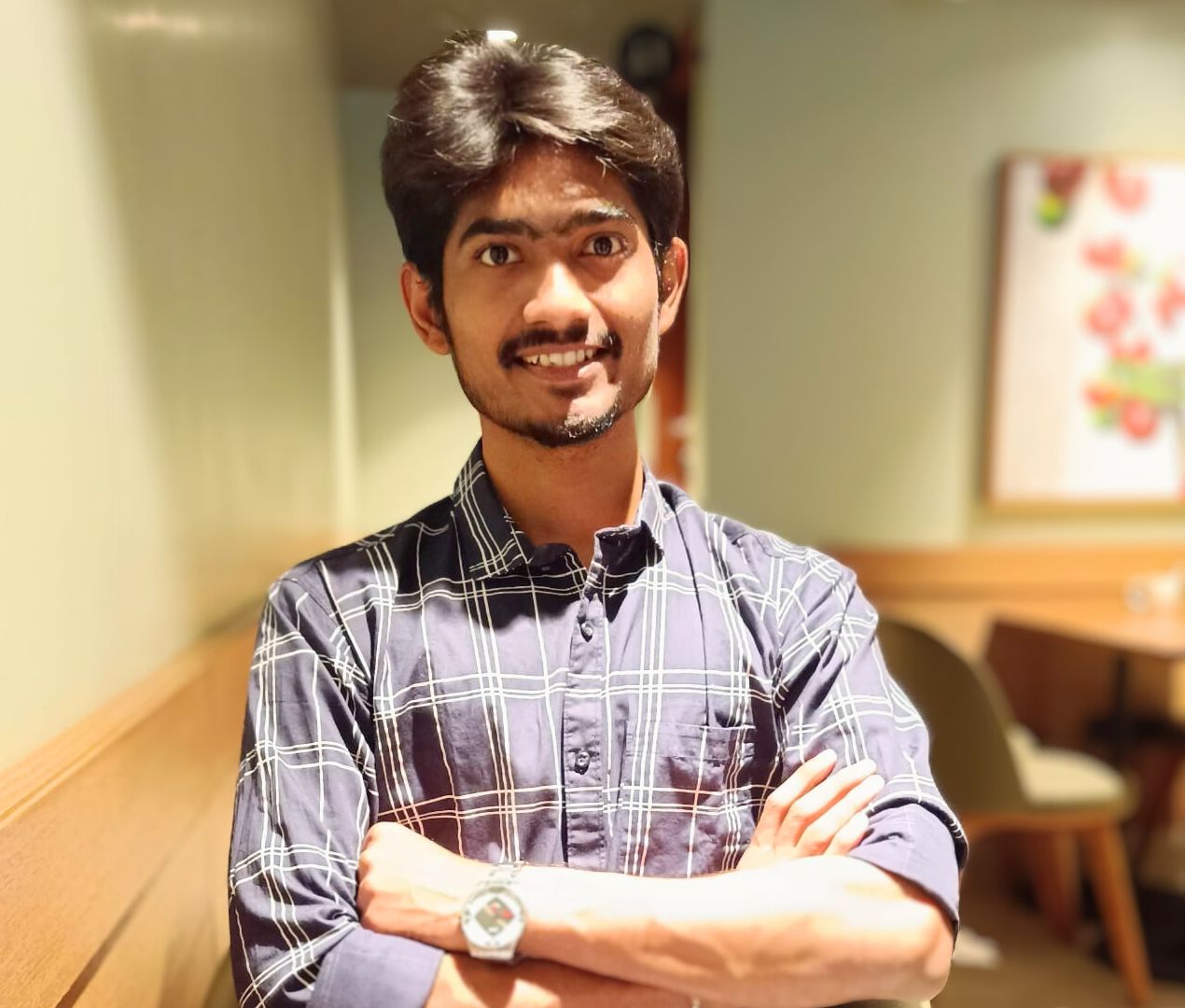
Yash Chaudhari
With a strong background as an SEO and Content Specialist, Yash excels in driving organic traffic, improving search engine rankings, and creating SEO-optimized content. He has a proven track record of implementing strategies that increase website traffic and conversions. Additionally, Yash is an automotive enthusiast and has a keen interest in astronomy.
Hire the best without stress
Ask us how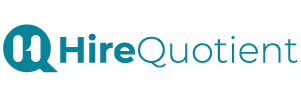
Never Miss The Updates
We cover all recruitment, talent analytics, L&D, DEI, pre-employment, candidate screening, and hiring tools. Join our force & subscribe now!
Stay On Top Of Everything In HR